componentDidMount()页面加载完后执行
componentDidUpdate(prevProps)每次进页面都会无限调用,需要条件控制
componentWillReceiveProps(props) 这个是父组件有数据变化 子组件会及时更新的事件 如果你只执行一次 不用反复执行 就不用管
componentWillMount()页面销毁的时候执行
《《《---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------》》》
在单页面里面,父子组件传值是比较常见的,react的父子组件传值,和vue差不多的思路,父组件向子组件传值,父通过初始state,子组件通过this.props进行接收就可以了;子组件向父组件传值需要绑定一个事件,然后事件是父组件传递过来的this.props.event来进行值的更替,
父组件向子组件传值:
父组件Comment.js:
import React from "react"
import ComentList from "./ComentList"
class Comment extends React.Component {
constructor(props) {
super(props);
this.state = {
arr: [{
"userName": "fangMing", "text": "123333", "result": true
}, {
"userName": "zhangSan", "text": "345555", "result": false
}, {
"userName": "liSi", "text": "567777", "result": true
}, {
"userName": "wangWu", "text": "789999", "result": true
},]
}
}
render() {
return (
<div>
<ComentList arr={this.state.arr}> //这里把state里面的arr传递到子组件
</ComentList>
</div>
)
}
}
export default Comment;
子组件ComentList.js:
import
React from
"react"
class
ComentList
extends
React.Component {
constructor(props) {
super
(props);
}
render() {
return
(
<div className=
"list"
>
<ul>
{
this
.props.arr.map(item => {
//这个地方通过this.props.arr接收到父组件传过来的arr,然后在{}里面进行js的循环
return
(
<li key={item.userName}>
{item.userName} 评论是:{item.text}
</li>
)
})
}
</ul>
</div>
)
}
}
export
default
ComentList;
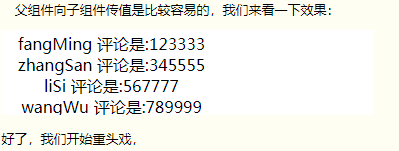
子组件向父组件传值,
同样是父组件:
import
React from
"react"
import
ComentList from
"./ComentList"
class
Comment
extends
React.Component {
constructor(props) {
super
(props);
this
.state = {
parentText:
"this is parent text"
,
arr: [{
"userName"
:
"fangMing"
,
"text"
:
"123333"
,
"result"
:
true
}, {
"userName"
:
"zhangSan"
,
"text"
:
"345555"
,
"result"
:
false
}, {
"userName"
:
"liSi"
,
"text"
:
"567777"
,
"result"
:
true
}, {
"userName"
:
"wangWu"
,
"text"
:
"789999"
,
"result"
:
true
},]
}
}
fn(data) {
this
.setState({
parentText: data
//把父组件中的parentText替换为子组件传递的值
},() =>{
console.log(
this
.state.parentText);
//setState是异步操作,但是我们可以在它的回调函数里面进行操作
});
}
render() {
return
(
<div>
//通过绑定事件进行值的运算,这个地方一定要记得.bind(this),
不然会报错,切记切记,因为通过事件传递的时候
this
的指向已经改变
<ComentList arr={
this
.state.arr} pfn={
this
.fn.bind(
this
)}>
</ComentList>
<p>
text is {
this
.state.parentText}
</p>
</div>
)
}
}
export
default
Comment;
import
React from
"react"
class
ComentList
extends
React.Component {
constructor(props) {
super
(props);
this
.state = ({
childText:
"this is child text"
})
}
clickFun(text) {
this
.props.pfn(text)
//这个地方把值传递给了props的事件当中
}
render() {
return
(
<div className=
"list"
>
<ul>
{
this
.props.arr.map(item => {
return
(
<li key={item.userName}>
{item.userName} 评论是:{item.text}
</li>
)
})
}
</ul>
//通过事件进行传值,如果想得到event,可以在参数最后加一个event,
这个地方还是要强调,
this
,
this
,
this
<button onClick={
this
.clickFun.bind(
this
,
this
.state.childText)}>
click me
</button>
</div>
)
}
}
export
default
ComentList;
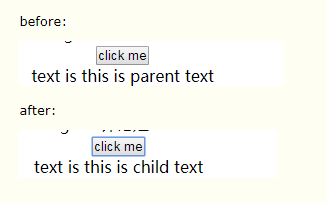