实验题目1:
创建一个简单的银行程序包
实验目的:
Java语言中面向对象的封装性及构造器的创建与使用。
实验说明:
在这个练习里,创建一个简单版本的Account类。将这个文件放入banking程序包中。在创建单个账户的默认程序包中,已编写了一个测试程序TestBanking。这个测试程序初始化账户余额,并可执行几种简单的事务处理。最后,该测试程序显示该账户的最终余额。
提示:
- 创建banking包;
- 在banking包下创建Account类。该类必须实现上述UML框图中的模型。
- 声明一个私有对象属性:balance,这个属性保留了银行账户的当前(或即时)余额。
- 声明一个带有一个参数(init_balance)的公有构造器,这个参数为balance属性赋值。
- 声明一个公有方法getBalance,该方法用于获取账户余额。
- 声明一个公有方法deposit,该方法向当前余额增加金额。
- 声明一个公有方法withdraw从当前余额中减去金额。
- 打开TestBanking.java文件,按提示完成编写,并编译TestBanking.java文件。
- 运行TestBanking类。可以看到下列输出结果。
程序代码实现:
(1)、banking包中的Account类:
package banking;
public class Account {
public double balance; //账户余额
public Account(double init_balance) {
balance=init_balance;
}
public double getBalance() {
return balance;
}
//存钱
public void deposit(double amt) {
balance+=amt;
}
//取钱
public void withdraw(double amt) {
if(balance>=amt) {
balance-=amt;
}else {
System.out.println("余额不足!");
}
}
}
(2)、testbanking包中的TestBanking类:
package testbanking;
import banking.Account;
public class TestBanking {
public static void main(String[] args) {
Account account;
//Create an account that can has a 500.00 balance
account=new Account(500);
System.out.println("Create an account with a 500.00 balance.");
//code
account.withdraw(150.0);
System.out.println("Withdraw 150.00");
//code
account.deposit(22.5);
System.out.println("Deposit 22.50");
//code
account.withdraw(47.62);
System.out.println("Withdraw 47.62");
//code
//print out the final account balance
System.out.println("The account has a balance of"+account.getBalance());
}
}
实验题目二:
扩展银行项目,添加一个Customer类。Customer类将包含一个Account对象。
实验目的:
使用引用类型的成员变量。
扫描二维码关注公众号,回复:
6749544 查看本文章
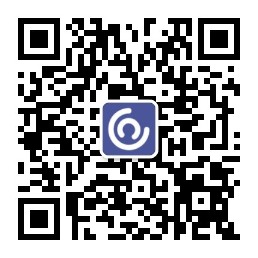
提示:
- 在banking包下的创建Customer类。该类必须实现上面的UML图表中的模型。
a、声明三个私有对象的对象属性:firstName、lastName和account。
b、声明一个公有构造器,这个构造器带有两个带有对象属性的参数(f和l)
c、声明两个公有存储器来访问对象属性,方法getFirstName和getLastName返回相应的属性
d、声明setAccount方法来对account赋值。
e、声明getAccount方法以获取account的属性。
2、在exercise主目录里,编译运行这个TestBanking程序。应该看到如下输出结果。
程序代码实现:
(1)、包banking2中的Account类:
package banking2;
public class Account {
public double balance; //账户余额
public Account(double init_balance) {
balance=init_balance;
}
public double getBalance() {
return balance;
}
//存钱
public void deposit(double amt) {
balance+=amt;
}
//取钱
public void withdraw(double amt) {
if(balance>=amt) {
balance-=amt;
}else {
System.out.println("余额不足!");
}
}
}
(2)、包banking2中的Customer类:
package banking2;
public class Customer {
private String firstName;
private String lastName;
private Account account; //定义一个Account类型的变量account(Account类型为自定义的一个类);
public Customer(String f,String l) {
firstName=f;
lastName=l;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public void setAccount(Account acct) { //setAccount方法用来设置形参为类Account定义的变量用于赋值;
account=acct;
}
public Account getAccount() { //返回Account类定义的Account类型的值.
return account;
}
}
(3)、包testbanking中的TestBanking1类:
package testbanking;
import banking2.Account;
import banking2.Customer;
public class TestBanking1 {
public static void main(String[] args) {
//声明两个对象
Customer customer;
Account account;
//Create an account that can has a 500.00 balance.
account=new Account(500.00); //(构造器)实例化创建一个账户,里面有500元。如果没有此语句,以后的account会出现错误。
System.out.println("Creating the customer Jane Smith.");
customer=new Customer("Jane","Smith"); //(构造器)创建一个客户,如果没有此语句,以后的customer会有错误导致不能用。
customer.setAccount(account); //为一个客户创建一个有钱的账户。用于存钱、取钱等等
//code
System.out.println("Creating her account with a 500.00 balance.");
//code
customer.getAccount().withdraw(150.00);
System.out.println("Withdraw 150.0");
//code
customer.getAccount().deposit(22.50);
System.out.println("Deposit 22.50");
//code
customer.getAccount().withdraw(47.62);
System.out.println("Withdraw 47.62");
//code
//Print out the final account balance.(打印账户余额)
System.out.println("Customer ["+customer.getLastName()+","+customer.getFirstName()+"] has a balance of "+account.getBalance()); //customer.getAccount().getBalance()
}
}