Java集合框架(常用类) JCF
为了实现某一目的或功能而预先设计好一系列封装好的具有继承关系或实现关系类的接口;
集合的由来:
特点:元素类型可以不同,集合长度可变,空间不固定;
管理集合类和接口; list ,set, map 3大类
collection:是所有集合中心的接口(装东西的中心)
collections:是操作集合的算法类
collection(三大阵营):
List:ArrayList, LinkedList, Vector(legacy) (面试),
Set:HashSet,
扫描二维码关注公众号,回复:
5570056 查看本文章
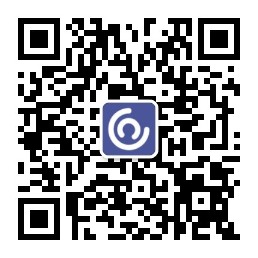
Map:HashMap,Hashtahle(面试),properties,
collection接口和Iterator接口:
集合框架中,分为两种API:
程序例:
1:List容器
public static void main(String[] args) {
// TODO Auto-generated method stub
//List---列表。
//List特点:线性。即:有序,元素的放入顺序和元素的存储顺序保持一致。
// 表象上,List最大的特点就是有下标。
//ArrayList就是作为一个数组的封装出现的,底层就是数组
//LinkedList底层封装的是一个双向链表。
//当需要做大量的查询动作的时候,使用ArrayList;
//当需要做大量的增加删除动作(特别是往中间增删),使用LinkedList
//面试中:ArrayList常常和Vector比较
//泛型---用来控制集合只能操作某一种数据类型
LinkedList<StudentBean> lst = new LinkedList<StudentBean>();
//增
lst.add(new StudentBean("zhang3",44,90));
lst.add(new StudentBean("zhao4",32,76));
lst.add(new StudentBean("lidaye",55,62));
lst.add(new StudentBean("zhoudama",60,84));
//求长度
int size = lst.size();
//修改
lst.set(0, new StudentBean("zhang3feng",102,45));
//删除
lst.remove(0);
//获取某个元素
// StudentBean stu = (StudentBean)lst.get(1);
StudentBean stu = lst.get(1);//加上泛型不需强转
//遍历---将集合中的每个元素依次取出,做同样的操作
//1、使用普通for循环进行遍历
for(int i = 0; i < lst.size(); i++){
StudentBean tmpStu = lst.get(i);
System.out.println(tmpStu.getName());
}
//2、使用迭代器---Iterator完成遍历----是集合框架类Collection直接分支专用
// 特点是没有下标,从头到尾走一遍
Iterator<StudentBean> it = lst.iterator();
while(it.hasNext()){
StudentBean tmpStu = it.next();
System.out.println(tmpStu.getName());
}
//3、for-each循环:底层封装的就是迭代器,但语法更简单,还可以操作数组
for(StudentBean tmpStu : lst){
System.out.println(tmpStu.getName());
}
//for-each还可以操作数组
int[] array = {12,13,14,15,16,17};
for(int tmp : array){
tmp ++;
System.out.println(tmp);
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
//Map---映射
//Map的特点:键值对。键要求唯一,值可以重复
// 放入元素的顺序和存储顺序无关
//常用子类:HashMap(主要用于集合操作)、Properteis(专用于操作属性文件)
//面试中:HashMap常常和Hashtable进行比较
HashMap<String, StudentBean> map = new HashMap<String, StudentBean>();
//增加元素
map.put("j34001", new StudentBean("zhang3",18,80));
map.put("j34002", new StudentBean("li4",28,85));
map.put("j34003", new StudentBean("wang5",18,87));
//得到长度
System.out.println(map.size());
//修改元素
map.put("j34003", new StudentBean("zhao6",24,75));
//删除元素---通过键去移除元素
map.remove("j34003");
//获取指定的元素对象
StudentBean stu = map.get("j34003");
//遍历Map
//不能同时遍历键和值;只能分开遍历
//遍历所有的键
Set<String> keySet = map.keySet();//得到所有的键,装入一个Set集合中,返回给调用者
for(String key : keySet){
System.out.println(key);
}
//遍历所有的值
Collection<StudentBean> allStus = map.values();//得到所有的值,思考为什么不返回List或其它集合类型?
for(StudentBean tmpStu : allStus){
System.out.println(tmpStu.getName());
}
}
public static void main(String[] args) {
//Set---集
//Set的特点---不能放置重复元素、无序。
// 表象上,Set没有下标
//常用子类HashSet
//HashSet如何判断两个元素是否重复呢?
