输入整型数组和排序标识,对其元素按照升序或降序进行排序
(一组测试用例可能会有多组数据)
接口说明
原型:
void sortIntegerArray(Integer[] pIntegerArray, int iSortFlag);
输入参数:
Integer[] pIntegerArray:整型数组
int iSortFlag:排序标识:0表示按升序,1表示按降序
输出参数:
无
返回值:
void
示例
输入
// 输入需要输入的整型数个数
8
1 2 4 9 3 55 64 25
0
输出
// 输出排好序的数字
1 2 3 4 9 25 55 64
输入字符串中的字符排序
编写一个程序,将输入字符串中的字符按如下规则排序。
规则 1 :英文字母从 A 到 Z 排列,不区分大小写。
如,输入: Type 输出: epTy
规则 2 :同一个英文字母的大小写同时存在时,按照输入顺序排列。
如,输入: BabA 输出: aABb
扫描二维码关注公众号,回复:
8454081 查看本文章
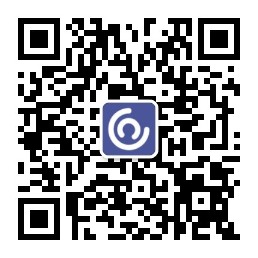
规则 3 :非英文字母的其它字符保持原来的位置。
如,输入: By?e 输出: Be?y
示例1
输入
// 输入字符串
A Famous Saying: Much Ado About Nothing (2012/8).
输出
// 输出字符串
A aaAAbc dFgghh: iimM nNn oooos Sttuuuy (2012/8).
C++
#include <iostream>
#include <string>
using namespace std;
int main(){
string input;
while(getline(cin,input))
{
//getline(cin,input);
string temp;
for(int i=0;i<input.length();i++){
if(isalpha(input[i]))
temp += input[i];
}
for(int i=0;i<temp.length();i++){
for(int j=0;j<temp length="" -1-i="" j="" if="" toupper="" temp="" j=""> toupper(temp[j+1])){
char c = temp[j];
temp[j] = temp[j+1];
temp[j+1] = c;
}
}
}
int k = 0;
for(int i=0;i<input.length();i++){
if(isalpha(input[i]))
input[i] = temp[k++];
}
cout<<input<<endl;
}
return 0;
}
Java
import java.io.IOException;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class Main{
public static void main(String []args) throws IOException{
BufferedReader bf=new BufferedReader(new InputStreamReader(System.in));
String str;
while((str=bf.readLine())!=null){
StringBuffer builder = new StringBuffer();
for(int i=0;i<26;i++){
char c=(char)(i+'A');
for(int j=0;j<str.length();j++){
char sc=str.charAt(j);
if(c==sc||c==sc-32){
builder.append(sc);
}
}
}
for(int i=0;i<str.length();i++){
char c=str.charAt(i);
if(!(c>='a'&&c<='z')&&!(c>='A'&&c<='Z')){
builder.insert(i,c);
}
}
System.out.println(builder.toString());
}
bf.close();
}
}
最短排序
对于一个无序数组A,请设计一个算法,求出需要排序的最短子数组的长度。
给定一个整数数组A及它的大小n,请返回最短子数组的长度。
测试样例:
[1,5,3,4,2,6,7],7
返回:4
C++
class ShortSubsequence {
public:
int findShortest(vector<int> A, int n) {
// write code here
int k = -1;
int max = A[0];
for(int i=1;i<n;i++){
if(max > A[i])
k = i;
else
max = A[i];
}
if(k==-1)return 0;
int m = -1;
int min = A[n-1];
for(int i=n-2;i>=0;i--){
if(min < A[i])
m = i;
else
min = A[i];
}
return k-m+1;
}
};
Java
/*
思路:
1.先判断依次最小值是否在正确位置,直到找到不在正确位置最小值的应该在的位置,作为最小需排序的起始点
2.依次判断最大值是否在正确位置,直到找不到正确位置最大值应该在的位置,作为最小需排序的末尾点
3.计算首末位点间的整数个数,即为需要排序的最短子数组长度
*/
import java.util.*;
public class ShortSubsequence {
public int findShortest(int[] A, int n) {
int maxIndex = n-1;
int i=0;
for(;i<n;i++){
//判断当前子串最小值是否位置正确,若正确继续判断剩余子串最小值是否正确;
//若不正确,判断子串最大值位置是否正确
if(maxIndex==n-1){//
int tmp = findMin(A,i,n);
if(tmp==i){
continue;
}
}
int tmp = findMax(A,i,maxIndex+1);
if(maxIndex==tmp){//若子串最大值位置正确,更新子串最大值位置
maxIndex--;
i--;
continue;
}else{//若子串最大值位置不正确,结束循环
break;
}
}
return maxIndex-i+1;
} //寻找子串中的最小值的下标 public int findMin(int[] sub,int start, int n){
int min = sub[start];
int index = start;
for(int i=start+1;i<n;i++){
if(min>sub[i]){
min=sub[i];
index = i;
}
}
return index;
}
//寻找子串中的最大值的下标
public int findMax(int[] sub,int start, int n){
int max = sub[start];
int index = start;
for(int i=start+1;i<n;i++){
if(max<sub[i]){
max=sub[i];
index = i;
}
}
return index;
}
}