- 本代码描述如何确保当前一个线程使用某些资源时,同时其他线程无法使用该资源。
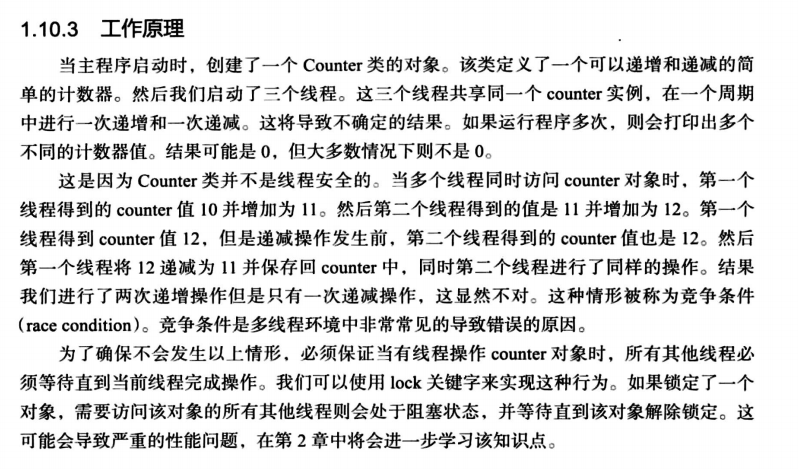
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace ConsoleApplication3
{
class Program
{
static void Main(string[] args)
{
#region 1.10
var c = new Counter();
var t1 = new Thread(() => Class1_10.TestCounter(c));
var t2 = new Thread(() => Class1_10.TestCounter(c));
var t3 = new Thread(() => Class1_10.TestCounter(c));
t1.Start();
t2.Start();
t3.Start();
t1.Join();
t2.Join();
t3.Join();
Console.WriteLine("有问题的 counter类");
Console.WriteLine("Total count:{0}",c.Count);//一个循环周期内先增1然后在减1,原则上最终结果是0,实际上可能是0,但是更多的是其他值(不确定)。原因:Counter是线程不安全的。
Console.WriteLine("-----");
Console.WriteLine("正确的 counter类");
var c1 = new CounterWithLock();
var tt1 = new Thread(() => Class1_10.TestCounter(c1));
var tt2 = new Thread(() => Class1_10.TestCounter(c1));
var tt3 = new Thread(() => Class1_10.TestCounter(c1));
tt1.Start();
tt2.Start();
tt3.Start();
tt1.Join();
tt2.Join();
tt3.Join();
Console.WriteLine("Total count:{0}", c1.Count);
#endregion
}
public class Class1_10
{
public static void TestCounter(CounterBase c)
{
for (int i = 0; i < 10000; i++)
{
c.Increment();//增量
c.Decrement();//减量
}
}
}
public class Counter : CounterBase
{
public int Count { get; private set; }
//递增
public override void Increment()
{
Count++;
}
//递减
public override void Decrement()
{
Count--;
}
}
public class CounterWithLock : CounterBase
{
private readonly object _syncRoot = new object();
public int Count { get; private set; }
//递增
public override void Increment()
{
lock (_syncRoot)
{
Count++;
}
}
//递减
public override void Decrement()
{
lock (_syncRoot)
{
Count--;
}
}
}
public abstract class CounterBase
{
public abstract void Increment();
public abstract void Decrement();
}
}