人工智能深度学习、机器学习 公众号:机器工匠
学习目标:
- 理解Pytorch 的 Tensor库,以及神经网络。
- 训练一个简单的图像分类网络。
假设已经了解numpy的基本用法,并确保已经安装好torch和torchvision。
什么是Pytorch
Pytorch是一个基于Python的科学计算包,用于以下两个目的:
- 代替NumPy,使用GPU的加速能力。
- 用于提供最大灵活性和速度的深度学习研究平台。
张量(Tensors)
张量与NumPy的数组类似,但是张量可以用在GPU上用来加速计算。
现在我们用Pytorch构造一个未经初始化的5×3的矩阵:
x = torch.empty(5, 3)
print(x)
结果:
tensor([[0.0000e+00, 0.0000e+00, 0.0000e+00],
[0.0000e+00, 0.0000e+00, 0.0000e+00],
[0.0000e+00, 0.0000e+00, 0.0000e+00],
[0.0000e+00, 7.6231e-43, 0.0000e+00],
[0.0000e+00, 2.9966e+32, 0.0000e+00]])
构造一个随机初始化的矩阵:
x = torch.rand(5, 3)
print(x)
结果:
tensor([[0.4140, 0.7152, 0.0888],
[0.6878, 0.9607, 0.2579],
[0.0088, 0.5816, 0.1782],
[0.3815, 0.6752, 0.9996],
[0.4057, 0.3032, 0.8977]])
用0初始化矩阵,并且数据类型为long:
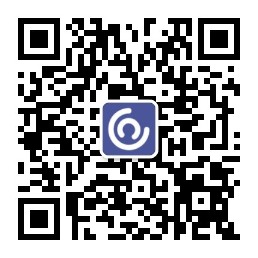
x = torch.zeros(5, 3, dtype=torch.long)
print(x)
结果:
tensor([[0, 0, 0],
[0, 0, 0],
[0, 0, 0],
[0, 0, 0],
[0, 0, 0]])
直接从数据中构造张量:
x = torch.tensor([5.5, 3])
print(x)
结果:
tensor([5.5000, 3.0000])
从现有tensor构造另外一个tensor,这会重用输入tensor的一些属性,比如数据类型,除非用户指定另外的值。
x = x.new_ones(5, 3, dtype=torch.double) # new_* 方法接收tensor大小参数
print(x)
x = torch.randn_like(x, dtype=torch.float) # 重载数据类型!
print(x) # 结果有相同的大小
结果:
tensor([[1., 1., 1.],
[1., 1., 1.],
[1., 1., 1.],
[1., 1., 1.],
[1., 1., 1.]], dtype=torch.float64)
tensor([[ 0.6743, -0.3788, 0.7561],
[-0.7612, -1.6943, -1.2451],
[ 0.6169, -1.1207, -1.1664],
[ 0.4309, 1.3055, -0.0481],
[ 0.7891, -1.7696, 1.4875]])
获取tensor的大小:
print(x.size())
结果:
torch.Size([5, 3])
注意:torch.Size实际上是一个tuple,因此它支持所有的tuple操作。
操作(Operations)
操作有许多种语法,下面将展示操作的几种语法。
加法:语法一
y = torch.rand(5, 3)
print(x + y)
结果:
tensor([[1.2953, 0.3779, 0.9404],
[0.8671, 0.5968, 0.7860],
[1.3698, 1.2983, 1.1537],
[0.3706, 0.6296, 0.7085],
[0.8819, 0.8260, 0.9962]])
加法:语法二
print(torch.add(x, y))
结果:
tensor([[1.2953, 0.3779, 0.9404],
[0.8671, 0.5968, 0.7860],
[1.3698, 1.2983, 1.1537],
[0.3706, 0.6296, 0.7085],
[0.8819, 0.8260, 0.9962]])
加法:将结果存入另外一个tensor
result = torch.empty(5, 3)
torch.add(x, y, out=result)
print(result)
结果:
tensor([[1.2953, 0.3779, 0.9404],
[0.8671, 0.5968, 0.7860],
[1.3698, 1.2983, 1.1537],
[0.3706, 0.6296, 0.7085],
[0.8819, 0.8260, 0.9962]])
加法:in-place(在被加数上加上加数,因此会改变被加数的值)
# 把x加在y上
y.add_(x)
print(y)
结果:
tensor([[1.2953, 0.3779, 0.9404],
[0.8671, 0.5968, 0.7860],
[1.3698, 1.2983, 1.1537],
[0.3706, 0.6296, 0.7085],
[0.8819, 0.8260, 0.9962]])
注意:任何带有下划线(_)的操作,都会改变tensor的值,比如
x.copy_(y)
,x.t_()
都会改变x的值。
在tensor上还可以尽情使用类似NumPy的索引方法:
print(x[:, 1])
结果:
tensor([0.1801, 0.1028, 0.7038, 0.3089, 0.1738])
使用torch.view来改变tensor大小:
x = torch.randn(4, 4)
y = x.view(16)
z = x.view(-1, 8) # -1表示这个维度的数值通过其他维度推算而出
print(x.size(), y.size(), z.size())
结果:
torch.Size([4, 4]) torch.Size([16]) torch.Size([2, 8])
如果tensor中只有1个值,那么通过.item()
可以取得:
x = torch.randn(1)
print(x)
print(x.item())
结果:
tensor([0.3665])
0.36647817492485046
对接NumPy
下面介绍Torch Tensor 和 NumPy之间如何相互转换。
Torch Tensor和NumPy会共享数据底层的内存存储位置,所以修改其中一个也会改变另外一个的值。
Torch Tensor转成NumPy数组:
a = torch.ones(5)
print(a)
结果:
tensor([1., 1., 1., 1., 1.])
b = a.numpy()
print(b)
结果:
[1. 1. 1. 1. 1.]
现在我们改变tensor中的值,看看NumPy数组中的值是什么:
a.add_(1)
print(a)
print(b)
结果:
tensor([2., 2., 2., 2., 2.])
[2. 2. 2. 2. 2.]
从结果可以看出,在tensor上加上了1,由它转成的NumPy数组上的数值也随之修改了。
NumPy数组转Torch Tensor
和上面一样,由NumPy数组生成的Tensor,如果修改了数组的值,对应的Tensor也会随之修改:
import numpy as np
a = np.ones(5)
b = torch.from_numpy(a)
np.add(a, 1, out=a)
print(a)
print(b)
结果:
[2. 2. 2. 2. 2.]
tensor([2., 2., 2., 2., 2.], dtype=torch.float64)
CPU上的Tensor,除类型为CharTensor之外,都能和NumPy互转。
CUDA Tensors
通过.to
方法可以将tensor移到任何CUDA设备上:
# 如果支持CUDA设备
# 使用 ``torch.device`` 将tensor移入或移出GPU
if torch.cuda.is_available():
device = torch.device("cuda") # CUDA设备对象
y = torch.ones_like(x, device=device) # 直接在GPU上创建
x = x.to(device) # 或者使用 ``.to("cuda")``将tensor放到GPU上
z = x + y
print(z)
print(z.to("cpu", torch.double)) # ``.to`` 也能同时修改数据类型!
结果:
tensor([-0.7816], device='cuda:0')
tensor([-0.7816], dtype=torch.float64)