(三)键盘事件
1、键盘弹颜色KeyColor。运行结果如下,当按下1/2/3个键时,按钮的颜色分别为蓝黄绿。试用普通当前类和匿名内部类两种方式分别实例化监听器对象。最后,请把它扩展到7个键,颜色分别为:赤橙黄绿青蓝紫。(40分)
代码1:
package MyAdvancedEvent;
import java.awt.Color;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import javax.swing.*;
public class MyKeyEvent_Example {
JFrame f;
JPanel p;
JButton b1,b2,b3;
public MyKeyEvent_Example() {
f=new JFrame();
p=new JPanel();
b1=new JButton("1");
b2=new JButton("2");
b3=new JButton("3");
b1.addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
if(e.getKeyChar()=='1') {
b1.setBackground(Color.BLUE);
}else if(e.getKeyChar()=='2') {
b2.setBackground(Color.YELLOW);
}else {
b3.setBackground(Color.GREEN);
}
}
public void keyReleased(KeyEvent e) {
b1.setBackground(null);
b2.setBackground(null);
b3.setBackground(null);
}
});
p.add(b1);
p.add(b2);
p.add(b3);
f.add(p);
f.setSize(300,200);
f.setLocationRelativeTo(null);
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new MyKeyEvent_Example();
}
}
代码2:
package MyAdvancedEvent;
import java.awt.Color;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import javax.swing.*;
public class MyKeyEvent_Example1 implements KeyListener{
JFrame f;
JPanel p;
JButton b1,b2,b3;
public MyKeyEvent_Example1() {
f=new JFrame();
p=new JPanel();
b1=new JButton("1");
b1.addKeyListener(this);
b2=new JButton("2");
b2.addKeyListener(this);
b3=new JButton("3");
b3.addKeyListener(this);
p.add(b1);
p.add(b2);
p.add(b3);
f.add(p);
f.setSize(300,200);
f.setLocationRelativeTo(null);
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new MyKeyEvent_Example1();
}
public void keyTyped(KeyEvent e) {
}
public void keyPressed(KeyEvent e) {
char str=e.getKeyChar();
if(str=='1') {
b1.setBackground(Color.BLUE);
}else if(str=='2') {
b2.setBackground(Color.YELLOW);
}else {
b3.setBackground(Color.GREEN);
}
}
public void keyReleased(KeyEvent e) {
b1.setBackground(null);
b2.setBackground(null);
b3.setBackground(null);
}
}
代码3:
package MyAdvancedEvent;
import java.awt.Color;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import javax.swing.*;
public class MyKeyEvent_Example2 {
JFrame f;
JPanel p;
JButton b1,b2,b3,b4,b5,b6,b7;
public MyKeyEvent_Example2() {
f=new JFrame();
p=new JPanel();
b1=new JButton("1");
b2=new JButton("2");
b3=new JButton("3");
b4=new JButton("4");
b5=new JButton("5");
b6=new JButton("6");
b7=new JButton("7");
b1.addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
if(e.getKeyChar()=='1') {
b1.setBackground(Color.RED);
}else if(e.getKeyChar()=='2') {
b2.setBackground(Color.ORANGE);
}else if(e.getKeyChar()=='3') {
b3.setBackground(Color.YELLOW);
}else if(e.getKeyChar()=='4') {
b4.setBackground(Color.GREEN);
}else if(e.getKeyChar()=='5') {
b5.setBackground(Color.CYAN);
}else if(e.getKeyChar()=='6') {
b6.setBackground(Color.BLUE);
}else {
b7.setBackground(new Color(138,43,226));
}
}
public void keyReleased(KeyEvent e) {
b1.setBackground(null);
b2.setBackground(null);
b3.setBackground(null);
b4.setBackground(null);
b5.setBackground(null);
b6.setBackground(null);
b7.setBackground(null);
}
});
p.add(b1);
p.add(b2);
p.add(b3);
p.add(b4);
p.add(b5);
p.add(b6);
