1. open
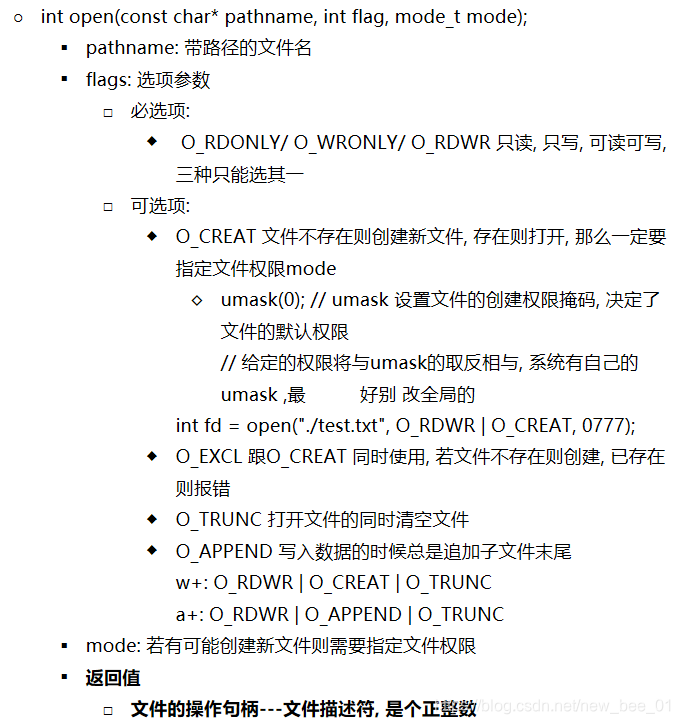
2. write
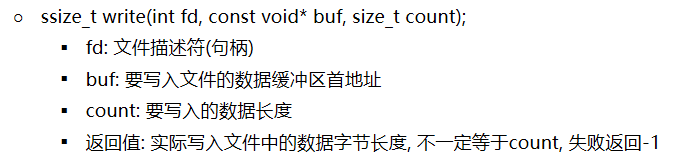
1 #include <stdio.h>
2 #include <unistd.h>
3 #include <sys/types.h>
4 #include <sys/stat.h>
5 #include <fcntl.h>
6 #include <string.h>
7 int main()
8 {
9 int fd;
10 umask(0);
11 const char* filename = "/home/test/a.txt";
12 fd = open(filename, O_RDWR|O_APPEND, 0664);
13 if (fd < 0)
14 {
15 perror("open error!");
16 return -1;
17 }
18 char string[] = "hello IO!\n";
19 const char* buf = "good!\n";
20 write(fd, string, strlen(buf));
21 write(fd, string, strlen(string));
22 close(fd);
23 return 0;
24 }
3. read
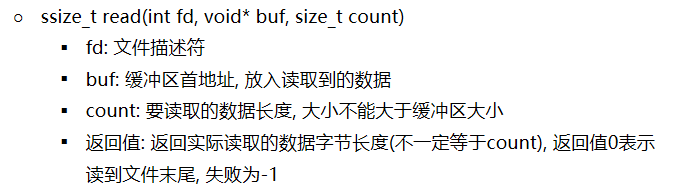
1 #include <stdio.h>
2 #include <sys/types.h>
3 #include <sys/stat.h>
4 #include <fcntl.h>
5 #include <unistd.h>
6 #include <string.h>
7
8 int main()
9 {
10 int fd = open("/home/test/a.txt", O_RDONLY);
11 if (fd < 0)
12 {
13 perror("open error");
14 return -1;
15 }
16 char buf[1024] = "";
17 while(1)
18 {
19 ssize_t s = read(fd, buf, 1);
20 if (s > 0)
21 {
22 printf("%s", buf);
23 }
24 else
25 {
26 break;
27 }
28 }
29 close(fd);
30
31 return 0;
32 }
4. 其他
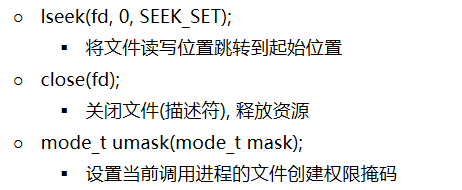