pandas采用了很多Numpy的代码风格,但是最大的不同在于pandas用来处理表格型或者异质类数据。而Numpy则相反,它更适合处理同质型的数值类数组数据。
Series
Series是一种一维的数组型对象,它包含了一个值序列,并且包含了数据标签,也就是索引。
import pandas as pd
from pandas import Series, DataFrame
obj = pd.Series([4, 7, -5, 3])
print(obj)
#输出:
#0 4
#1 7
#2 -5
#3 3
这里我们看到了左侧0-4是索引,右边是值。
可以通过values和index来获取对应的值和索引。
print(obj.values)
print(obj.index)
#[ 4 7 -5 3]
#RangeIndex(start=0, stop=4, step=1)
上面用的系统默认的索引,其实可以自己创建一个索引标签。
obj2 = pd.Series([4, 7, -5, 3], index=['d', 'b', 'a', 'c'])
print(obj2)
# d 4
# b 7
# a -5
# c 3
# dtype: int64
print(obj2.index)
Index(['d', 'b', 'a', 'c'], dtype='object')
上面发现,我们自己定义的标签替换了系统默认的标签。后期我们也可以去修改index。
obj = pd.Series([4, 7, -5, 3])
print(obj)
obj.index = ['Bob', 'Steve', 'Jeff', 'Ryan']
print(obj)
输出:
0 4
1 7
2 -5
3 3
dtype: int64
Bob 4
Steve 7
Jeff -5
Ryan 3
dtype: int64
这样我们就可以用我们自己的标签来读取数据或者修改数据。
obj2['a']
# -5
obj2['d'] = 6
obj2[['c', 'a', 'd']]
# c 3
# a -5
# d 6
# dtype: int64
上面的代码中,我们用了标签列表来获取数据。
可以用Numpy相同的风格操作,比如布尔值过滤,标量相乘,数学函数等。
print(obj2[obj2 > 0])
print(obj2 * 2)
print(np.exp(obj2))
输出结果:
d 6
b 7
c 3
dtype: int64
d 12
b 14
a -10
c 6
dtype: int64
d 403.428793
b 1096.633158
a 0.006738
c 20.085537
dtype: float64
其实可以这样理解Series,它是一个长度固定且有序的字典,因为其是将索引值与数据值按位置配对。所以我们可以使用字典中方法用于Series中。
'b' in obj2 # True
'e' in obj2 # False
如果已经有数据刚好是Python中的字典,那么可以直接使用这个字典来生成Series。
sdata = {'Ohio':35000, 'Texas':71000, 'Oregon':16000, 'Utah':5000}
obj3 = pd.Series(sdata)
print(obj3)
输出:
Ohio 35000
Texas 71000
Oregon 16000
Utah 5000
dtype: int64
用字典转换的时候,直接利用了字典的key和value配对,同时按照字典排号的字典键作为索引的顺序。同样,可以按照自己的要求来生成索引顺序。
states = ['California', 'Ohio', 'Oregon', 'Texas']
obj4 = pd.Series(sdata, index = states)
输出:
California NaN
Ohio 35000.0
Oregon 16000.0
Texas 71000.0
dtype: float64
上面的数据,我们发现California的value为NaN,这是因为对应的索引在sdata中找不到对应的key,所以缺失的值用NaN代替。而states中没有Utah,所以舍弃掉。
pandas中使用isnull和notnull函数来检查缺失数据。
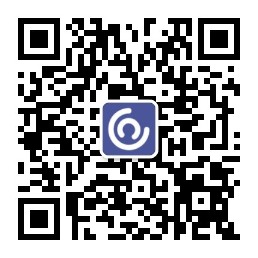
pd.isnull(obj4)
# 或 obj4.isnull()
pd.notnull(obj4)
# 或 obj4.notnull()
在pandas中,其数据操作中自动对齐索引是Series中非常有用的特性。
print(obj3)
print(obj4)
print(obj3 + obj4)
输出:
Ohio 35000
Texas 71000
Oregon 16000
Utah 5000
dtype: int64
California NaN
Ohio 35000.0
Oregon 16000.0
Texas 71000.0
dtype: float64
California NaN
Ohio 70000.0
Oregon 32000.0
Texas 142000.0
Utah NaN
dtype: float64
发现obj3+obj4结果类似于数据库中的join操作。会把两组数据合并,缺省的值会用NaN表示。
Series对象自身和其索引都有一个name熟悉。
obj4.name = 'population'
obj4.index.name = 'state'
print(obj4)
输出:
state
California NaN
Ohio 35000.0
Oregon 16000.0
Texas 71000.0
Name: population, dtype: float64