01.方法:
- 定义:一段特定功能的代码块,提高程序的复用性和可读性;
- 格式:访问权限修饰符+[其他修饰符]+返回值类型+方法名(形参列表:参数类型+参数名){方法体};
- 形参:方法定义时的参数;
- return:结束带返回值的方法;
import java.util.Scanner;
public class Test {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("请输入年份:");
int year = input.nextInt();
boolean bool = isRunYear(year);
if (bool){
System.out.println("是闰年");
} else {
System.out.println("是平年");
}
}
public static boolean isRunYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return true;
} else {
return false;
}
}
}
- 方法的重载(overloading):在类中可以创建多个方法,具有相同的名字,但具有不同的参数、不同的定义(返回值不能作为重载的条件);
public class Test {
public static int add(int a,int b) {
return a+b;
}
public static float add(float a,float b) {
return a+b;
}
public static float add(int a,float b) {
return a+b;
}
}
02.数组
- 定义:一组能够存储相同数据类型的变量的集合;
- 赋值方式:有四种;
2.1 使用默认初始值初始化数组中的每一个元素:int[] scores=new int[3];
2.2 先声明,再赋予默认的初始值:int[] scores; scores=new int[3];
2.3 先声明,再使用指定的值初始化:int[] scores=new int[]{56,78,98};
2.4 使用数组常量值给数组进行赋值:int[] scores={56,78,98};
- 注意:定义数组一定要有长度,每个数据称为元素,数组下标从0开始;
- 数组的遍历(迭代):
public class Test {
public static void main(String[] args) {
int[] scores = {59,75,84,93,100};
int len = scores.length;
for(int i=0;i<len;i++){
int score = scores[i];
System.out.println(score);
}
for(int score:scores){
System.out.println(score);
}
print(59,75,84,93,100);
}
public static void print(int... score){
int len = score.length;
for(int i=0;i<len;i++){
System.out.println(score[i]);
}
}
}
- 使用数组时要注意避免:空指针异常和数组越界异常;
- 数组内存:
6.1 栈内存:大小固定,用于存储局部、临时变量(基本数据类型)和引用变量;
6.2 堆内存:大小不固定,用于存放对象;
6.3 变量存的是地址,栈内存指向堆内存的首地址;
6.4 数组是引用类型,会存放在堆内存;
- 数组示例:
public class Test {
public static void main(String[] args) {
int[] nums = {12,23,14,20,8,45};
Scanner scann = new Scanner(System.in);
System.out.println("输入你猜的数(50以内):");
int input = scann.nextInt();
boolean flag=false;
for(int num:nums){
if(input==num){
flag=true;
break;
}
}
if(flag){
System.out.println("猜对了!");
}else{
System.out.println("猜错了。。。");
}
}
public static void main(String[] args) {
char[] ch = {'A','B','C','D','E','F','G'};
int len = ch.length;
for(int i=1;i<=len;i++){
for(int j=i;j<len;j++){
System.out.print(" ");
}
for(int j=1;j<=i*2-1;j++){
System.out.print(ch[i-1]);
}
System.out.println();
}
}
int[] [] scores = {{10,12},{15,8},{20,18}};
}
- 多维数组:Java中没有真正的多维数组,只是数组当中的元素还是数组;
- 最大值和最小值算法:
public class Test {
public static void main(String[] args) {
int[] nums = {49,30,12,84,28,76};
System.out.println("最大值:"+maxNum(nums));
System.out.println("最小值:"+minNum(nums));
}
public static int maxNum(int[] nums){
int max = nums[0];
int len = nums.length;
for(int i=1;i<len;i++){
if(nums[i]>max){
nums[i]=nums[i]+max;
max=nums[i]-max;
nums[i]=nums[i]-max;
}
}
return max;
}
public static int minNum(int[] nums){
int min = nums[0];
int len = nums.length;
for(int i=1;i<len;i++){
if(nums[i]<min){
nums[i]=nums[i]+min;
min=nums[i]-min;
nums[i]=nums[i]-min;
}
}
return min;
}
}
03.排序算法
- 冒泡排序:比较相邻元素,谁大谁放后面,直到最大的放到最后面;重复上面的步骤,直至就剩最后一个;相同元素的前后顺序并没有改变,所以冒泡排序是一种稳定排序算法。
public class Test {
public static void main(String[] args) {
int[] nums = {49,30,12,84,28,76};
for(int i=0;i<nums.length-1;i++){
for(int j=0;j<nums.length-1-i;j++){
if(nums[j]>nums[j+1]){
nums[j]=nums[j+1]+nums[j];
nums[j+1]=nums[j]-nums[j+1];
nums[j]=nums[j]-nums[j+1];
}
}
}
for(int num:nums){
System.out.print(num+" ");
}
}
}
- 选择排序:每一次从待排序的数据元素中选取最小(或最大)的一个元素,顺序放在已排好序的数列的最后,直到全部待排元素排完;相同元素有可能调换位置,选择排序是不稳定的排序算法。
public class Test {
public static void main(String[] args) {
int[] nums = {49,30,12,84,28,76};
int minIndex=0;
for(int i=0;i<nums.length-1;i++){
minIndex = i;
for(int j=i+1;j<nums.length;j++){
if(nums[j]<nums[minIndex]){
minIndex = j;
}
}
if(minIndex!=i){
nums[i]=nums[i]+nums[minIndex];
nums[minIndex]=nums[i]-nums[minIndex];
nums[i]=nums[i]-nums[minIndex];
}
}
for(int num:nums){
System.out.print(num+" ");
}
}
}
- 插入排序:记录待排序的数,在已排好序的序列中,从后往前,找到合适的位置插入。
public class Test {
public static void main(String[] args) {
int[] nums = {49,30,12,84,28,76};
for(int i=1;i<nums.length;i++){
int temp=nums[i];
int j=0;
for(j=i-1;j>=0;j--){
if(nums[j]>temp){
nums[j+1]=nums[j];
}else{
break;
}
}
if(nums[j+1]!=temp){
nums[j+1]=temp;
}
}
for(int num:nums){
System.out.print(num+" ");
}
}
}
- 二分查找:在已排好序的数组中,将待查找元素和中间索引值对应的元素比较,若大于中间索引值对应的元素,去右半部分查找;否则去左半边查找。
public class Test {
public static void main(String[] args) {
int[] nums = {49,30,12,84,28,76};
for(int i=1;i<nums.length;i++){
int temp=nums[i];
int j=0;
for(j=i-1;j>=0;j--){
if(nums[j]>temp){
nums[j+1]=nums[j];
}else{
break;
}
}
if(nums[j+1]!=temp){
nums[j+1]=temp;
}
}
for(int num:nums){
System.out.print(num+" ");
}
int index = search(nums,28);
System.out.println(index);
}
public static int search(int[] nums,int key){
int start = 0;
int end = nums.length-1;
while(start<=end){
int middle = (start+end)/2;
if(nums[middle]>key){
end=middle-1;
}else if(nums[middle]<key){
start=middle+1;
}else{
return middle;
}
}
return -1;
}
}
04.Arrays工具类
- 用来操作数组的各种方法(比如排序、搜索);
1.1 二分查找:Arrays.binarySearch(int[] array,int value);
1.2 数组内容转换成字符串输出:Arrays.toString(int[] array);
1.3 排序(快速排序):Arrays.sort(int[] array);
1.4 复制指定数组:Arrays.copyOf(int[] array,int length),Arrays.copyOf(int[] array,int from,int to)–>System.arraycopy(num,0,newnum,0,num.length)(int[] newnum= new int [num.length]);
1.5 判断数组是否相等:Arrays.equal();
1.6 使用指定元素填充数组:Arrays.fill();
05.双色球案例
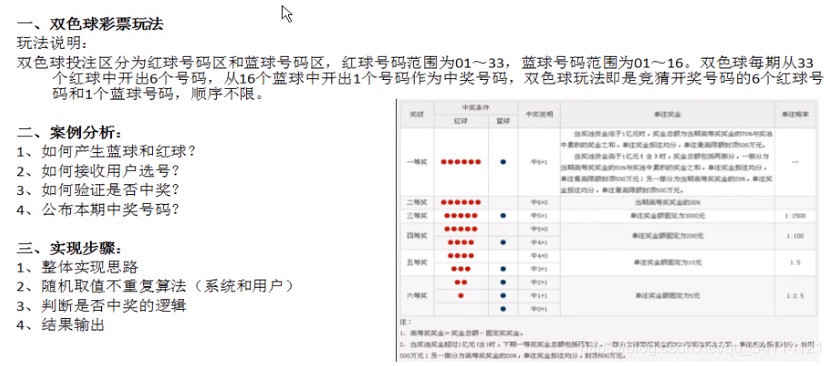
