版权声明:本文为博主原创文章。转载请联系博主授权。博主微信公众号【知行校园汇】。 https://blog.csdn.net/cxh_1231/article/details/84454016
- 注:这是WHUT 计算机学院 移动终端应用开发课程、实验1:SharedPreferences的应用 的文章。
- 源码下载地址:https://download.csdn.net/download/cxh_1231/10805430
- >>点击查看WUTer计算机专业实验汇总
- 纸上得来终觉浅,觉知此事需躬行!
一、实验目的:
- 熟悉Android应用程序的开发环境和开发过程;
- 熟悉Android应用程序的基本框架;
- 掌握Activity组件的创建与使用方法;
- 掌握Android布局管理和基本控件的使用方法;
- 熟悉Android数据存储方案,掌握SharedPreferences的使用方法。
二、实验内容:
- 新建一个Android应用程序,设计一个能够输入用户姓名、密码、电话、邮箱和性别等信息的界面。
- 为界面添加“保存”、“读取”和“清空”等按钮,并设计按钮响应代码。“保存”按钮将界面输入信息保存于SharedPrefences共享文件;“读取”按钮从共享文件中读取信息显示于界面上。“清空”按钮清空界面信息。
- 退出应用程序或关机时自动保存界面信息到共享文件。
三、实验指导:
- 新建一个Android应用程序,设计一个GUI界面,效果图如图1所示。
- 图1中下拉列表采用Spinner控件实现。该控件的使用方法与ListView控件类似,在加载数据时需要一个Adapte对象,并在创建Adapter对象的过程中指定要加载的数据(数组或List对象)。关键代码如下:
private String[] gender_list = new String[]{"男", "女"};
Spinner sp_gender = (Spinner) findViewById(R.id.sp_gengder);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(MainActivity.this,R.layout.spinner_item, gender_list);
sp_gender.setAdapter(adapter);
//获取下拉列表当前列项的内容
String user_gender = (String)sp_gender.getSelectedItem();
- 将数据存储到SharedPreferences中的基本步骤如下:
(1)使用Context类的getSharedPreferences(String name, int mode) 方法获得SharedPreferences对象;
(2)使用SharedPreferences对象的edit()方法获得一个SharedPreferences.Editor对象。
(3)使用SharedPreferences.Editor对象的putXxx()方法(如putString()、putInt()等)向SharedPreferences.Editor对象中添加数据。
(4)调用apply()方法将添加的数据提交。
例如: -
SharedPreferences pref = getSharedPreferences("user_info", MODE_PRIVATE); SharedPreferences.Editor editor = pref.edit(); editor.putString("user_name", "Tom"); // 存入数据 editor.apply(); // 提交修改
- 从SharedPreferences中读取数据的方法
(1)使用Context类的getSharedPreferences(String name, int mode)获得SharedPreferences对象。
(2)使用SharedPreferences对象的getXxx()方法(如getString()、getInt()等)从SharedPreferences中读取数据。
例如:
SharedPreferences pref = getSharedPreferences("user_info", MODE_PRIVATE);
String userName = pref.getString("user_name", ""); //获取数据
四、过程记录:
1、activity_main.xml布局设计:
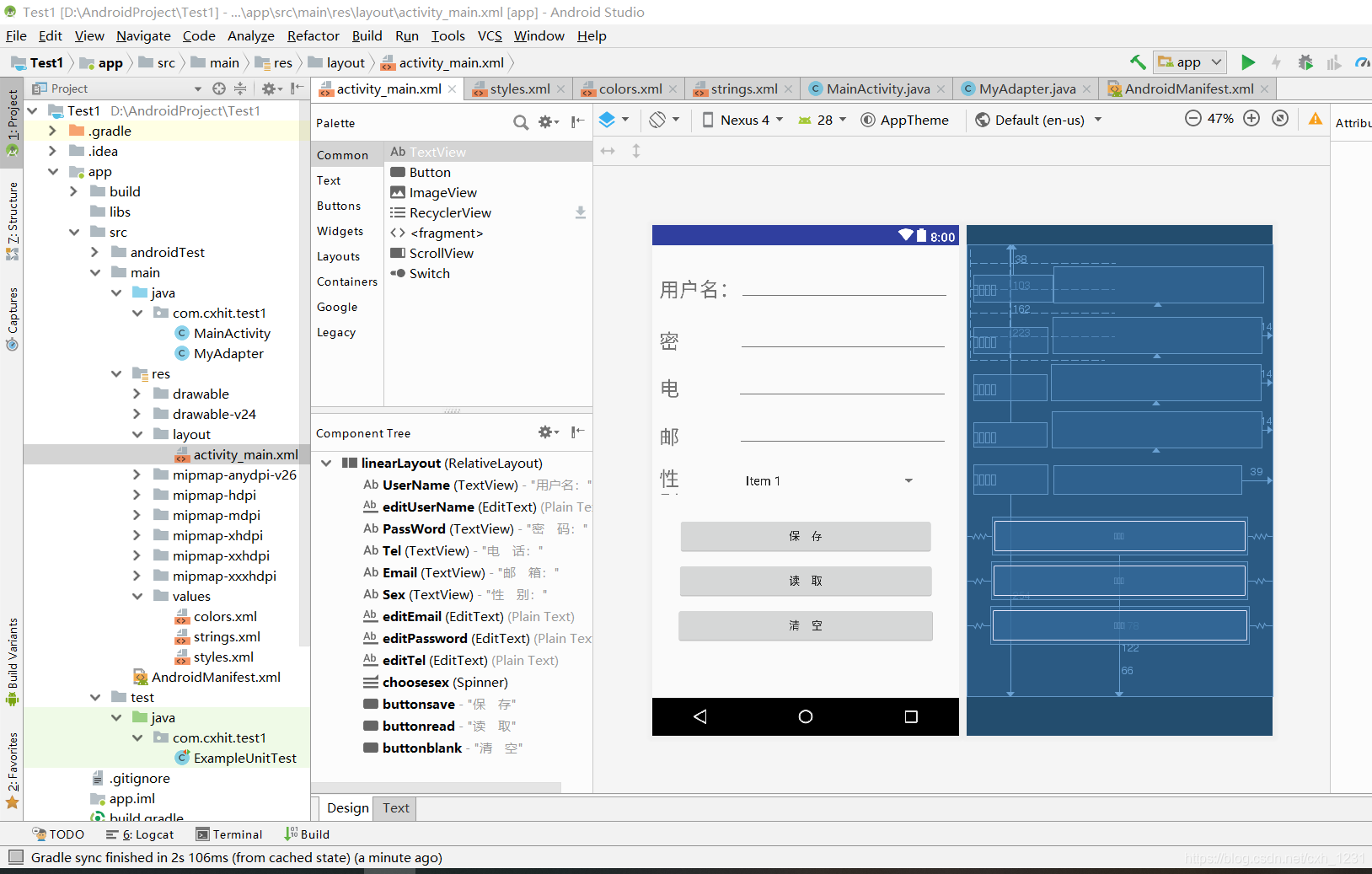
2、activity_main代码:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/linearLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/UserName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_marginTop="38dp"
android:layout_toStartOf="@+id/choosesex"
android:text="用户名:"
android:textSize="50px" />
<EditText
android:id="@+id/editUserName"
android:layout_width="263dp"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/UserName"
android:layout_toEndOf="@+id/UserName"
android:ems="10"
android:inputType="textPersonName"
android:text="" />
<TextView
android:id="@+id/PassWord"
android:layout_width="93dp"
android:layout_height="33dp"
android:layout_alignParentTop="true"
android:layout_alignStart="@+id/UserName"
android:layout_marginTop="103dp"
android:text="密 码:"
android:textSize="50px" />
<TextView
android:id="@+id/Tel"
android:layout_width="92dp"