p.add(b7);
f.add(p);
f.setSize(300,200);
f.setLocationRelativeTo(null);
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new MyKeyEvent_Example2();
}
}
代码4:
package MyAdvancedEvent;
import java.awt.Color;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import javax.swing.*;
public class MyKeyEvent_Example4 implements KeyListener{
JFrame f;
JPanel p;
JButton b1,b2,b3,b4,b5,b6,b7;
public MyKeyEvent_Example4() {
f=new JFrame();
p=new JPanel();
b1=new JButton("1");
b2=new JButton("2");
b3=new JButton("3");
b4=new JButton("4");
b5=new JButton("5");
b6=new JButton("6");
b7=new JButton("7");
b1.addKeyListener(this);
b2.addKeyListener(this);
b3.addKeyListener(this);
b4.addKeyListener(this);
b5.addKeyListener(this);
b6.addKeyListener(this);
b7.addKeyListener(this);
p.add(b1);
p.add(b2);
p.add(b3);
p.add(b4);
p.add(b5);
p.add(b6);
p.add(b7);
f.add(p);
f.setSize(300,200);
f.setLocationRelativeTo(null);
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new MyKeyEvent_Example4();
}
public void keyTyped(KeyEvent e) {
}
public void keyPressed(KeyEvent e) {
if(e.getKeyChar()=='1') {
b1.setBackground(Color.RED);
}else if(e.getKeyChar()=='2') {
b2.setBackground(Color.ORANGE);
}else if(e.getKeyChar()=='3') {
b3.setBackground(Color.YELLOW);
}else if(e.getKeyChar()=='4') {
b4.setBackground(Color.GREEN);
}else if(e.getKeyChar()=='5') {
b5.setBackground(Color.CYAN);
}else if(e.getKeyChar()=='6') {
b6.setBackground(Color.BLUE);
}else {
b7.setBackground(new Color(138,43,226));
}
}
public void keyReleased(KeyEvent e) {
b1.setBackground(null);
b2.setBackground(null);
b3.setBackground(null);
b4.setBackground(null);
b5.setBackground(null);
b6.setBackground(null);
b7.setBackground(null);
}
}
运行截图:
(四)窗体事件
2、P463【例25.3】捕获和处理窗体焦点变化事件示例WindowFocusListener_Example(书上有完整代码!)(10分)
代码:
package MyAdvancedEvent;
import java.awt.event.WindowEvent;
import java.awt.event.WindowFocusListener;
import javax.swing.*;
public class WindowFocusListener_Example extends JFrame{
public static void main(String[] atrgs) {
WindowFocusListener_Example frame=new WindowFocusListener_Example();
frame.setVisible(true);
}
public WindowFocusListener_Example() {
super();
addWindowFocusListener(new myWindowFocusListener());
setTitle("捕获窗体焦点事件");
setBounds(100,100,500,375);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class myWindowFocusListener implements WindowFocusListener{
public void windowGainedFocus(WindowEvent e) {
System.out.println("窗口获得焦点");
}
public void windowLostFocus(WindowEvent e) {
System.out.println("窗口失去焦点");
}
}
}
截图:
3、P464【例25.4】捕获窗体状态变化事件典型示例WindowStateListener_Example(书上有完整代码!)(10分)
代码:
package MyAdvancedEvent;
import java.awt.Frame;
import java.awt.event.WindowEvent;
import java.awt.event.WindowStateListener;
import javax.swing.*;
public class WindowStateListener_Example extends JFrame{
public static void main(String[] args) {
WindowStateListener_Example frame=new WindowStateListener_Example();
frame.setVisible(true);
}
public WindowStateListener_Example() {
super();
addWindowStateListener(new MyWindowStateListener());
setTitle("捕获窗体状态事件");