import java.util.*;
public class Test {
public static void main(String[] args) {
int[] userRedBall = new int[6];
int[] sysRedBall = new int[6];
int userBlueBall = 0;
int sysBlueBall = 0;
int redCount = 0;
int blueCount = 0;
int[] redBall = new int[33];
for (int i = 0; i < redBall.length; i++) {
redBall[i] = i + 1;
}
System.out.println("Begin!");
System.out.println("1.机选;2.手选");
Scanner scann = new Scanner(System.in);
Random r = new Random();
boolean flag = true;
while (flag) {
int isAuto = scann.nextInt();
switch (isAuto) {
case 1:
comSelection(redBall, userRedBall);
userBlueBall = r.nextInt(16) + 1;
flag = false;
break;
case 2:
System.out.println("6个红球号码:1-33");
for (int i = 0; i < userRedBall.length; i++) {
userRedBall[i] = scann.nextInt();
}
System.out.println("1个篮球号码:1-16");
userBlueBall = scann.nextInt();
flag = false;
break;
default:
System.out.println("1.机选;2.手选");
break;
}
}
comSelection(redBall, sysRedBall);
sysBlueBall=r.nextInt(16)+1;
for(int i=0;i<userRedBall.length;i++){
for(int j=0;j<sysRedBall.length-redCount;j++){
if(userRedBall[i]==sysRedBall[j]){
int temp=sysRedBall[j];
sysRedBall[j]=sysRedBall[sysRedBall.length-1-redCount];
sysRedBall[sysRedBall.length-1-redCount]=temp;
redCount++;
}
}
}
if(userBlueBall==sysBlueBall){
blueCount=1;
}
if(blueCount==0&&redCount<=3){
System.out.println("没中奖");
}else if(blueCount==1&&redCount<3){
System.out.println("六等奖");
}else if((blueCount==1&&redCount==3)||(blueCount==0&&redCount==4)){
System.out.println("五等奖");
}else if((blueCount==1&&redCount==4)||(blueCount==0&&redCount==5)){
System.out.println("四等奖");
}else if(blueCount==1&&redCount==5){
System.out.println("三等奖");
}else if(blueCount==0&&redCount==6){
System.out.println("二等奖");
}else if(blueCount==1&&redCount==6){
System.out.println("一等奖");
}else{
System.out.println("无效");
}
System.out.println("中奖红球号码:");
sort(sysRedBall);
System.out.println(Arrays.toString(sysRedBall)+"中奖篮球号码:"+sysBlueBall);
System.out.println("用户红球号码:");
sort(userRedBall);
System.out.println(Arrays.toString(userRedBall)+"中奖篮球号码:"+userBlueBall);
}
public static void sort(int[] ball){
for(int i=0;i<ball.length-1;i++){
for(int j=0;j<ball.length-1-i;j++){
if(ball[j]>ball[j+1]){
ball[j]=ball[j+1]+ball[j];
ball[j+1]=ball[j]-ball[j+1];
ball[j]=ball[j]-ball[j+1];
}
}
}
}
public static void comSelection(int[] ballCount, int[] redBall) {
int index = -1;
Random r = new Random();
for (int i = 0; i < redBall.length; i++) {
index = r.nextInt(ballCount.length - i);
redBall[i] = ballCount[index];
int temp = ballCount[index];
ballCount[index] = ballCount[ballCount.length - i - 1];
ballCount[ballCount.length - i - 1] = temp;
}
}
}