android:layout_height="33dp"
android:layout_alignParentTop="true"
android:layout_alignStart="@+id/UserName"
android:layout_marginTop="162dp"
android:text="电 话:"
android:textSize="50px" />
<TextView
android:id="@+id/Email"
android:layout_width="92dp"
android:layout_height="31dp"
android:layout_alignParentTop="true"
android:layout_alignStart="@+id/UserName"
android:layout_marginTop="223dp"
android:text="邮 箱:"
android:textSize="50px" />
<TextView
android:id="@+id/Sex"
android:layout_width="93dp"
android:layout_height="38dp"
android:layout_alignParentBottom="true"
android:layout_alignStart="@+id/UserName"
android:layout_marginBottom="254dp"
android:text="性 别:"
android:textSize="50px" />
<EditText
android:id="@+id/editEmail"
android:layout_width="263dp"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/Email"
android:layout_alignParentEnd="true"
android:layout_marginEnd="14dp"
android:ems="10"
android:inputType="textPersonName"
android:text="" />
<EditText
android:id="@+id/editPassword"
android:layout_width="262dp"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/PassWord"
android:layout_alignParentEnd="true"
android:layout_marginEnd="14dp"
android:ems="10"
android:inputType="textPersonName"
android:text="" />
<EditText
android:id="@+id/editTel"
android:layout_width="264dp"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/Tel"
android:layout_alignParentEnd="true"
android:layout_marginEnd="14dp"
android:ems="10"
android:inputType="textPersonName"
android:text="" />
<Spinner
android:id="@+id/choosesex"
android:layout_width="236dp"
android:layout_height="36dp"
android:layout_alignBottom="@+id/Sex"
android:layout_alignParentEnd="true"
android:layout_marginEnd="39dp" />
<Button
android:id="@+id/buttonsave"
android:layout_width="320dp"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="178dp"
android:onClick="buttonsave_Click"
android:text="保 存" />
<Button
android:id="@+id/buttonread"
android:layout_width="322dp"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="122dp"
android:onClick="buttonread__Click"
android:text="读 取" />
<Button
android:id="@+id/buttonblank"
android:layout_width="325dp"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="66dp"
android:onClick="buttonclean__Click"
android:text="清 空" />
</RelativeLayout>
3、MainActivity.java文件代码:
package com.cxhit.test1;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Spinner;
import android.widget.Toast;
import android.content.SharedPreferences;
import android.widget.Button;
import android.widget.EditText;
import android.widget.*;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private EditText getUserName,getUserPassword,getUserTel,getUserEmail;
private Spinner getUserSex;
private Button saveButton,readButton,cleanButton;
////声明Sharedpreferenced对象
private SharedPreferences sp;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Spinner spinner = (Spinner) findViewById(R.id.choosesex);
