题目:最小栈
题目描述:设计一个支持 push,pop,top 操作,并能在常数时间内检索到最小元素的栈。
push(x) – 将元素 x 推入栈中。
pop() – 删除栈顶的元素。
top() – 获取栈顶元素。
getMin() – 检索栈中的最小元素。
示例:
MinStack minStack = new MinStack();
minStack.push(-2);
minStack.push(0);
minStack.push(-3);
minStack.getMin(); --> 返回 -3.
minStack.pop();
minStack.top(); --> 返回 0.
minStack.getMin(); --> 返回 -2.
分析:想法就是两个模拟栈,一个存放元素,另一个存放当前的最小值,需要注意,当你插入一个元素时,也要判断最小栈的栈顶与新加入元素的比较,还有就是,弹出元素时,更新最小栈,最后就是使用切片代替栈的一些常用方法
代码:
type MinStack struct {
Values []int
Min []int
}
/** initialize your data structure here. */
func Constructor() MinStack {
return MinStack{Values:make([]int,0),Min:make([]int,0)}
}
func (this *MinStack) Push(x int) {
this.Values=append(this.Values,x)
if len(this.Min)==0 || x<=this.GetMin(){
this.Min=append(this.Min,x)
}
}
func (this *MinStack) Pop() {
if this.Top()==this.GetMin(){
this.Min=this.Min[:len(this.Min)-1]
}
this.Values=this.Values[:len(this.Values)-1]
}
func (this *MinStack) Top() int {
return this.Values[len(this.Values)-1]
}
func (this *MinStack) GetMin() int {
if len(this.Min)==0{
return 0
}
return this.Min[len(this.Min)-1]
}
/**
* Your MinStack object will be instantiated and called as such:
* obj := Constructor();
* obj.Push(x);
* obj.Pop();
* param_3 := obj.Top();
* param_4 := obj.GetMin();
*/
题目:二叉搜索树中第K小的数
题目描述:
给定一个二叉搜索树,编写一个函数 kthSmallest 来查找其中第 k 个最小的元素。
说明:
你可以假设 k 总是有效的,1 ≤ k ≤ 二叉搜索树元素个数。
示例 1:
输入: root = [3,1,4,null,2], k = 1
3
/
1 4
2
输出: 1
示例 2:
输入: root = [5,3,6,2,4,null,null,1], k = 3
5
/
3 6
/
2 4
/
1
输出: 3
分析:这个题很明显考的就是二叉树的中序遍历,但是有更简单的方法,先计算左子树的数量,然后判断这个数是出现在左子树还是右子树,然后递归找到这个数
代码:
/**
* Definition for a binary tree node.
* type TreeNode struct {
* Val int
* Left *TreeNode
* Right *TreeNode
* }
*/
func kthSmallest(root *TreeNode, k int) int {
t:=size(root.Left)
if t>k-1{
return kthSmallest(root.Left,k)
}else if t<k-1{
return kthSmallest(root.Right,k-t-1)
}else{
return root.Val
}
return 0
}
func size(root *TreeNode) int{
if root==nil{
return 0
}
return size(root.Left)+size(root.Right)+1
}
题目:二叉树的中序遍历
题目描述:
给定一个二叉树,返回它的中序 遍历。
示例:
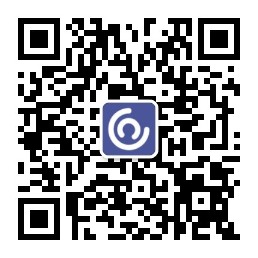
输入: [1,null,2,3]
1
2
/
3
输出: [1,3,2]
进阶: 递归算法很简单,你可以通过迭代算法完成吗?
分析:没什么难度,用迭代就用栈储存节点,go没有栈就用切片
代码:
/**
* Definition for a binary tree node.
* type TreeNode struct {
* Val int
* Left *TreeNode
* Right *TreeNode
* }
*/
func inorderTraversal(root *TreeNode) []int {
nums:=[]*TreeNode{}
res:=[]int{}
cur:=root
for len(nums)!=0 || cur!=nil{
if cur!=nil{
nums=append(nums,cur)
cur=cur.Left
}else{
cur=nums[len(nums)-1]
nums=nums[:len(nums)-1]
res=append(res,cur.Val)
cur=cur.Right
}
}
return res
}