1.题目链接
http://codeforces.com/contest/907/problem/A2.题面
A family consisting of father bear, mother bear and son bear owns three cars. Father bear can climb into the largest car and he likes it. Also, mother bear can climb into the middle car and she likes it. Moreover, son bear can climb into the smallest car and he likes it. It's known that the largest car is strictly larger than the middle car, and the middle car is strictly larger than the smallest car.
Masha came to test these cars. She could climb into all cars, but she liked only the smallest car.
It's known that a character with size a can climb into some car with size b if and only if a ≤ b, he or she likes it if and only if he can climb into this car and 2a ≥ b.
You are given sizes of bears and Masha. Find out some possible integer non-negative sizes of cars.
You are given four integers V1, V2, V3, Vm(1 ≤ Vi ≤ 100) — sizes of father bear, mother bear, son bear and Masha, respectively. It's guaranteed that V1 > V2 > V3.
Output three integers — sizes of father bear's car, mother bear's car and son bear's car, respectively.
If there are multiple possible solutions, print any.
If there is no solution, print "-1" (without quotes).
50 30 10 10
50 30 10
100 50 10 21
-1
In first test case all conditions for cars' sizes are satisfied.
In second test case there is no answer, because Masha should be able to climb into smallest car (so size of smallest car in not less than 21), but son bear should like it, so maximum possible size of it is 20.
3.题意
题意还是比较恶心的,喜欢一个车要求为体型n要n<=v&&2*n>=v.大概就是有三个人体型分别为a,b,c有三辆车体积v满足v1>=a&&v1<=2*a,v2>=b&&v2<=2*b,v3>=c&&v3<=2*c.然后现在给你一个人的体型,让你确定三个车的大小满足这个人能进去三个车但只喜欢c车。
4.思路
读懂题意就可以直接模拟了。
5.代码实现
#include<iostream> #include<math.h> #include<stdio.h> #include<algorithm> #include<queue> #include<map> #include<stack> #include<set> #include<vector> #include <time.h> #include<string.h> using namespace std; typedef long long ll; typedef unsigned long long ull; typedef double db; typedef pair <int, int> pii; typedef pair <ll, ll> pll; typedef pair <ll, int> pli; typedef pair <db, db> pdd; const int maxn = 2005; const int Mod=1000000007; const int INF = 0x3f3f3f3f; const ll LL_INF = 0x3f3f3f3f3f3f3f3f; const double e=2.718281828459045235360287471352662; const db PI = acos(-1); const db ERR = 1e-10; #define Se second #define Fi first #define pb push_back int main() { ios::sync_with_stdio(false); //freopen("a.txt","r",stdin); //freopen("b.txt","w",stdout); int a,b,c,n; cin>>a>>b>>c>>n; if(n>2*c||2*n<c||n>=a||n>=b) { //cout<<"ok"<<endl; cout<<-1<<endl; return 0; } else { for(int i=c;i<=2*c;i++) { //cout<<n<<" "<<i<<endl; if(n<=i&&2*n>=i) { cout<<a*2<<endl; cout<<b*2<<endl; cout<<i<<endl; return 0; } } } cout<<-1<<endl; //cout << "time: " << (long long)clock() * 1000 / CLOCKS_PER_SEC << " ms" << endl; return 0; }
6.反思
对于小范围的不确定的选择条件的时候,可以不需要手算推结论,直接暴力算方案。
1.题目链接
http://codeforces.com/contest/907/problem/B2.题面
Two bears are playing tic-tac-toe via mail. It's boring for them to play usual tic-tac-toe game, so they are a playing modified version of this game. Here are its rules.
The game is played on the following field.
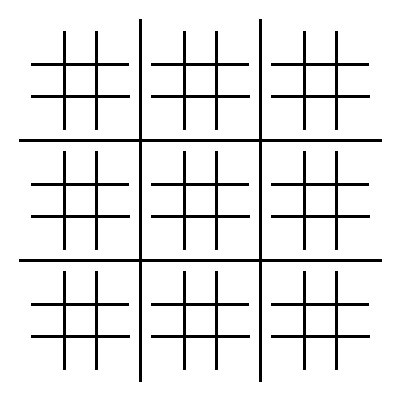
Players are making moves by turns. At first move a player can put his chip in any cell of any small field. For following moves, there are some restrictions: if during last move the opposite player put his chip to cell with coordinates (xl, yl) in some small field, the next move should be done in one of the cells of the small field with coordinates (xl, yl). For example, if in the first move a player puts his chip to lower left cell of central field, then the second player on his next move should put his chip into some cell of lower left field (pay attention to the first test case). If there are no free cells in the required field, the player can put his chip to any empty cell on any field.