setBounds(100,100,500,375);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class MyWindowStateListener implements WindowStateListener{
public void windowStateChanged(WindowEvent e) {
int oldstate=e.getOldState();
int newstate=e.getNewState();
String from="";
String to="";
switch(oldstate) {
case Frame.NORMAL:
from="正常化";break;
case Frame.MAXIMIZED_BOTH:
from="最大化";break;
default:
from="最小化";break;
}
switch(newstate) {
case Frame.NORMAL:
to="正常化";break;
case Frame.MAXIMIZED_BOTH:
to="最大化";break;
default:
to="最小化";
}
System.out.println(from+"---->"+to);
}
}
}
截图:
4、P465【例25.5】捕获和处理其它窗体事件示例WindowListener_Example(书上有完整代码!)(10分)
代码:
package MyAdvancedEvent;
import java.awt.event.WindowEvent;
import java.awt.event.WindowListener;
import javax.swing.*;
public class WindowListener_Example extends JFrame{
public static void main(String[] args) {
WindowListener_Example frame=new WindowListener_Example();
frame.setVisible(true);
}
public WindowListener_Example() {
super();
addWindowListener(new MyWindowListener());
setTitle("捕获其他窗体事件");
setBounds(100,100,500,375);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class MyWindowListener implements WindowListener{
public void windowOpened(WindowEvent e) {
System.out.println("窗口被打开!");
}
public void windowClosing(WindowEvent e) {
System.out.println("窗口将要被关闭!");
}
public void windowIconified(WindowEvent e) {
System.out.println("窗口被最小化!");
}
public void windowDeiconified(WindowEvent e) {
System.out.println("窗口被非最小化!");
}
public void windowActivated(WindowEvent e) {
System.out.println("窗口被激活!");
}
public void windowDeactivated(WindowEvent e) {
System.out.println("窗口不在处于激活状态!");
}
public void windowClosed(WindowEvent e) {
System.out.println("窗口已经被关闭!");
}
}
}
截图:
(五)选项事件
6、P467【例25.6】捕获和处理选项事件的示例ItemEvent_Example(20分)
代码:
package MyAdvancedEvent;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import javax.swing.*;
public class ItemEvent_Example {
JFrame f;
JPanel p;
JComboBox combobox;
public ItemEvent_Example() {
f=new JFrame("选项事件实例");
p=new JPanel();
combobox=new JComboBox();
for(int i=1;i<6;i++) {
combobox.addItem("选项"+i);
}
combobox.addItemListener(new ItemListener() {
public void itemStateChanged(ItemEvent e) {
int statechange=e.getStateChange();
String item=e.getItem().toString();
if(statechange==ItemEvent.SELECTED) {
System.out.println("此次事件由 选中 选项"+"“"+item+"”"+"触发!");
}else if(statechange==ItemEvent.DESELECTED) {
System.out.println("此次事件由 取消选中 选项"+"“"+item+"”"+"触发!");
}else {
System.out.println("此次事件由其他原因触发!");
}
}
});
p.add(combobox);
f.add(p);
f.setVisible(true);
f.setSize(400,200);
f.setLocationRelativeTo(null);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new ItemEvent_Example();
}
}
截图:
(六)选作题
7、九宫格井字棋游戏JFxunhuanT(10分)
效果如下图:
扫描二维码关注公众号,回复:
8453641 查看本文章
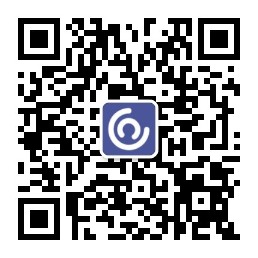
package MyAdvancedEvent;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class MyChessEvent implements ActionListener{
JFrame f1,f2;
JPanel p;
JButton b1,b2;
JButton[]button=new JButton[9];
JLabel l1,l2;
int flag;
int []a=new int[9];
int []b=new int[9];
int count;
public MyChessEvent(){
