1. 环形链表
public class DetectCycle142 {
//定义一个单链表
public class ListNode {
int val; //当前节点值
ListNode next; //下一个节点值
//构造方法 初始化当前节点值
ListNode(int x) {
val = x;
next=null;
}
}
/**环形链表
*方法1:哈希表 时间复杂度 O(n)
* @param head
* @return
*/
public ListNode detectCycle01(ListNode head) {
Set<ListNode> visited = new HashSet<ListNode>();
ListNode node = head;
while (node != null) {
if (visited.contains(node)) {
return node;
}
visited.add(node);
node = node.next;
}
return null;
}
/**环形链表
*方法2:双指针 时间复杂度 O(n)
* @param head
* @return
*/
public ListNode detectCycle(ListNode head) {
//定义双指针 快慢两指针
ListNode fast = head, slow = head;
while (true) {
//没有环
if (fast == null || fast.next == null) return null;
//当快慢指针相遇
fast = fast.next.next;
slow = slow.next;
if (fast == slow) break;
}
//找到环节点的头结点
fast = head;
while (slow != fast) {
slow = slow.next;
fast = fast.next;
}
return fast;
}
}
图解:
https://leetcode-cn.com/problems/linked-list-cycle-ii/solution/linked-list-cycle-ii-kuai-man-zhi-zhen-shuang-zhi-/
2. LeetCode代码测试
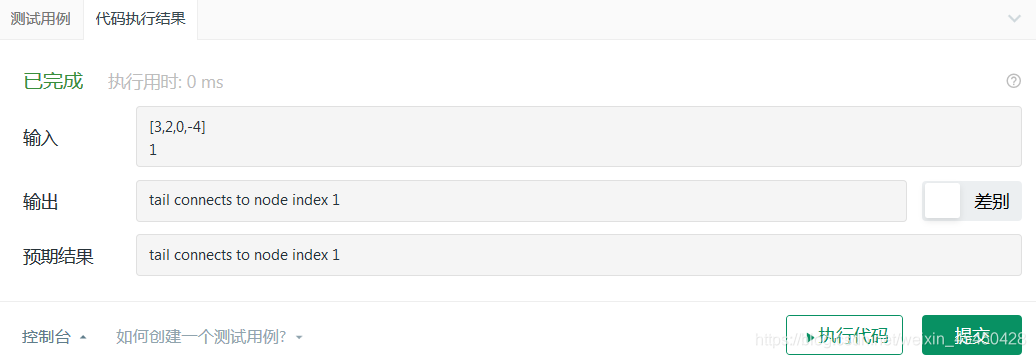