You are given current state of the game and coordinates of cell in which the last move was done. You should find all cells in which the current player can put his chip.
A hare works as a postman in the forest, he likes to foul bears. Sometimes he changes the game field a bit, so the current state of the game could be unreachable. However, after his changes the cell where the last move was done is not empty. You don't need to find if the state is unreachable or not, just output possible next moves according to the rules.
First 11 lines contains descriptions of table with 9 rows and 9 columns which are divided into 9 small fields by spaces and empty lines. Each small field is described by 9 characters without spaces and empty lines. character "x" (ASCII-code 120) means that the cell is occupied with chip of the first player, character "o" (ASCII-code 111) denotes a field occupied with chip of the second player, character "." (ASCII-code 46) describes empty cell.
The line after the table contains two integers x and y (1 ≤ x, y ≤ 9). They describe coordinates of the cell in table where the last move was done. Rows in the table are numbered from up to down and columns are numbered from left to right.
It's guaranteed that cell where the last move was done is filled with "x" or "o". Also, it's guaranteed that there is at least one empty cell. It's not guaranteed that current state of game is reachable.
Output the field in same format with characters "!" (ASCII-code 33) on positions where the current player can put his chip. All other cells should not be modified.
... ... ... ... ... ... ... ... ... ... ... ... ... ... ... ... x.. ... ... ... ... ... ... ... ... ... ... 6 4
... ... ... ... ... ... ... ... ... ... ... ... ... ... ... ... x.. ... !!! ... ... !!! ... ... !!! ... ...
xoo x.. x.. ooo ... ... ooo ... ... x.. x.. x.. ... ... ... ... ... ... x.. x.. x.. ... ... ... ... ... ... 7 4
xoo x!! x!! ooo !!! !!! ooo !!! !!! x!! x!! x!! !!! !!! !!! !!! !!! !!! x!! x!! x!! !!! !!! !!! !!! !!! !!!
o.. ... ... ... ... ... ... ... ... ... xxx ... ... xox ... ... ooo ... ... ... ... ... ... ... ... ... ... 5 5
o!! !!! !!! !!! !!! !!! !!! !!! !!! !!! xxx !!! !!! xox !!! !!! ooo !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!
In the first test case the first player made a move to lower left cell of central field, so the second player can put a chip only to cells of lower left field.
In the second test case the last move was done to upper left cell of lower central field, however all cells in upper left field are occupied, so the second player can put his chip to any empty cell.
In the third test case the last move was done to central cell of central field, so current player can put his chip to any cell of central field, which is already occupied, so he can move anywhere. Pay attention that this state of the game is unreachable.
