分支结构
应用场景
迄今为止,我们写的Python代码都是一条一条语句顺序执行,这种结构的代码我们称之为顺序结构。然而仅有顺序结构并不能解决所有的问题,比如我们设计一个游戏,游戏第一关的通关条件是玩家获得1000分,那么在完成本局游戏后我们要根据玩家得到分数来决定究竟是进入第二关还是告诉玩家“Game Over”,这里就会产生两个分支,而且这两个分支只有一个会被执行,这就是程序中分支结构。
1.if语句的使用
在python中,要构造分支结构可以使用if、elif和else关键字。
练习:用户的身份验证
代码:
username = input('user:')
password = input('pwd:')
if username == 'admin' and password == '123':
print('login')
else:
print('Error')
运行结果:

需要说明的是与其他语言不同的是,python中没有用花括号来构造代码而是使用了所进的方式来设置代码的层次结构,如果if
当然如果要构造出更多的分支,可以使用if…elif…else…
结构,例如下面的分段函数求值。
代码:
x = int(input('输入:'))
if x > 1:
f = 3 * x - 5
elif x < -1:
f = x + 2
else:
f = x * 5 + 3
print(f)
运行结果:

3.计算器
代码:
num4,num5 = map(float,input('请输入两个数:').split(','))
choose_method = input('choose method')
if choose_method == '+':
print('%f + %f = %f'%(num4,num5,num4 + num5))
elif choose_method == '-':
print(num4 - num5)
elif choose_method == '*':
print(num4 * num5)
elif choose_method == '/':
print(num4 / num5)
else:
raise KeyError('Only choose [+,-,*,/]')
运算结果:
4.石头剪刀布的游戏
代码:
import numpy as np
method = input('请出拳:')
computer = np.random.choice(['石头','剪刀','布'])
if method == '石头' and computer == '石头':
print('他出的 {} 平局呦!'.format(computer))
elif method == '石头' and computer == '剪刀':
print('他出的 {} 你赢了呢!'.format(computer))
elif method == '石头' and computer == '布':
print('他出的 {} 你输了呦!'.format(computer))
elif method == '剪刀' and computer == '石头':
print('他出的 {} 你输了呦!'.format(computer))
elif method == '剪刀' and computer == '剪刀':
print('他出的 {} 平局呦!'.format(computer))
elif method == '剪刀' and computer == '布':
print('他出的 {} 你赢了呢!'.format(computer))
elif method == '布' and computer == '石头':
print('他出的 {} 你赢了呦!'.format(computer))
elif method == '布' and computer == '剪刀':
print('他出的 {} 你输了呢!'.format(computer))
elif method == '布' and computer == '布':
print('他出的 {} 平局呦!'.format(computer))
运行结果:

5.输入三边的长度,判断是否是三角形,若是计算周长和面积
代码:
import math
a = float(input('请输入边长1:'))
b = float(input('请输入边长2:'))
c = float(input('请输入边长3:'))
if (a + b > c) and (a + c >b) and (b + c < a):
print('周长:%.1f'%(a+b+c))
p = (a+b+c)/2
area = math.sqrt(p * (p-a) * (p-b) * (p-c))
print('面积:%.1f'%area)
else:
print('这不是一个三角形')
运行结果:
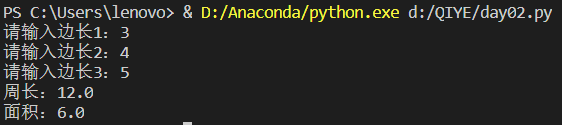