tf.split
tf.split(value, num_or_size_splits, axis=0, num=None, name='split')
将tensor拆分为新的tensor
如果'num_or_size_splits'是一个整数'num_split',就把'value'沿着'axis'所在的维拆分成'num_split'个更小的tensor。
如果'num_or_size_splits'不是一个整数,那就假定它是个tensor 'size_splits',然后将‘value’拆分为‘len(size_splits)’块。
第i块的形状和‘value’的形状相同,除了‘axis’维的形状是‘size_splits[i]’。
A=[[[1,2],[3,4],[5,6]],[[7,8],[9,0],[0,1]]]
np.array(A).shape
Output: (2, 3, 2)
x1=tf.split(A,2,0)
x2=tf.split(A,3,1)
x3=tf.split(A,[2,1],1)
with tf.Session() as sess:
c1=sess.run(x1)
c2=sess.run(x2)
print(c1,np.array(c1).shape)
print(c2,np.array(c2).shape)
Output:
[array([[[1, 2],
[3, 4],
[5, 6]]], dtype=int32),
array([[[7, 8],
[9, 0],
[0, 1]]], dtype=int32)] (2, 1, 3, 2)
[array([[[1, 2]],
[[7, 8]]], dtype=int32),
array([[[3, 4]],
[[9, 0]]], dtype=int32),
array([[[5, 6]],
[[0, 1]]], dtype=int32)] (3, 2, 1, 2)
with tf.Session() as sess:
c=sess.run(x3)
print(c,np.array(c).shape)
Output:
[array([[[1, 2],
[3, 4]],
[[7, 8],
[9, 0]]], dtype=int32),
array([[[5, 6]],
[[0, 1]]], dtype=int32)]
对比:tf.unstack
tf.unstack(value, num=None, axis=0, name='unstack')
Unpacks the given dimension of a rank-`R` tensor into rank-`(R-1)` tensors.
将本来维数为R的tensor解压到维数为R-1
例如,给定一个形状为(A,B,C,D)的tensor。如果axis==0,输出是个列表,在输出中的第i个tensor是'value[i,:,:,:]',每个tensor的形状是'B,C,D'。注意这里和tf.split的不同,tf.unstack被解压的那一维在输出中没有了,输出的每个tensor的形状是(B,C,D),而tf.split按axis=0拆分后得到的tensor的形状是(1,B,C,D),可以再用tf.squeeze将形状为1的维去除。但tf.split更灵活,可以设置将某个维拆成几部分,或者设置拆分后该维每个部分的形状,例如设置num_or_size_splits=[2,3,5]等。
tf.squeeze
tf.squeeze(input, axis=None, name=None, squeeze_dims=None)
从tensor中去除形状为1的维。(为了去除多余的维)
例如,t是一个形状为[1,2,1,3,1,1]的张量,则tf.squeeze(t)的形状为[2,3]
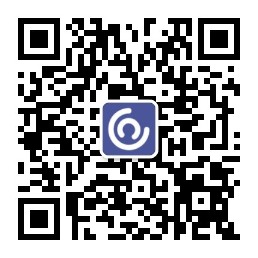
接tf.split的例子,tf.split(A,2,0)生成了2个形状为(1,3,2)的tensor,分别为
[[[1,2],[3,4],[5,6]]] 和[[[7,8],[9,0],[0,1]]],使用squeeze处理之后,2个tensor的形状变成(3,2)
x1=tf.split(A,2,0)[0]
with tf.Session() as sess:
c=sess.run(x1)
print(c)
print(np.array(c).shape)
Output:
[[[1 2]
[3 4]
[5 6]]]
(1, 3, 2)
x2=tf.squeeze(x1)
with tf.Session() as sess:
c=sess.run(x2)
print(c)
print(np.array(c).shape)
Output:
[[1 2]
[3 4]
[5 6]]
(3, 2)
tf.stop_gradient()
阻止节点BP的梯度
tf.argmax()
tf.argmax(input, axis=None, name=None, dimension=None, output_type=tf.int64)
Returns the index with the largest value across axes of a tensor. (deprecated arguments)
返回tensor某个维的最大值的索引位置。
在神经网络中,对全连接层的输出进行softmax得到"logits",通常使用tf.argmax(logits,1)来输出类别预测值。
tf.concat()
tf.concat(values, axis, name='concat')
Concatenates tensors along one dimension.