f1=new JFrame("游戏提示");
f2=new JFrame("九宫格/井字棋");
p=new JPanel(new GridLayout(3,3));
l1=new JLabel("游戏开始,请选定先手。");
l1.setFont(new Font("",Font.BOLD,20));
l2=new JLabel(" 游戏开始");
l2.setFont(new Font("",Font.BOLD,20));
b1=new JButton("红0方");
b1.addActionListener(this);
b2=new JButton("蓝×方");
b2.addActionListener(this);
f1.add(l1,BorderLayout.NORTH);
f1.add(b1,BorderLayout.WEST);
f1.add(b2,BorderLayout.EAST);
f1.setVisible(true);
f1.setSize(250, 120);
f1.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f1.setLocationRelativeTo(null);
for(int i=0;i<9;i++){
button[i]=new JButton();
button[i].addActionListener(this);
p.add(button[i]);
}
f2.add(l2,BorderLayout.NORTH);
f2.add(p,BorderLayout.CENTER);
f2.setVisible(false);
f2.setSize(300, 300);
f2.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f2.setLocationRelativeTo(null);
}
public static void main(String[] args){
new MyChessEvent();
}
public void actionPerformed(ActionEvent e) {
String str=e.getActionCommand();
if(str.equals("红0方")){
f1.setVisible(false);
f2.setVisible(true);
flag=1;
}else if(str.equals("蓝×方")){
f1.setVisible(false);
f2.setVisible(true);
flag=2;
}
if(e.getSource()==button[0]&&flag==1) {
button[0].setText("0");
button[0].setFont(new Font("",Font.BOLD,50));
button[0].setBackground(Color.red);
button[0].setEnabled(false);
count++;
a[0]=1;
flag=2;
}else if(e.getSource()==button[1]&&flag==1) {
button[1].setText("0");
button[1].setFont(new Font("",Font.BOLD,50));
button[1].setBackground(Color.red);
button[1].setEnabled(false);
count++;
a[1]=1;
flag=2;
}else if(e.getSource()==button[2]&&flag==1) {
button[2].setText("0");
button[2].setFont(new Font("",Font.BOLD,50));
button[2].setBackground(Color.red);
button[2].setEnabled(false);
count++;
a[2]=1;
flag=2;
}else if(e.getSource()==button[3]&&flag==1) {
button[3].setText("0");
button[3].setFont(new Font("",Font.BOLD,50));
button[3].setBackground(Color.red);
button[3].setEnabled(false);
count++;
a[3]=1;
flag=2;
}else if(e.getSource()==button[4]&&flag==1) {
button[4].setText("0");
button[4].setFont(new Font("",Font.BOLD,50));
button[4].setBackground(Color.red);
button[4].setEnabled(false);
count++;
a[4]=1;
flag=2;
}else if(e.getSource()==button[5]&&flag==1) {
button[5].setText("0");
button[5].setFont(new Font("",Font.BOLD,50));
button[5].setBackground(Color.red);
button[5].setEnabled(false);
count++;
a[5]=1;
flag=2;
}else if(e.getSource()==button[6]&&flag==1) {
button[6].setText("0");
button[6].setFont(new Font("",Font.BOLD,50));
button[6].setBackground(Color.red);
button[6].setEnabled(false);
count++;
a[6]=1;
flag=2;
}else if(e.getSource()==button[7]&&flag==1) {
button[7].setText("0");
button[7].setFont(new Font("",Font.BOLD,50));
button[7].setBackground(Color.red);
button[7].setEnabled(false);
count++;
a[7]=1;
flag=2;
}else if(e.getSource()==button[8]&&flag==1) {
button[8].setText("0");
button[8].setFont(new Font("",Font.BOLD,50));
button[8].setBackground(Color.red);
button[8].setEnabled(false);
count++;
a[8]=1;
flag=2;
}else {
if(e.getSource()==button[0]&&flag==2) {
button[0].setText("×");
button[0].setFont(new Font("",Font.BOLD,50));
