版权声明:访问者可将本博客提供的内容或服务用于个人学习、研究或欣赏,以及其他非商业性或非盈利性用途,但同时应遵守著作权法及其他相关法律的规定,不得侵犯本网站及相关权利人的合法权利。除此以外,将本网站任何内容或服务用于其他用途时,须及时征得本网站及相关权利人的明确许可。 https://blog.csdn.net/qq_38262728/article/details/88545091
《机器学习实战——基于Scikit-Learn和TensorFlow》
这是一本非常好的机器学习和深度学习入门书,既有基本理论讲解,也有实战代码示例。
我将认真阅读此书,并为每一章内容做一个知识笔记。
我会摘录一些原书中的关键语句和代码,若有错误请为我指出。
目录
第九章 运行TensorFlow
TensorFlow的原理:首先在python中定义一个用来计算的图,然后TensorFlow就会使用这个图,并用优化过的c++代码来执行计算。
最重要的是,TensorFlow可以将一个计算图划分成多个子图,然后并行地在多个CPU或GPU上执行。
支持分布式计算。
可以在数十亿个实例组成的训练集上训练具有数百万个参数的神经网络,每个实例具有数百万个特征。
亮点:
- 可运行在Windows、Linux、macOS和移动设备上,包括iOS和Android。
- 提供一个TF.Learn的API兼容scikit-learn。
- 提供一个TF-Slim的API来简化神经网络的构建、训练和评估。
- 在TensorFlow基础上独立构建了一些高级的API,如Keras,prettyTensor。
- API提供了很多灵活的方式来创建所有类型的计算。
- 包含很多高效实用的C++实现的机器学习操作,通过API可以用C++来实现自己的高性能操作。
- 搜索最小化成本函数的参数提供了很多优化的节点。
- 提供了Tensorboard可视化工具来浏览计算图,查看学习曲线。
- 云服务。
- 开发团队、社区。
1 安装
pip3 install tensorflow
pip3 install tensorflow-gpu
2 创建一个计算图并在会话中执行
import tensorflow as tf
reset_graph()
x = tf.Variable(3, name="x")
y = tf.Variable(4, name="y")
f = x*x*y + y + 2
with tf.Session() as sess:
x.initializer.run()
y.initializer.run()
result = f.eval()
或
init = tf.global_variables_initializer()
with tf.Session() as sess:
init.run()
result = f.eval()
一个TensorFlow程序的两部分:构建阶段,构建一个计算图;执行阶段,执行这个图。
3 管理图
创建的所有节点会自动添加到默认图上。
扫描二维码关注公众号,回复:
5551680 查看本文章
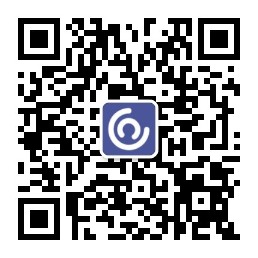
避免混乱可以:
graph = tf.Graph()
with graph.as_default():
x2 = tf.Variable(2)
x2.graph is graph
4 节点值的生命周期
当求解一个节点时,TensorFlow会自动检测该节点依赖的节点,并先对这些节点求值。
TensorFlow不会复用上一步求值的结果,会重新开始求值。
或者在一个执行中就同时完成多个求解操作,例:
with tf.Session() as sess:
y_val, z_val = sess.run([y, z])
print(y_val) # 10
print(z_val) # 15
在图的每次执行间,所有节点值都会被丢弃,但是变量的值不会。变量的生命周期从初始化器的执行开始,到关闭会话才结束。
5 TensorFlow中的线性回归
import numpy as np
from sklearn.datasets import fetch_california_housing
reset_graph()
housing = fetch_california_housing()
m, n = housing.data.shape
housing_data_plus_bias = np.c_[np.ones((m, 1)), housing.data]
X = tf.constant(housing_data_plus_bias, dtype=tf.float32, name="X")
y = tf.constant(housing.target.reshape(-1, 1), dtype=tf.float32, name="y")
XT = tf.transpose(X)
theta = tf.matmul(tf.matmul(tf.matrix_inverse(tf.matmul(XT, X)), XT), y)
with tf.Session() as sess:
theta_value = theta.eval()
6 实现梯度下降
当使用梯度下降法时,先对输入的特征向量做归一化,否则训练过程非常慢。
6.1 手工计算梯度
reset_graph()
n_epochs = 1000
learning_rate = 0.01
X = tf.constant(scaled_housing_data_plus_bias, dtype=tf.float32, name="X")
y = tf.constant(housing.target.reshape(-1, 1), dtype=tf.float32, name="y")
theta = tf.Variable(tf.random_uniform([n + 1, 1], -1.0, 1.0, seed=42), name="theta")
y_pred = tf.matmul(X, theta, name="predictions")
error = y_pred - y
mse = tf.reduce_mean(tf.square(error), name="mse")
gradients = 2/m * tf.matmul(tf.transpose(X), error)
training_op = tf.assign(theta, theta - learning_rate * gradients)
init = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init)
for epoch in range(n_epochs):
if epoch % 100 == 0:
print("Epoch", epoch, "MSE =", mse.eval())
sess.run(training_op)
best_theta = theta.eval()
6.2 使用自动微分
reset_graph()
n_epochs = 1000
learning_rate = 0.01
X = tf.constant(scaled_housing_data_plus_bias, dtype=tf.float32, name="X")
y = tf.constant(housing.target.reshape(-1, 1), dtype=tf.float32, name="y")
theta = tf.Variable(tf.random_uniform([n + 1, 1], -1.0, 1.0, seed=42), name="theta")