3.题意
就是给你一个图中样式的棋盘,如果最后一步下在本九宫格的某个方向,下一步就要下在整体来看那个方向的九宫格,如果那个九宫格已经满了,那么可以随意下。
4.思路
模拟模拟,当初选择懒一点用cin读,导致几十行能解决的写了200行,太惨了。以后不懒了。
5.代码实现
#include<iostream> #include<math.h> #include<stdio.h> #include<algorithm> #include<queue> #include<map> #include<stack> #include<set> #include<vector> #include <time.h> #include<string.h> using namespace std; typedef long long ll; typedef unsigned long long ull; typedef double db; typedef pair <int, int> pii; typedef pair <ll, ll> pll; typedef pair <ll, int> pli; typedef pair <db, db> pdd; const int maxn = 2005; const int Mod=1000000007; const int INF = 0x3f3f3f3f; const ll LL_INF = 0x3f3f3f3f3f3f3f3f; const double e=2.718281828459045235360287471352662; const db PI = acos(-1); const db ERR = 1e-10; #define Se second #define Fi first #define pb push_back int flag; char str[15][15][15]; int xx[10]={0,2,2,2,5,5,5,8,8,8}; int yy[10]={0,2,5,8,2,5,8,2,5,8}; void check(int x) { for(int i=1;i<=3;i++) { for(int j=1;j<=3;j++) { if(str[x][i][j]=='.') { flag=1; str[x][i][j]='!'; } } } } void print() { for(int i=1;i<=3;i++) { for(int j=1;j<=3;j++) { for(int k=1;k<=3;k++) { cout<<str[j][i][k]; } cout<<" "; } cout<<endl; } cout<<endl; for(int i=1;i<=3;i++) { for(int j=4;j<=6;j++) { for(int k=1;k<=3;k++) { cout<<str[j][i][k]; } cout<<" "; } cout<<endl; } cout<<endl; for(int i=1;i<=3;i++) { for(int j=7;j<=9;j++) { for(int k=1;k<=3;k++) { cout<<str[j][i][k]; } cout<<" "; } cout<<endl; } } int main() { int x,y; ios::sync_with_stdio(false); //freopen("a.txt","r",stdin); //freopen("b.txt","w",stdout); for(int i=1;i<=3;i++) { for(int j=1;j<=3;j++) { for(int k=1;k<=3;k++) { cin>>str[j][i][k]; } } } for(int i=1;i<=3;i++) { for(int j=4;j<=6;j++) { for(int k=1;k<=3;k++) { cin>>str[j][i][k]; } } } for(int i=1;i<=3;i++) { for(int j=7;j<=9;j++) { for(int k=1;k<=3;k++) { cin>>str[j][i][k]; } } } //print(); cin>>x>>y; int tmp=((x-1)/3)*3+(y/3+(y%3!=0)); int nx=xx[tmp]; int ny=yy[tmp]; //cout<<nx<<" "<<ny<<endl; if(x<nx&&y<ny) { check(1); } else if(x<nx&&y==ny) { check(2); } else if(x<nx&&y>ny) { check(3); } else if(x==nx&&y<ny) { check(4); } else if(x==nx&&y==ny) { check(5); } else if(x==nx&&y>ny) { check(6); } else if(x>nx&&y<ny) { check(7); } else if(x>nx&&y==ny) { check(8); } else if(x>nx&&y>ny) { check(9); } if(flag==0) { for(int i=1;i<=3;i++) { for(int j=1;j<=3;j++) { for(int k=1;k<=3;k++) { if(str[j][i][k]=='.') cout<<"!"; else cout<<str[j][i][k]; } cout<<" "; } cout<<endl; } cout<<endl; for(int i=1;i<=3;i++) { for(int j=4;j<=6;j++) { for(int k=1;k<=3;k++) { if(str[j][i][k]=='.') cout<<"!"; else cout<<str[j][i][k]; } cout<<" "; } cout<<endl; } cout<<endl; for(int i=1;i<=3;i++) { for(int j=7;j<=9;j++) { for(int k=1;k<=3;k++) { if(str[j][i][k]=='.') cout<<"!"; else cout<<str[j][i][k]; } cout<<" "; } cout<<endl; } } else { print(); } //cout << "time: " << (long long)clock() * 1000 / CLOCKS_PER_SEC << " ms" << endl; return 0; }
6.反思
注意存图方式,一般按照读入存图就可以,不要自己想太多。
1.题目链接
http://codeforces.com/contest/907/problem/C2.题面
Valentin participates in a show called "Shockers". The rules are quite easy: jury selects one letter which Valentin doesn't know. He should make a small speech, but every time he pronounces a word that contains the selected letter, he receives an electric shock. He can make guesses which letter is selected, but for each incorrect guess he receives an electric shock too. The show ends when Valentin guesses the selected letter correctly.
Valentin can't keep in mind everything, so he could guess the selected letter much later than it can be uniquely determined and get excessive electric shocks. Excessive electric shocks are those which Valentin got after the moment the selected letter can be uniquely determined. You should find out the number of excessive electric shocks.
The first line contains a single integer n (1 ≤ n ≤ 105) — the number of actions Valentin did.