//1、调用equals方法得到两个对象比较为true
//2、两个元素的hashcode值保持一致
//只有这两个条件同时满足,Java才认为这是同一个对象
//所以重写了equals方法一般也要重写hashcode方法,让equals返回true的对象
//hashcode也能返回同样的整数值
HashSet set = new HashSet();
//放入元素
set.add("hello");
set.add(new Date());
StudentBean stu0 = new StudentBean("zhang3",18,80);
set.add(stu0);
set.add(200);
StudentBean stu1 = new StudentBean("zhang3",18,80);
set.add(stu1);
//放入元素的个数
System.out.println(set.size());
System.out.println(stu0.equals(stu1));
System.out.println(stu0.hashCode());
System.out.println(stu1.hashCode());
//修改---没有修改方法
//删除---只能根据对象进行删除,还是用的equals和hashCode来判断到底删除哪个对象
set.remove(new StudentBean("zhang3",18,80));
//获取某个元素---无法获取某个指定的元素
//遍历---将集合中的每个元素依次取出,做同样的操作
//1、不支持普通for循环,没有下标
//2、支持迭代器
Iterator it = set.iterator();
while(it.hasNext()){
Object obj = it.next();
System.out.println(obj);
}
//3、支持for-each循环
for(Object obj : set){
System.out.println(obj);
}
}
public class StudentBean implements Comparable<StudentBean>{
private String name;
private int age;
private int score;
public StudentBean(){
}
public StudentBean(String name, int age, int score) {
super();
this.name = name;
this.age = age;
this.score = score;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
@Override
public boolean equals(Object obj) {
// TODO Auto-generated method stub
if(obj instanceof StudentBean){
StudentBean stu = (StudentBean)obj;
if(this.name.equals(stu.getName()) && this.age == stu.getAge() &&
this.score == stu.getScore()){
return true;
}
}
return false;
}
@Override
public int hashCode() {
// TODO Auto-generated method stub
int num = 0;
if(this.name != null){
char[] array = this.name.toCharArray();
for(int a : array){
num += a;
}
}
num += this.age;
num += this.score;
return num;
}
@Override
public int compareTo(StudentBean stu) {
// TODO Auto-generated method stub
//返回的正数、负数,依赖于根据比较规则两个元素的位置之差
// if(this.age < stu.getAge()){
// return -1;
// }else if(this.age > stu.getAge()){
// return 1;
// }else{
// return 0;
// }
if(this.score > stu.getScore()){
return -1;
}else if(this.score < stu.getScore()){
return 1;
}else{
if(this.age > stu.getAge()){
return 1;
}else if(this.age < stu.getAge()){
return -1;
}else{
return 0;
}
}
}
@Override
public String toString() {
// TODO Auto-generated method stub
return this.name + " " + this.age + " " + this.score;
}
}
public class IntegerComparator implements Comparator<Integer>{
@Override
public int compare(Integer o1, Integer o2) {
// TODO Auto-generated method stub
if(o1 > o2){
return -1;
}else if(o1 < o2){
return 1;
}
return 0;
}
}
public class StudentComparator implements Comparator<StudentBean>{
@Override
public int compare(StudentBean o1, StudentBean o2) {
// TODO Auto-generated method stub
if(o1.getScore() > o2.getScore()){
return -1;
}else if(o1.getScore() < o2.getScore()){
return 1;
}
return 0;
}
}