final List<String> datas = new ArrayList<>();
datas.add("男");
datas.add("女");
MyAdapter adapter = new MyAdapter(this);
spinner.setAdapter(adapter);
adapter.setDatas(datas);
saveButton = (Button) findViewById(R.id.buttonsave);
readButton = (Button) findViewById(R.id.buttonsave);
cleanButton = (Button) findViewById(R.id.buttonblank);
}
public void buttonsave_Click(View view) {
getUserName = (EditText) findViewById(R.id.editUserName);
getUserPassword = (EditText) findViewById(R.id.editPassword);
getUserTel = (EditText) findViewById(R.id.editTel);
getUserEmail = (EditText) findViewById(R.id.editEmail);
getUserSex = (Spinner) findViewById(R.id.choosesex);
SharedPreferences pref = getSharedPreferences("user_info",MODE_PRIVATE);
SharedPreferences.Editor editor =pref.edit();
editor.putString("username",getUserName.getText().toString());
editor.putString("userpassword",getUserPassword.getText().toString());
editor.putString("usertel",getUserTel.getText().toString());
editor.putString("useremail",getUserEmail.getText().toString());
editor.putString("usersex",getUserSex.getSelectedItem().toString());
editor.apply();
Toast.makeText(MainActivity.this,"保存成功",Toast.LENGTH_LONG).show();
}
public void buttonread__Click(View view)
{
getUserName = (EditText) findViewById(R.id.editUserName);
getUserPassword = (EditText) findViewById(R.id.editPassword);
getUserTel = (EditText) findViewById(R.id.editTel);
getUserEmail = (EditText) findViewById(R.id.editEmail);
getUserSex = (Spinner) findViewById(R.id.choosesex);
SharedPreferences pref = getSharedPreferences("user_info",MODE_PRIVATE);
getUserName.setText(pref.getString("username",""));
getUserPassword.setText(pref.getString("userpassword",""));
getUserTel.setText(pref.getString("usertel",""));
getUserEmail.setText(pref.getString("useremail",""));
SpinnerAdapter spAdapter = getUserSex.getAdapter();
int k = spAdapter.getCount();
for (int i=0;i<k;i++)
{
if(pref.getString("usersex","").equals(spAdapter.getItem(i)))
{
getUserSex.setSelection(i);
break;
}
}
Toast.makeText(MainActivity.this,"读取成功",Toast.LENGTH_LONG).show();
}
public void buttonclean__Click(View view)
{
getUserName = (EditText) findViewById(R.id.editUserName);
getUserPassword = (EditText) findViewById(R.id.editPassword);
getUserTel = (EditText) findViewById(R.id.editTel);
getUserEmail = (EditText) findViewById(R.id.editEmail);
getUserSex = (Spinner) findViewById(R.id.choosesex);
getUserSex.setSelection(0);
getUserName.setText("");
getUserPassword.setText("");
getUserEmail.setText("");
getUserTel.setText("");
Toast.makeText(MainActivity.this,"清空成功",Toast.LENGTH_LONG).show();
}
}
五、运行结果:
六、写在最后:
今天晚上刚考完移动终端应用开发,才将这片文章发出来。
其实,Android开发的两次实验并不难,也是Android必须zhan掌握的基本知识。今晚上考试的两道程序设计题就是这次实验的两道题,即分别使用SharedPreferences和SQLitebaoc保存TestView输入的用户名和密码。
本文内容仅供参考。