转行学开发,代码100天——2018-04-23
一、运动基础框架
JavaScript的运动可以广义理解为渐变效果,直接移动效果等,图网页上常见的“分享到”,banner,透明度变化等。其实现的基本方法就是利用前面学到的定时器。
通过学习,总结一下基本的运动实现——运动框架。
运动框架可以理解为实现元素运动的流程。比如让一个div盒子运动起来,即给其left样式加一个定时器即可。
<input type="button" value="开始运动" id="btn"> <div id="div1"></div>
<script type="text/javascript"> var timer =null; window.onload = function () { var speed =6;//设置移动速度 var oDiv = document.getElementById('div1'); var oBtn = document.getElementById('btn'); oBtn.onclick =function(){ clearInterval(timer); //运动前需要关闭其他所以定时器 timer = setInterval(function(){ if(oDiv.offsetLeft>300) { //在指定范围内移动 clearInterval(timer); }else oDiv.style.left = oDiv.offsetLeft+speed+"px"; },200); } } </script>
注意:
1、实现运动时,往往要设置一个速度变量speed,用来控制物体的运动速度。
2、这里能实现在指定范围内的移动,但这里存在一个移动的问题,按照 距离= 速度x时间 ,往往不会恰好在指定位置停止。所以需要设置好停止条件。
扫描二维码关注公众号,回复:
52517 查看本文章
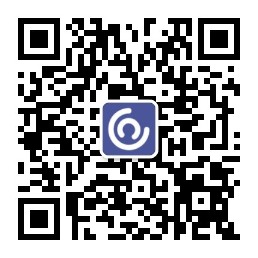
3、如果在按钮事件—开始运动之时,连续点击"开始运动“,若没有关闭其他定时器,DIV盒子会越来越快,这就不符合设计要求了。所以在运动前需要添加
clearInterval(timer);
4、这种固定速度的运动方式可以成为匀速运动。
5、运动的实现,需要物体绝对定位。position:absolute;top:0;left0;
二、应用案例
2.1 网页“分享到”“反馈”等侧边栏效果。
当鼠标移动到“分享到”区域时,div盒子向左伸出,移开鼠标时,回到原位。
按照前面对运动框架的分析,我们同样需要写一个运动的框架,将其放置到鼠标onmouseover和onmouseout事件中。
CSS样式设置:
*{margin: 0;padding: 0;}
#div1{width: 160px;height: 260px;background: #666;position: absolute;top: 200px;left: -160px;} #div1 span{width: 20px;height: 60px;font-size:12px;color:#fff;background: blue;line-height:20px;position: absolute;left:160px; top: 120px;}
//左移 function startMove1(){ var odiv = document.getElementById("div1"); clearInterval(timer); timer= setInterval(function(){ if (odiv.offsetLeft==0) { clearInterval(timer); }else { odiv.style.left = odiv.offsetLeft+10+"px"; } },200); } //右移 function startMove2(){ var odiv = document.getElementById("div1"); clearInterval(timer); timer= setInterval(function(){ if (odiv.offsetLeft==160) { clearInterval(timer); }else { odiv.style.left = odiv.offsetLeft-10+"px"; } },200); }
然后在鼠标事件中添加事件
var oDiv = document.getElementById("div1"); //鼠标移入 oDiv.onmouseover = function(){ startMove1(); }; //鼠标移出 oDiv.onmouseout = function(){ startMove2(); };
这样就实现了这个侧边栏的移出移进的动作。
但这并不意为着代码的结果,观察“左移”和“右移”的代码,高度相似,不同之处仅仅在于“偏移量”和“移动速度”。因此,可以考虑将这两种实现方式通过传参来进行优化。
增加参数1:iTarget 目标位置 ;参数2:speed 移动速度
var timer = null; //设置定时器变量 var speed = 10; //设置速度变量 function startMove(iTarget,speed){ var odiv = document.getElementById("div1"); clearInterval(timer); timer= setInterval(function(){ if (odiv.offsetLeft==iTarget) { clearInterval(timer); }else { odiv.style.left = odiv.offsetLeft+speed+"px"; } },200); }
//鼠标移入 oDiv.onmouseover = function(){ // startMove1(); startMove(0,10); }; //鼠标移出 oDiv.onmouseout = function(){ // startMove2(); startMove(-160,-10); };
如此,既能满足基本的功能效果,又能大量优化代码。