1.SpringBoot访问静态资源(有两种方法)
1.1SpringBoot 从 classpath/static 的目录访问(src/main/resources),注意目录名称必须是 static
如图所示:
注意:在src/main/resources下建立static可能会报失败说是无效的包名,此时不是建立包package而是建立一个floder
1.1.1编写启动器
@SpringBootApplication
public class App {
public static void main(String[] args) {
SpringApplication.run(App.class,args);
}
}
启动后直接访问index.html即可,或者是images/java.jpg也可以
1.2ServletContext 根目录下,在 src/main/webapp 目录名称必须要是webapp
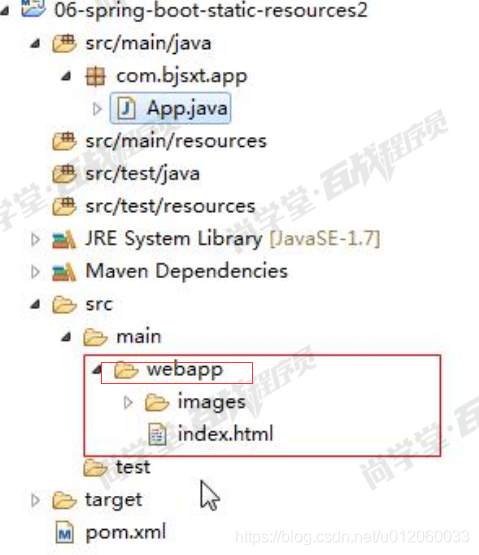
1.2.1启动器编写后直接访问即可
@SpringBootApplication
public class App {
public static void main(String[] args) {
SpringApplication.run(App.class, args);
}
}
2.SpringBoot文件上传
2.1编写 Controller
//表示该类下的方法的返回值会自动做json格式的转换
@RestController
public class FileUpload {
@RequestMapping("/fileUpload")
public Map<String,Object> fileUpload(@RequestParam("filename") MultipartFile file)
throws IllegalStateException, IOException{
System.out.println(file.getOriginalFilename());
Map<String,Object> map = new HashMap<String,Object>();
file.transferTo(new File("e:/"+file.getOriginalFilename()));
map.put("msg","ok");
return map;
}
}
注意:此处的@RequestParam这个注解,是为了应对从前台传过来的参数名字和自己命名的形参不一致所需要的
2.2编写启动类
@SpringBootApplication
public class App {
public static void main(String[] args) {
SpringApplication.run(App.class, args);
}
}
2.3编写页面在src/main/resources下的static文件夹
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="fileUpload" method="post" enctype="multipart/form-data">
上传文件:<input type="file" name="filename"/><br/>
<input type="submit" value="上传"/>
</form>
</body>
</html>
2.4设置上传文件大小的默认值
需要添加一个 springBoot 的配置文件application.properties,名字只能是application.properties
#设置单个上传文件的大小
spring.http.multipart.maxFileSize=200MB
#设置一次请求上传文件的总容量
spring.http.multipart.maxRequestSize=200MB