01.异常处理
- 基本概念:阻止当前方法或作用域继续执行的问题,在程序中导致程序中断运行的一些指令;
- 体系结构:
2.1 Throwable是异常的基类,分为Error和Exception,在编程里我们关注Exception;
2.2 Exception分为编译时异常(受检)和运行时异常(非受检);
2.3 异常会导致程序中断,无法继续执行;
2.4 在开发中,我们需要把可能出现异常的代码使用try语句块包裹起来;
2.5 处理异常可以让程序保持运行状态;
2.6 catch可以有多个,顺序为从子类到父类,大的放后面,小的放前面;
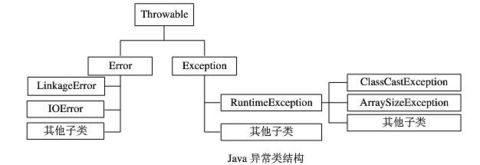
- 异常处理:try和catch关键字
public class Test {
public static void main(String[] args) {
div(10,2);
div(10,0);
}
private static void div (int num1,int num2) {
int[] arr = {1, 2, 3, 4, 5};
try {
System.out.println(arr[5]);
int result = num1/num2;
System.out.println(result);
} catch (ArithmeticException e) {
System.out.println("除数不能为0");
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("数组下标越界");
} catch (Exception e) {
System.out.println("出错了");
} finally {
System.out.println("程序执行完毕");
}
System.out.println("程序结束");
}
}
public class Test {
public static void main(String[] args) {
try {
div(10,0);
} catch (Exception e) {
e.printStackTrace();
}
}
private static void div (int num1,int num2) throws Exception {
try {
int result = num1/num2;
System.out.println(result);
} catch (ArithmeticException e) {
throw new Exception ("除数不能为0");
}
System.out.println("程序结束");
}
}
- 异常处理过程分析:
4.1 一旦产生异常,则系统会自动产生一个异常类的实例化对象;
4.2 如果存在try语句,则会自动找到匹配的catch语句执行;如果没有异常处理,则程序退出,并由系统报告错误;
4.3 所有的catch根据方法的参数匹配异常类的实例化对象,如果匹配成功,则由此catch进行处理;
- finally关键字:在进行异常的处理之后,在异常的处理格式中还有一个finally语句,那么此语句将作为异常的统一出口,不管是否产生了异常,最终都要执行此段代码;
- throw和throws关键字:
6.1 throws关键字主要在方法的声明上使用,表示方法中不处理异常,而交给调用处处理,实际上对于java程序来讲,如果没有加入任何的异常处理,默认由JVM进行异常的处理操作;
6.2 throw关键字:表示在程序中手动抛出一个异常,因为从异常处理机制来看,所有的异常一旦产生之后,实际上抛出的就是一个异常类的实例化对象,此对象也可以由throw直接抛出;
- 语法规则:
7.1 三种结构:try/catch/finally、try/catch、try/finally,try、catch、finally三个关键字均不能单独使用,catch语句可以有多个,finally语句最多一个;
7.2 try、catch、finally三个代码块中变量得作用域分别独立而不能相互访问;
7.3 多个catch块时,java虚拟机会匹配其中一个异常类或其子类,就执行这个catch块,而不会再执行别的catch块。
02.自定义异常
- 在java中,已经提供了很多的异常类的定义,但是我们在实际项目开发中,可能需要使用一些自己的异常类,那么可以通过继承Exception类或已有的异常类的方式完成一个自定义异常类的操作;
- 一般可以继承:Throwable、Exception、RuntimeException;
- 自定义异常类的是实现是,提供构造方法;
- 异常对象本身并没有实际功能,只是一个有意义的标识;
public class MyException extends Exception {
public MyException(){
super();
}
public MyException(String message){
super(message);
}
}
public class User {
private String username;
private String password;
private int age;
private String sex;
public User() {
super();
}
public User(String username, String password, int age, String sex) {
super();
this.username = username;
this.password = password;
this.age = age;
this.sex = sex;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
@Override
public String toString() {
return "User [username=" + username + ", password=" + password + ", age=" + age + ", sex=" + sex + "]";
}
}
public class UserService {
public User login(String username, String password) throws MyException {
if(!"admin".equals(username)){
throw new MyException("用户名错误");
}
if(!"12345".equals(password)){
throw new MyException("密码错误");
}
User user = new User("admin","12345",20,"男");
return user;
}
}
import java.util.Scanner;
public class UserTest {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("用户名:");
String name = input.nextLine();
System.out.println("密码:");
String password = input.nextLine();
UserService us = new UserService();
try {
User user = us.login(name, password);
System.out.println("登录成功");
System.out.println(user.toString());
} catch (MyException e) {
e.printStackTrace();
}
}
}
03.受检与非受检异常
- 受检异常(Exception):定义方法时必须声明所有可能会抛出的exception,在调用这个方法时,必须捕获它的checked exception,不然就得把它的exception传递下去;
- 非受检异常(RuntimeException):在定义方法时不需要声明会抛出runtime exception,在调用这个方法时不需要捕获这个runtime exception;
- assert关键字:表示断言,当程序执行到某个固定位置的时候,程序中的某个变量的取值肯定是预期的结果,那么这种操作可以使用断言完成;
public class AssertTest {
public static void main(String[] args) {
int result = add(10,10);
assert result==10:"结果不正确";
}
private static int add(int a,int b){
return a+b+1;
}
}