button[0].setBackground(Color.blue);
button[0].setEnabled(false);
count++;
b[0]=1;
flag=1;
}else if(e.getSource()==button[1]&&flag==2) {
button[1].setText("×");
button[1].setFont(new Font("",Font.BOLD,50));
button[1].setBackground(Color.blue);
button[1].setEnabled(false);
count++;
b[1]=1;
flag=1;
}else if(e.getSource()==button[2]&&flag==2) {
button[2].setText("×");
button[2].setFont(new Font("",Font.BOLD,50));
button[2].setBackground(Color.blue);
button[2].setEnabled(false);
count++;
b[2]=1;
flag=1;
}else if(e.getSource()==button[3]&&flag==2) {
button[3].setText("×");
button[3].setFont(new Font("",Font.BOLD,50));
button[3].setBackground(Color.blue);
button[3].setEnabled(false);
count++;
b[3]=1;
flag=1;
}else if(e.getSource()==button[4]&&flag==2) {
button[4].setText("×");
button[4].setFont(new Font("",Font.BOLD,50));
button[4].setBackground(Color.blue);
button[4].setEnabled(false);
count++;
b[4]=1;
flag=1;
}else if(e.getSource()==button[5]&&flag==2) {
button[5].setText("×");
button[5].setFont(new Font("",Font.BOLD,50));
button[5].setBackground(Color.blue);
button[5].setEnabled(false);
count++;
b[5]=1;
flag=1;
}else if(e.getSource()==button[6]&&flag==2) {
button[6].setText("×");
button[6].setFont(new Font("",Font.BOLD,50));
button[6].setBackground(Color.blue);
button[6].setEnabled(false);
count++;
b[6]=1;
flag=1;
}else if(e.getSource()==button[7]&&flag==2) {
button[7].setText("×");
button[7].setFont(new Font("",Font.BOLD,50));
button[7].setBackground(Color.blue);
button[7].setEnabled(false);
count++;
b[7]=1;
flag=1;
}else if(e.getSource()==button[8]&&flag==2) {
button[8].setText("×");
button[8].setFont(new Font("",Font.BOLD,50));
button[8].setBackground(Color.blue);
button[8].setEnabled(false);
count++;
b[8]=1;
flag=1;
}
}
if((a[0]==1&&a[1]==1&&a[2]==1)||(a[0]==1&&a[3]==1&&a[6]==1)||(a[0]==1&&a[4]==1&&a[8]==1)||(a[1]==1&&a[4]==1&&a[7]==1)
||(a[2]==1&&a[5]==1&&a[8]==1)||(a[3]==1&&a[4]==1&&a[5]==1)||(a[2]==1&&a[4]==1&&a[6]==1)||(a[6]==1&&a[7]==1&&a[8]==1))
{
JLabel l=new JLabel();
l.setText("红方获胜,是否再来一局?");
l.setFont(new Font("",Font.BOLD,20));
int i=JOptionPane.showConfirmDialog(f2, l,"游戏结束",JOptionPane.YES_NO_OPTION,JOptionPane.PLAIN_MESSAGE);
if(i==JOptionPane.YES_NO_OPTION) {
f2.setVisible(false);
new MyChessEvent();
}
}
else if((b[0]==1&&b[1]==1&&b[2]==1)||(b[0]==1&&b[3]==1&&b[6]==1)||(b[0]==1&&b[4]==1&&b[8]==1)||(b[1]==1&&b[4]==1&&b[7]==1)
||(b[2]==1&&b[5]==1&&b[8]==1)||(b[3]==1&&b[4]==1&&b[5]==1)||(b[2]==1&&b[4]==1&&b[6]==1)||(b[6]==1&&b[7]==1&&b[8]==1))
{
JLabel l=new JLabel();
l.setText("蓝方获胜,是否再来一局?");
l.setFont(new Font("",Font.BOLD,20));
int i=JOptionPane.showConfirmDialog(f2, l,"游戏结束",JOptionPane.YES_NO_OPTION,JOptionPane.PLAIN_MESSAGE);
if(i==JOptionPane.YES_NO_OPTION) {
f2.setVisible(false);
new MyChessEvent();
}
}
else if(count==9){
JLabel l=new JLabel();
l.setText("平局,是否再来一局?");
l.setFont(new Font("",Font.BOLD,20));
int i=JOptionPane.showConfirmDialog(f2, l,"游戏结束",JOptionPane.YES_NO_OPTION,JOptionPane.PLAIN_MESSAGE);
if(i==JOptionPane.YES_NO_OPTION) {
f2.setVisible(false);
new MyChessEvent();
}
}
}
}
运行截图: