62. Unique Paths
Medium
1473104FavoriteShare
A robot is located at the top-left corner of a m x n grid (marked 'Start' in the diagram below).
The robot can only move either down or right at any point in time. The robot is trying to reach the bottom-right corner of the grid (marked 'Finish' in the diagram below).
How many possible unique paths are there?
Above is a 7 x 3 grid. How many possible unique paths are there?
Note: m and n will be at most 100.
Example 1:
Input: m = 3, n = 2 Output: 3 Explanation: From the top-left corner, there are a total of 3 ways to reach the bottom-right corner: 1. Right -> Right -> Down 2. Right -> Down -> Right 3. Down -> Right -> Right
Example 2:
Input: m = 7, n = 3 Output: 28
这道题一会儿写好了,小的数可以,但大数字超时。下面的是超时的代码。思路是用递归,如果x或y坐标没有到达边界则存在两种可能,然后再分别计算两种可能。如下图,黄色格子的话增加一条可能路径,红色格子不增加可能路径。
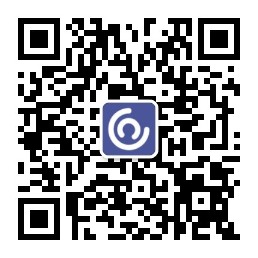
class Solution {
public:
int p=2;
void go(int x,int y,int mX,int mY)
{
if(x==mX||y==mY)return;
p++;
go(x+1,y,mX,mY);
go(x,y+1,mX,mY);
}
int uniquePaths(int m, int n) {
int x=0;
int y=0;
int possible;
if(m==0||n==0)return 0;
if(m==1||n==1)return 1;
go(1,0,m-1,n-1);
go(0,1,m-1,n-1);
return p;
}
};
下面用动态规划的方法做:
参考:https://blog.csdn.net/yuanliang861/article/details/83513788
在这个矩形网格框内,第一行和第一列中的每一位置,到达的可能路径都为1。对其他位置,到达的可能路径数量为其正上面位置对应路径的数量加上左边路径的数量(因为只可以向下走或者向右走)。如下表:
代码自己写一遍:
class Solution {
public:
int uniquePaths(int m, int n) {
if(m==0||n==0)return 0;
if(m==1||n==1)return 1;
vector<int> t(m,1);
vector<vector<int>> p(n,t);
for(int x=1;x<n;x++)
{
for(int y=1;y<m;y++)
{
p[x][y]=p[x-1][y]+p[x][y-1];
}
}
return p[n-1][m-1];
}
};