版权声明:欢迎转载,转载请注明出处-->http://blog.csdn.net/angcyo https://blog.csdn.net/angcyo/article/details/84952176
一 类型映射
Java type | Kotlin type |
---|---|
byte | kotlin.Byte |
short | kotlin.Short |
int | kotlin.Int |
long | kotlin.Long |
char | kotlin.Char |
float | kotlin.Float |
double | kotlin.Double |
boolean | kotlin.Boolean |
二 基础语法
变量声明
//java 声明变量, 类型在 左边, 变量名在右边;
int a;
String b;
final float c;
//kotlin 声明变量,可以显示的指定类型, 也可以智能判断类型.
//;号是可选的
val a = 0; //val 赋值之后, 不可再次赋值
var b:String; //var 可以多次赋值
const val c:Float=0f; //const 声明的变量必须初始化
const
必须在
class Test {
companion object {
//此对象中声明的所有变量和方法, 都是静态的
const val c:Float=0f;
}
}
或
object Test {
//此对象中声明的所有变量和方法, 都是静态的
const val c:Float=0f;
}
中使用
函数声明
//java
public void test(int a, String b){
return;
}
private String test2(int a, String b){
return "";
}
kotlin
默认是public
的访问权限.
fun test(a:Int, b:String){
return;
}
private fun test2(a:Int, b:String):String{
return "";
}
静态方法
public static void test3(){
}
//1:使用 `companion object`
class Test {
companion object {
fun test3() {
}
}
}
object Test {
fun test3() {
}
}
接口和类
//定义接口, 接口默认是 open 修饰. 意思就是 可被继承.
interface MyInterface {
fun bar()
fun foo() {
// 可选的方法体
}
}
//实现接口, class 默认是不可以 被继承的. 如需继承, 需要使用 open 修饰
class Child : MyInterface {
override fun bar() {
// 方法体
}
}
//比如:
open class Child : MyInterface {
override fun bar() {
// 方法体
}
}
类的构造函数
//constructor 关键字 是可选的
class Person constructor(firstName: String) { ... }
class Person(firstName: String) { ... }
class InitOrderDemo(name: String) {
val firstProperty = "First property: $name".also(::println)
//可以有多个init模块, 会按顺序执行
init {
println("First initializer block that prints ${name}")
}
val secondProperty = "Second property: ${name.length}".also(::println)
init {
println("Second initializer block that prints ${name.length}")
}
}
//使用val/var 修饰过的变量, 会自动变成 类的成员变量.否则此变量只在初始化的时候可以使用.
class Person(val firstName: String, val lastName: String, var age: Int) { …… }
//也可以使用这种方式构造类
class Person {
constructor(parent: Person) {
parent.children.add(this)
}
}
class Person(val name: String) {
constructor(name: String, parent: Person) : this(name) {
parent.children.add(this)
}
}
class DontCreateMe private constructor () { ... }
类的继承
//允许被继承的类, 需要使用 open 修饰.
open class Base(p: Int)
class Derived(p: Int) : Base(p)
//
class MyView : View {
constructor(ctx: Context) : super(ctx)
constructor(ctx: Context, attrs: AttributeSet) : super(ctx, attrs)
}
//允许被重写的 fun, 也需要使用 open 修饰, 否则不可以被 override
open class Base {
open fun v() { ... }
fun nv() { ... }
}
class Derived() : Base() {
override fun v() { ... }
}
三 扩展特性
可以对任意类
进行扩展, 其实就是对应java
中的静态方法.
只是省略了第一个参数为this
的传递
//比如:
//java中
public static String int2Str(int i){
return "test"
}
//调用方式: int2Str(888)
//kotlin 扩展
fun Int.int2Str():String{
return "test"
}
//调用方式: 888.int2Str()
fun MutableList<Int>.swap(index1: Int, index2: Int) {
val tmp = this[index1] // “this”对应该列表
this[index1] = this[index2]
this[index2] = tmp
}
有了扩展, kotlin
才变得好玩.
//你就可以这么玩
class Test{
var a = ""
var b : String
var c = 0
}
Test().apply{
a = "a"
b = "b"
c = 2
}
Test().let{
it.a = "a"
it.b = "b"
it.c = 2
}
//上述中的 apply , let 就是扩展方法. 还有很多好玩的扩展方法需要你们去发现.
扫描二维码关注公众号,回复:
5629353 查看本文章
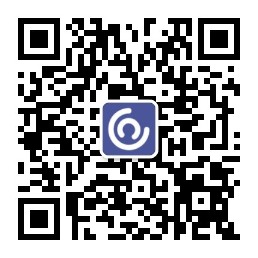
四 表达式
if表达式
//if语句支持返回值, 所以你可以用变量接收这个返回值.
fun maxOf(a: Int, b: Int): Int {
if (a > b) {
return a
} else {
return b
}
}
whenb表达式
//同上
fun describe(obj: Any): String =
when (obj) {
1 -> "One"
"Hello" -> "Greeting"
is Long -> "Long"
!is String -> "Not a string"
else -> "Unknown"
}
关于 ? !! 空安全
使用?
声明的类型, 即表示支持空安全检查.
var a:String = ""
a=null //编译错误, a不允许接收 null 类型.
var b:String? = ""
b=null //ok
val l = b.length //可能触发 NPE 异常.因为 b 允许为null
//改进写法
val l = b?.length ?: 0 //当b为null时, 返回0, 否则返回b.length
//?在这里, 代表需要进行非空检查.
//如果为空, 则返回null, 否则返回原对象.
//如果能够明确对象不为空, 那么就可以使用哦 !!,放弃非空检查
//即
val l=b!!.length //这种写法要确保b不为空, 否则NPE异常.
群内有各(pian)种(ni)各(jin)样(qun)的大佬,等你来撩.
联系作者
请使用QQ扫码加群, 小伙伴们都在等着你哦!
关注我的公众号, 每天都能一起玩耍哦!