y_pred = tf.matmul(X, theta, name="predictions")
error = y_pred - y
mse = tf.reduce_mean(tf.square(error), name="mse")
gradients = tf.gradients(mse, [theta])[0]
training_op = tf.assign(theta, theta - learning_rate * gradients)
init = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init)
for epoch in range(n_epochs):
if epoch % 100 == 0:
print("Epoch", epoch, "MSE =", mse.eval())
sess.run(training_op)
best_theta = theta.eval()
print("Best theta:")
print(best_theta)
6.3 使用优化器
optimizer = tf.train.GradientDescentOptimizer(learning_rate=learning_rate)
training_op = optimizer.minimize(mse)
7 给训练算法提供数据
批量提供:
def fetch_batch(epoch, batch_index, batch_size):
np.random.seed(epoch * n_batches + batch_index) # not shown in the book
indices = np.random.randint(m, size=batch_size) # not shown
X_batch = scaled_housing_data_plus_bias[indices] # not shown
y_batch = housing.target.reshape(-1, 1)[indices] # not shown
return X_batch, y_batch
with tf.Session() as sess:
sess.run(init)
for epoch in range(n_epochs):
for batch_index in range(n_batches):
X_batch, y_batch = fetch_batch(epoch, batch_index, batch_size)
sess.run(training_op, feed_dict={X: X_batch, y: y_batch})
best_theta = theta.eval()
8 保存和恢复模型
可以在训练过程中定期将检查点保存起来,这样当电脑崩溃时,可以从最近一个检查点恢复,而不是从头再来。
reset_graph()
n_epochs = 1000 # not shown in the book
learning_rate = 0.01 # not shown
X = tf.constant(scaled_housing_data_plus_bias, dtype=tf.float32, name="X") # not shown
y = tf.constant(housing.target.reshape(-1, 1), dtype=tf.float32, name="y") # not shown
theta = tf.Variable(tf.random_uniform([n + 1, 1], -1.0, 1.0, seed=42), name="theta")
y_pred = tf.matmul(X, theta, name="predictions") # not shown
error = y_pred - y # not shown
mse = tf.reduce_mean(tf.square(error), name="mse") # not shown
optimizer = tf.train.GradientDescentOptimizer(learning_rate=learning_rate) # not shown
training_op = optimizer.minimize(mse) # not shown
init = tf.global_variables_initializer()
saver = tf.train.Saver()
with tf.Session() as sess:
sess.run(init)
for epoch in range(n_epochs):
if epoch % 100 == 0:
print("Epoch", epoch, "MSE =", mse.eval()) # not shown
save_path = saver.save(sess, "/tmp/my_model.ckpt")
sess.run(training_op)
best_theta = theta.eval()
save_path = saver.save(sess, "/tmp/my_model_final.ckpt")
9 用TensorBoard来可视化图和训练曲线
with tf.Session() as sess: # not shown in the book
sess.run(init) # not shown
for epoch in range(n_epochs): # not shown
for batch_index in range(n_batches):
X_batch, y_batch = fetch_batch(epoch, batch_index, batch_size)
if batch_index % 10 == 0:
summary_str = mse_summary.eval(feed_dict={X: X_batch, y: y_batch})
step = epoch * n_batches + batch_index
file_writer.add_summary(summary_str, step)
sess.run(training_op, feed_dict={X: X_batch, y: y_batch})
best_theta = theta.eval() # not shown
10 命名作用域
创建命名作用域来将相关的节点分组。
with tf.name_scope("loss") as scope:
error = y_pred - y
mse = tf.reduce_mean(tf.square(error), name="mse")
11 模块化
reset_graph()
def relu(X):
w_shape = (int(X.get_shape()[1]), 1)
w = tf.Variable(tf.random_normal(w_shape), name="weights")
b = tf.Variable(0.0, name="bias")
z = tf.add(tf.matmul(X, w), b, name="z")
return tf.maximum(z, 0., name="relu")
n_features = 3
X = tf.placeholder(tf.float32, shape=(None, n_features), name="X")
relus = [relu(X) for i in range(5)]
output = tf.add_n(relus, name="output")