该文主要记录自己学习shell所踩的一些坑!!!
什么是shell
windows下有.bat后缀的批处理,在linux下也有类似于批处理的东西,那就是shell,一般后缀为.sh,使用bash运行这种文件。
严格说,shell是一种脚本语言,和python, js一样,与c++,java不同。关于脚本语言和编译语言的区别可以参考知乎:编程语言 标记语言 脚本语言分别有哪些? 区别是什么?
为什么要学shell
在linux下,使用shell可以方便的与系统交互,实现很多任务的自动化处理。如果还想要更多理由,可以参考豆瓣:我们为什么要学习Bash Shell
第一个shell文件
这里写下我自己的第一个shell文件,后面简单介绍这个文件所涵盖的知识点,只希望能够点出shell中的若干有趣的点和容易踩到的坑。
#!/bin/bash
str="hello world"
name="lijiguo"
str1="helloworld"
# how to use echo
echo "the" first line : $str "\n"
echo 'the second line :'${str} ${name} '\n'
echo -e "the third line:" ${str1} "\n next line"
#how to use parameter
echo $0
a=10
b=20
#how to use expression
value=`expr $a + $b`
echo $value
value=`expr $a \* $b`
echo $value
#how to use if statement
if [ $a == $b ]
then
echo "a==b"
else
echo "a != b"
fi
#how to use while statement
idx=1
while (($idx<=5 ))
do
echo $idx
idx=`expr ${idx} + 1`
done
#how to redirect
echo "this is a example" >> test.log
#how to compare str
#how to use python
/bin/python test.py
#how to use case
while :
do
echo "input 1-5, input others to end"
read num
case $num in
1|2|3|4|5) echo "you enter $num"
;;
*) echo "end"
break;
;;
esac
done
程序输出如下:
the first line : \n
the second line : lijiguo \n
the third line: helloworld
next line
./blog.sh
30
200
a != b
1
2
3
4
5
python print test
input 1-5, input others to end
1
you enter 1
input 1-5, input others to end
2
you enter 2
input 1-5, input others to end
6
end
下面逐项介绍shell中涉及到的知识点。
文件第一行
#!/bin/bash
这一行指定执行该文件的shell,该行是约定俗成的。
变量赋值
变量赋值这么简单的事还要说?
这里确实要说,等号两边不能加空格!不能加空格!不能加空格!而且变量赋值变量前面不加美元符。
使用echo
echo "the" first line : $str "\n"
echo 'the second line :'${str} ${name} '\n'
echo -e "the third line:" ${str1} "\n next line"
参考输出:
the first line : \n
the second line : lijiguo \n
the third line: helloworld
next line
- 单引号和双引号,甚至不加引号都可以输出字符串。
- 变量使用时是 $name
- echo不会自动转义输出,加上-e参数才会转义输出。
如何使用参数
假如我们想向shell中传入参数,在shell中如何获取呢?
$0表示shell的文件名,$1表示第一个参数,$2表示第二个参数,以此类推,$*表示将所有参数合成一个字符串返回,$@表示将多有参数分成多个字符串返回。
如何使用表达式
shell不支持直接求解表达式,需要借助expr。
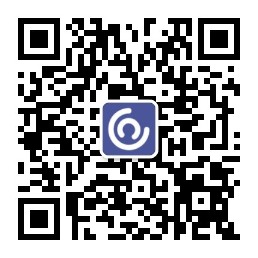
value=`expr $a + $b`
echo $value
value=`expr $a \* $b`
echo $value
输出是
30
200
敲黑板敲黑板:
1. 求解的表达式用反引号引起来,是反引号,ESC下面那个
2. 变量和运算符之间要有空格,$a+$b是不对的,$a + $b才对。
3. 乘法要用\*来实现.
4. 其它运算,都可以这样实现。
使用if语句
- 基本框架是 if condition then else fi
- condition中使用[]或者(())将条件表达式括起来。
- fi是if倒序,表示if语句结束。
如何使用while语句
- 基本框架 while condition do done
- 当condition是冒号(:)时,表示无限循环。
- do/done表示一个语句块,两者成对出现。
如何使用重定向
echo "this is a example" >> test.log
>>两边要有空格
如何使用case
while :
do
echo "input 1-5, input others to end"
read num
case $num in
1|2|3|4|5) echo "you enter $num"
;;
*) echo "end"
break;
;;
esac
done
- ;;表示一个分支结束
- *表示others
- esac表示case的结束,是case的倒序
结语
新手,上面没有全面的语法,没有完整的例程,只是自己踩的一些坑。如有错误,还请不吝赐教。