沿着某维串接tensors。和pandas包的concat方法差不多
例如,有tensor1 "t1"形状为[2,3],tensor2 "t2"形状为[2,3],则
tf.concat([t1,t2],0)的形状为[4,3],tf.concat([t1,t2],1)的形状为[2,6]
a=tf.Variable([[1,2,3],[4,5,6]])
b=tf.Variable([[7,8,9],[10,11,12]])
c=tf.concat([a,b],0)
d=tf.concat([a,b],1)
with tf.Session() as sess:
tf.global_variables_initializer().run()
cx=sess.run(c)
dx=sess.run(d)
print(cx)
print(dx)
Output:
[[ 1 2 3]
[ 4 5 6]
[ 7 8 9]
[10 11 12]]
[[ 1 2 3 7 8 9]
[ 4 5 6 10 11 12]]
对比:tf.stack
tf.stack(values, axis=0, name='stack')
Stacks a list of rank-`R` tensors into one rank-`(R+1)` tensor.
将一个维数为R的tensor列表压缩为维数为R+1的tensor
例如:给定长度为N的tensor列表,每个tensor的形状是(A,B,C)
如果axis==0,则tf.stack输出的tensor形状是'(N,A,B,C)'
如果axis==1,则tf.stack输出的tensor形状是'(A,N,B,C)'
对比tf.concat,tf.concat输出的tensor形状不会增加一维。也就是如果N个形状为(A,B,C)的tensor按axis=0进行tf.concat操作,则输出的tensor的形状是'(N*A,B,C)'
tf.reshape()
tf.reshape(tensor, shape, name=None)
Reshapes a tensor.
重构tensor的形状
给定一个tensor,这个运算返回一个值相同,但形状为“shape”参数规定形状的tensor
如果“shape”参数里面有个值是-1,表示这个维的size是被计算出来的,从而保持总的size不变。如果“shape”参数是“[-1]”,那就是把这个tensor铺平为1维的。大多数情况下,“shape”参数中会有一个-1。
如果"shape"是1维的或更高维的,那这个运算返回一个tensor,形状为"shape"参数值,值为“tensor”的值。在这种情况下,"shape"参数隐含的元素数量("shape"参数所有维的size的乘积)需要和"tensor"中的元素数量相同。
例如:
# tensor 't' is [1, 2, 3, 4, 5, 6, 7, 8, 9]
# tensor 't' has shape [9]
reshape(t, [3, 3]) ==> [[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
# tensor 't' is [[[1, 1], [2, 2]],
# [[3, 3], [4, 4]]]
# tensor 't' has shape [2, 2, 2]
reshape(t, [2, 4]) ==> [[1, 1, 2, 2],
[3, 3, 4, 4]]
# tensor 't' is [[[1, 1, 1],
# [2, 2, 2]],
# [[3, 3, 3],
# [4, 4, 4]],
# [[5, 5, 5],
# [6, 6, 6]]]
# tensor 't' has shape [3, 2, 3]
# pass '[-1]' to flatten 't'
reshape(t, [-1]) ==> [1, 1, 1, 2, 2, 2, 3, 3, 3, 4, 4, 4, 5, 5, 5, 6, 6, 6]
# -1 can also be used to infer the shape
# -1 is inferred to be 9:
reshape(t, [2, -1]) ==> [[1, 1, 1, 2, 2, 2, 3, 3, 3],
[4, 4, 4, 5, 5, 5, 6, 6, 6]]
# -1 is inferred to be 2:
reshape(t, [-1, 9]) ==> [[1, 1, 1, 2, 2, 2, 3, 3, 3],
[4, 4, 4, 5, 5, 5, 6, 6, 6]]
# -1 is inferred to be 3:
reshape(t, [ 2, -1, 3]) ==> [[[1, 1, 1],
[2, 2, 2],
[3, 3, 3]],
[[4, 4, 4],
[5, 5, 5],
[6, 6, 6]]]
tf.get_variable()
当需要共享变量时,使用tf.get_variable(),因为当“name”参数冲突时,会报错。而tf.Variable()遇到冲突的“name”,会自己解决冲突(重命名)