文章目录
一、配置
(0)POM
配置
pom.xml
下配置
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
(1)客户端
主流有:
JedisConnectionFactory
: 使用Jedis
作为Redis
客户端LettuceConnectionFactory
:使用Lettuce
作为Redis
客户端
Lettuce
是基于Netty
的开源连接器
@Configuration
public class RedisConfig {
@Bean
public RedisTemplate<Object, Object> redisCacheTemplate(LettuceConnectionFactory redisConnectionFactory) {
RedisTemplate<Object, Object> template = new RedisTemplate<>();
template.setKeySerializer(new StringRedisSerializer());
template.setValueSerializer(new GenericJackson2JsonRedisSerializer());
template.setConnectionFactory(redisConnectionFactory);
template.afterPropertiesSet();
return template;
}
}
(2)操作
Spring Data Redis
提供了 RedisTemplate
和 StringRedisTemplate
两个模板来进行数据操作。
@Repository
public class RedisDao {
@Autowired
StringRedisTemplate stringRedisTemplate;
@Autowired
RedisTemplate<Object, Object> redisTemplate;
public void setString(String k, String v) {
stringRedisTemplate.opsForValue().set(k, v);
}
}
(3)序列化
RedisTemplate
默认使用 JdkSerializationRedisSerializer
StringRedisTemplate
默认使用 StringRedisSerializer
Spring Data JPA
提供了如下Serializer
:
GenericToStringSerializer
Jackson2JsonRedisSerializer
JacksonJsonRedisSerializer
JdkSerializationRedisSerializer
OxmSerializer
StringRedisSerializer
对应上文RedisConfig.java
中:
template.setKeySerializer(new StringRedisSerializer());
template.setValueSerializer(new GenericJackson2JsonRedisSerializer());
(4)属性配置
在 application.properties
spring.redis.host=localhost
spring.redis.password=
# 连接超时时间(毫秒)
spring.redis.timeout=10000
# Redis默认情况下有16个分片,这里配置具体使用的分片,默认是0
spring.redis.database=0
# 连接池最大连接数(使用负值表示没有限制) 默认 8
spring.redis.lettuce.pool.max-active=8
# 连接池最大阻塞等待时间(使用负值表示没有限制) 默认 -1
spring.redis.lettuce.pool.max-wait=-1
# 连接池中的最大空闲连接 默认 8
spring.redis.lettuce.pool.max-idle=8
# 连接池中的最小空闲连接 默认 0
spring.redis.lettuce.pool.min-idle=0
二、问题
(1)SpringBoot 2.x 默认依赖是 Lettuce
,为什么不是Jedis
?
官方解释:https://github.com/spring-projects/spring-session/issues/789
性能测试:https://translate.google.com/translate?hl=&sl=ko&tl=en&u=https%3A%2F%2Fjojoldu.tistory.com%2F418
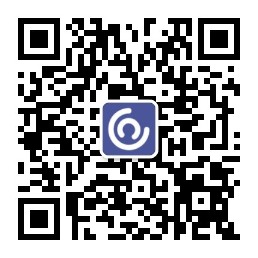
Lettuce
:
Lettuce is built on netty and connection instances (StatefulRedisConnection) can be shared across multiple threads. So a multi-threaded application can use a single connection regardless the number of concurrent threads that interact with Lettuce.
按凡凡理解:就是底层支持的是netty
,有经验啊。
Jedis
:
Each concurrent thread using Jedis gets its own Jedis instance for the duration of Jedis interaction. Connection pooling comes at the cost of a physical connection per Jedis instance which increases the number of Redis connections.
按凡凡理解:每一个业务线程都会获得Jedis
的一个实例来连接,相对浪费实例。
三、文档
https://docs.spring.io/spring-data/data-redis/docs/current/reference/html/#redis.repositories