## 顺序结构
- 按照从上到下的顺序,一条语句一条语句的执行,是最基本的结构
## 分支结构
if condition:
statement
statement
...
elif condition>:
statement
statement
...
else:
statement
statement
...
- if 语句可以嵌套,但不推荐, 尽量保持代码简洁
- Python没有switch-case语句
- Python条件语句允许下列写法
x = 10
print(1 < x < 20) # -> True
print(1 < x < 20 < 100) # -> True
- 以下数据将被判定为False:
False、None、0、0.0、""、[]、()、{}、set()
## 循环结构
- 写循环程序时需要注意循环变量的初值,循环条件和循环变量的增量,三者共称为循环三要素
- while循环
count = 0 # -> 循环变量
while count < 5: # -> 循环条件
print(count, end=",") # -> 0,1,2,3,4,
count += 1 # -> 循环变量的增量,对循环变量进行修改
- for迭代
- 在C或者Java等语言中,for循环将循环三要素结合在一行语句中:大概如下:
for(int i = 0; i < 10; i++){循环体}
- 但Python中的for循环相对而言更加简洁
words = ["and", "or", "not"]
for word in words:
print(word, end=", ") # -> and, or, not,
- 列表、元组、字典、集合、字符串都是可以迭代的对象
- 对字典的迭代可以:
a_dict = {"name": "Stanley", "age": "22"}
for k, v in a_dict.items():
print("{0}: {1}".format(k, v))
# -> name: Stanley
age: 22
- 单独迭代字典的key或者value可以使用字典的keys()或values()函数
- break关键字
- 在循环体中使用break关键字,整个循环会立刻无条件停止
count = 0
while count < 5:
if count == 2:
break
print(count, end=" ")
count += 1
# -> 0 1
# -> 由于当count等于2时,进入if语句执行了break,所以循环结束,未完成的循环不再执行
- continue关键字
- 在循环体中使用continue关键字,此次循环无条件体停止,执行之后的循环
for i in range(0, 5):
if i == 2:
continue
print(i, end=" ")
# -> 0 1 3 4
# -> 当i等于2时进入if语句,执行continue,本次循环跳过,进入下一循环
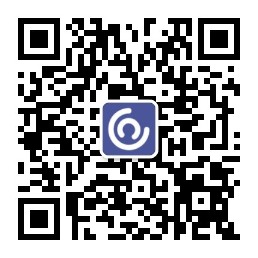
- 与循环一起使用else
for i in range(0, 5):
print(i, end=" ")
else:
print("循环结束")
# -> 0 1 2 3 4 循环结束
- 当循环完全结束后(不被break和cuntinue打断)执行else中的代码
- else同样适用于while循环
- 使用zip()并行迭代
numbers = [1, 2, 3, 4]
words = ["one", "two", "three", "four"]
days = ["Mon.", "Tues.", "Wed.", "Thur."]
for number, word, day in zip(numbers, words, days):
print(number, word, day)
输出:
1 one Mon.
2 two Tues.
3 three Wed.
4 four Thur.
- zip()函数在长度最小的参数中的元素用完后自动停止,其他参数未使用的元素将被略去,除非手动扩展其他较短的参数长度
- zip()函数的返回值不是列表或元组,而是一个整合在一起的可迭代变量
list(zip(words, days))
# -> [('one', 'Mon.'), ('two', 'Tues.'), ('three', 'Wed.'), ('four', 'Thur.')]
本文参考书籍:[美]Bill Lubanovic 《Python语言及其应用》