静态效果图如下,时钟运行起来可以走动
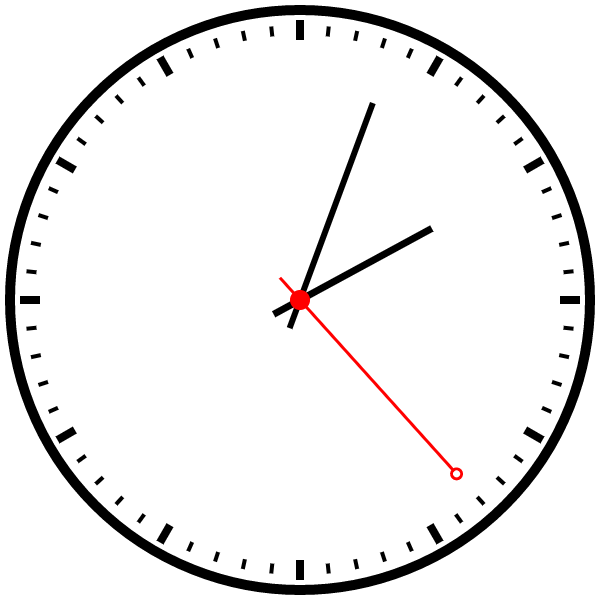
HTML代码如下
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>时钟</title>
<link href="style.css" type="text/css" rel="stylesheet"/>
<script type="text/javascript" src="script.js"></script>
</head>
<body onload="main()">
<canvas id="canvas" height=600 width=600></canvas>
</body>
</html>
CSS代码如下
canvas{
margin: 0 auto;
display: block;
}
JavaScript代码如下
var canvas = null
var context = null
function main()
{
canvas = document.getElementById("canvas")
context = canvas.getContext("2d")
window.setInterval(function ()
{
var date = new Date()
var hour = date.getHours()>12 ? date.getHours()-12 : date.getHours()
var minute = date.getMinutes()
var second = date.getSeconds()
context.clearRect(0,0,600,600)
clockBorder()
graduate()
minutePointer(minute,second)
hourPointer(hour,minute)
secondPointer(second)
},1000)
}
//表盘轮廓
function clockBorder()
{
context.beginPath()
context.strokeStyle = "black"
context.lineWidth = 10
context.arc(300,300,290,0,2*Math.PI,true)
context.stroke()
context.closePath()
}
//刻度
function graduate()
{
for (var i=0
{
context.beginPath()
context.lineWidth = 8
context.save()
context.translate(300, 300)
context.rotate(i*30*Math.PI/180)
context.moveTo(0,-280)
context.lineTo(0,-260)
context.stroke()
context.restore()
context.closePath()
}
for (var i=0
{
context.beginPath()
context.lineWidth = 4
context.save()
context.translate(300, 300)
context.rotate(i*6*Math.PI/180)
context.moveTo(0,-275)
context.lineTo(0,-265)
context.stroke()
context.restore()
context.closePath()
}
}
function hourPointer(hour, minute)
{
context.beginPath()
context.save()
context.lineWidth = 7
context.translate(300, 300)
context.rotate(hour*30*Math.PI/180 + minute/60*30*Math.PI/180)
context.moveTo(0, 30)
context.lineTo(0,-150)
context.stroke()
context.restore()
context.closePath()
}
function minutePointer(minute,second)
{
context.beginPath()
context.save()
context.lineWidth = 6
context.translate(300, 300)
context.rotate(minute*6*Math.PI/180 + second/60*6*Math.PI/180)
context.moveTo(0, 30)
context.lineTo(0,-210)
context.stroke()
context.restore()
context.closePath()
}
function secondPointer(second)
{
context.lineWidth = 3
context.strokeStyle = "red"
context.beginPath()
context.save()
context.translate(300, 300)
context.rotate(second*6*Math.PI/180)
context.moveTo(0, 30)
context.lineTo(0,-230)
context.stroke()
context.closePath()
context.beginPath()
context.arc(0,0,10,0,2*Math.PI,true)
context.fillStyle = "red"
context.fill()
context.closePath()
context.beginPath()
context.arc(0,-234,5,0,2*Math.PI,true)
context.lineWidth = 3
context.stroke()
context.closePath()
context.restore()
}