The next n lines contain descriptions of his actions, each line contains description of one action. Each action can be of one of three types:
- Valentin pronounced some word and didn't get an electric shock. This action is described by the string ". w" (without quotes), in which "." is a dot (ASCII-code 46), and w is the word that Valentin said.
- Valentin pronounced some word and got an electric shock. This action is described by the string "! w" (without quotes), in which "!" is an exclamation mark (ASCII-code 33), and w is the word that Valentin said.
- Valentin made a guess about the selected letter. This action is described by the string "? s" (without quotes), in which "?" is a question mark (ASCII-code 63), and s is the guess — a lowercase English letter.
All words consist only of lowercase English letters. The total length of all words does not exceed 105.
It is guaranteed that last action is a guess about the selected letter. Also, it is guaranteed that Valentin didn't make correct guesses about the selected letter before the last action. Moreover, it's guaranteed that if Valentin got an electric shock after pronouncing some word, then it contains the selected letter; and also if Valentin didn't get an electric shock after pronouncing some word, then it does not contain the selected letter.
Output a single integer — the number of electric shocks that Valentin could have avoided if he had told the selected letter just after it became uniquely determined.
5 ! abc . ad . b ! cd ? c
1
8 ! hello ! codeforces ? c . o ? d ? h . l ? e
2
7 ! ababahalamaha ? a ? b ? a ? b ? a ? h
0
In the first test case after the first action it becomes clear that the selected letter is one of the following: a, b, c. After the second action we can note that the selected letter is not a. Valentin tells word "b" and doesn't get a shock. After that it is clear that the selected letter is c, but Valentin pronounces the word cd and gets an excessive electric shock.
In the second test case after the first two electric shocks we understand that the selected letter is e or o. Valentin tries some words consisting of these letters and after the second word it's clear that the selected letter is e, but Valentin makes 3 more actions before he makes a correct hypothesis.
In the third example the selected letter can be uniquely determined only when Valentin guesses it, so he didn't get excessive electric shocks.
3.题意
就是给你n个语句,每个语句有三种状态,如果是!,则表明该语句中有目标字符,如果为. 则表明该语句中没有目标字符。如果为? 则表明猜测是否为目标字符。每次可以猜对或者可避免的!都让ans++.
4.思路
开两个数组,一个存安全字符,另一个存待定字符,按照输入语句模拟就好了。注意细节,
5.代码实现
#include<iostream> #include<math.h> #include<stdio.h> #include<algorithm> #include<queue> #include<map> #include<stack> #include<set> #include<vector> #include <time.h> #include<string.h> using namespace std; typedef long long ll; typedef unsigned long long ull; typedef double db; typedef pair <int, int> pii; typedef pair <ll, ll> pll; typedef pair <ll, int> pli; typedef pair <db, db> pdd; const int maxn =1e5+5; const int Mod=1000000007; const int INF = 0x3f3f3f3f; const ll LL_INF = 0x3f3f3f3f3f3f3f3f; const double e=2.718281828459045235360287471352662; const db PI = acos(-1); const db ERR = 1e-10; #define Se second #define Fi first #define pb push_back int n; char op[10]; char s[maxn]; int num[maxn],t[maxn]; int main() { int ans = 0; int flag =0; cin>>n; while(n--) { cin>>op>>s; if(op[0] == '.') { for(int i=0;s[i];i++)//降本字符串中所有字符放进安全数组 num[s[i]]=1; } if(op[0] == '!') { memset(t,0,sizeof(t)); for(int i=0; s[i]; i++)//将本字符串中的都放进待定数组 t[s[i]] = 1; for(int i='a'; i<='z'; i++)//将本字符串中没有的字符放进安全数组 if( !t[i] ) num[i] = 1; if(flag) ans++; } if(op[0] == '?') { if(flag && num[s[0]]) //安全数组中有25个字符,说明已经能确定答案。 ans++; else num[s[0]] = 1; } int cnt = 0; for(int i='a'; i<='z'; i++) if(!num[i]) cnt++; if(cnt == 1) flag = 1; } cout<<ans<<endl; return 0; }
6.反思
如果代码越改越长,越来if越多,删除重写会更好。注意能用数组解决的不要用set,操作不方便,容易RE。