Problem:
Given a string, find the first non-repeating character in it and return it's index. If it doesn't exist, return -1. Examples: s = "leetcode" return 0. s = "loveleetcode", return 2. Note: You may assume the string contain only lowercase letters.
try:
class Solution { public int firstUniqChar(String s) { int[] counts = new int[26]; for(int i=0;i<s.length();i++){ counts[s.charAt(i)-'a']++; } for(int i=0;i<counts.length;i++){ if(counts[i]==1) return i; } return -1; } }
result:
想当然!
second:
import java.util.HashMap; import java.util.LinkedHashMap; class Solution { public int firstUniqChar(String s) { HashMap<Character, Integer> map = new LinkedHashMap<Character, Integer>(); for(int i=0;i<s.length();i++){ //if(map.get((Character)s.charAt(i))==null) map.put() Character c = s.charAt(i); Integer int = map.get(c); map.put(c, integer==null ? 1 : (++integer)); } for(MapEntry entry : map){ if(entry.getValue()==1) return entry.getKey(); } return -1; } }
result:
Run Code Status: Compile Error Run Code Result: Your input "leetcode" Your answer Line 10: error: not a statement Expected answer 0
被这个stupid的问题卡了好久。。因为leetcode的编辑器没有数据类型的关键字高亮提示,所以把这个问题忽略了,int是不能作为变量名称使用的。
扫描二维码关注公众号,回复:
84061 查看本文章
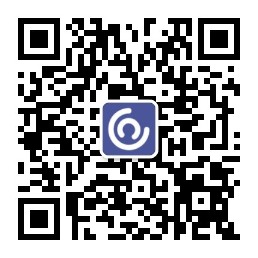
re-try:
import java.util.HashMap; import java.util.LinkedHashMap; import java.util.Map.Entry; class Solution { public int firstUniqChar(String s) { HashMap<Character, Integer> map = new LinkedHashMap<Character, Integer>(); for(int i=0;i<s.length();i++){ //if(map.get((Character)s.charAt(i))==null) map.put() Character c = s.charAt(i); Integer integer = map.get(c); map.put(c, integer==null ? 1 : (++integer)); } for(Map.Entry<Character, Integer> entry : map.entrySet()){ if(entry.getValue()==1){ return s.indexOf(entry.getKey()); } } return -1; } }
result:
分析:
用LinkedHashMap效率较低,应该可以找到更优方案。
re-try:
import java.util.HashSet; class Solution { public int firstUniqChar(String s) { int[] counts = new int[26]; char[] charArray = s.toCharArray(); for(char c : charArray){ counts[c-'a']++; } HashSet<Character> set = new HashSet<Character>(); for(int i=0;i<counts.length;i++){ if(counts[i]==1) set.add((char)('a'+i)); } for(int i=0;i<charArray.length;i++){ if(set.contains(charArray[i])) return i; } return -1; } }
result:
conclusion: