InputFiled组件(输入框)
Text Component(显示内容):显示输入内容的Text的组件
Text(输入内容):输入的文本内容
Character Limit:字符数量限值,0是无限制,中英文字符长度相同
Content Type:输入内容限值
----Standard:标准类型,什么字符都行
----Integer Number:整数类型
----Decimal Number:整数或小数类型
----Alphanumeric:字母和数字
----Name:首字母大写
----Email Address:@和.
----Password:字母和数字,星号隐藏
Line Type
----Single Line:单行,不允许换行,只有一行
----Multi Line Submit:多行,当一行不能装下所有的字符时,自动换行,不允许手动回车换行
----Multi Line Newline:多行,当一行不能装下所有的字符时,自动换行,允许手动回车换行
Placeholder:默认显示的内容Text组件(当输入内容为空时,显示的内容)
Caret Blink Rate:光标的闪烁速度
Caret Width:光标的宽度
Custom Caret Color:自定义光标的颜色
Selection Color:选中文本的背景颜色
Hide Mobile Input:隐藏手机的虚拟键盘(移动端)
Read Only:只读
自制输入框
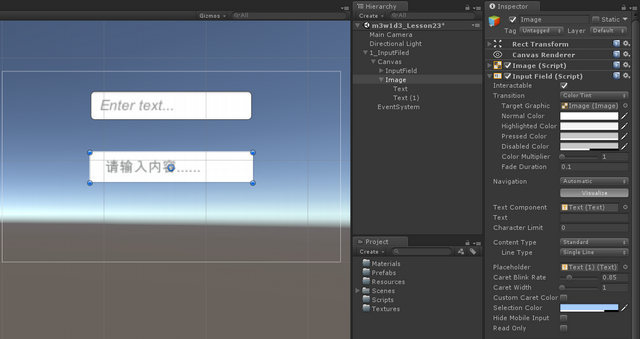
文本内容的左右边距缩进
输入内容Text组件报错
去掉Text组件的富文本选项
OnValueChanged,当输入内容Text值改变的时候,执行里边存储的所有的方法。
OnEndEdit:当输入结束的时候执行(按下回车键或光标失去焦点的时候)
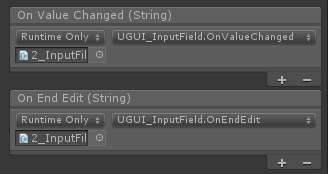
两个输入框的TAB切换
代码操作
常用
input.text//获取或者修改文本的内容
input.textComponent
input.contentType//改变文本类型
input.readOnly
input.ForceLabelUpdate();//强制更新显示内容,在改变完文本类型的情况下最好强制更新一次
input.isFocused//检查输入框是否锁定
input.Select();//选择输入框,默认只能选定一个输入框
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class UGUI_InputField : MonoBehaviour { public InputField[] inputs; private int count = 0; // Use this for initialization void Start () { //获取或者修改文本的内容 inputs[0].text = "小明"; //使用代码注册事件 inputs[0].onValueChanged.AddListener(OnValueChanged); //执行onValueChanged里的所有的方法 inputs[0].onValueChanged.Invoke(""); inputs[0].onEndEdit.AddListener(OnEndEdit); inputs[0].Select(); } // Update is called once per frame void Update () { if (Input.GetKeyDown(KeyCode.Alpha1)) { //改变文本类型 inputs[0].contentType = InputField.ContentType.Password; //强制更新显示内容,在改变完文本类型的情况下最好强制更新一次 inputs[0].ForceLabelUpdate(); } else if (Input.GetKeyDown(KeyCode.Alpha2)) { //改变文本类型 inputs[0].contentType = InputField.ContentType.Standard; inputs[0].ForceLabelUpdate(); } if (Input.GetKeyDown(KeyCode.Tab)) { //inputs[0].isFocused 是否是锁定的 if (inputs[0].isFocused) { inputs[1].Select();//选择 } else if(inputs[1].isFocused) { inputs[2].Select(); } else if (inputs[2].isFocused) { inputs[0].Select(); } } if (Input.GetKeyDown(KeyCode.Return)) { count++; inputs[count % inputs.Length].Select(); } } public void OnValueChanged(string str) { Debug.Log("OnValueChanged:" + str); } public void OnEndEdit(string str) { Debug.Log("OnEndEdit:" + str); } }
Dropdown组件(下拉菜单)
Dropdown(下拉菜单)
Template : 下拉的模板
Caption Text:当前选择的选项显示的文本组件Text
Caption Image:当前选择的选项显示的Sprite的图片Image
Item Text:模板中每个元素的Text组件
Item Image:模板中每个元素的Image组件
Value:当前选择选项的索引
Options:选项内容
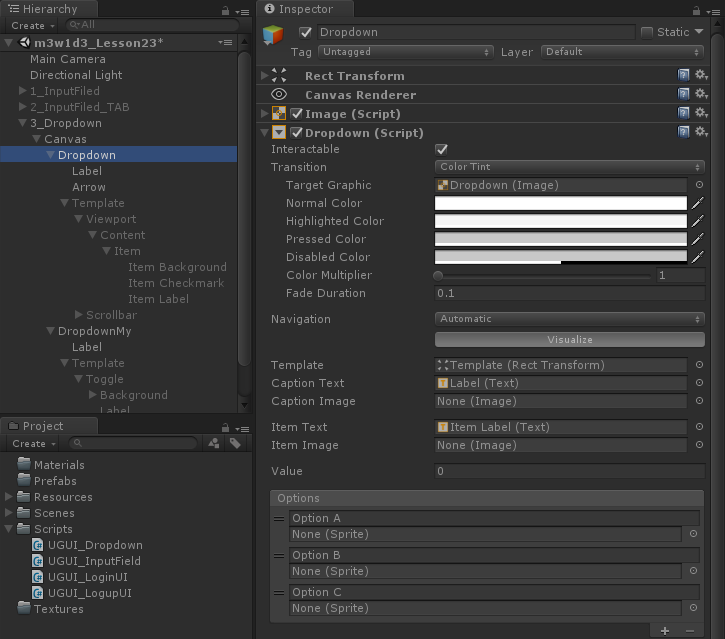
代码操作
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class UGUI_Dropdown : MonoBehaviour { public Dropdown drop; private void Awake() { drop.onValueChanged.AddListener(Test); } // Use this for initialization void Start () { //清空所有的选项 drop.options.Clear(); //drop.ClearOptions(); //选项的索引 drop.value = 1; //首先先实例化一个类对象 Dropdown.OptionData data1 = new Dropdown.OptionData("小明"); //把类对象添加到Options中 drop.options.Add(data1); //第二种添加方式 List<string> list = new List<string>(); list.Add("如花"); //有三个重载的方法,自己酌情选择 drop.AddOptions(list); } // Update is called once per frame void Update () { } public void Test(int value) { Debug.Log("你选择了" + value); } }
综合练习-登录界面
背景图尺寸1620X720,画布缩放设置1280X720(选第2项)
Shift+拖动:原比例缩放
代码操作:
登录逻辑:
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class UGUI_LoginUI : MonoBehaviour { public GameObject logupObj; private Button loginButton; private Button logupButton; private InputField usernameInput; private InputField passwordInput; private Toggle passwordToggle; private Text errorText; //暂时使用静态的两个变量存储用户民和密码 public static string user = "admin"; public static string password = "123456"; private void Awake() { loginButton = transform.Find("LoginButton").GetComponent<Button>(); logupButton = transform.Find("LogupButton").GetComponent<Button>(); usernameInput = transform.Find("User").GetComponent<InputField>(); passwordInput = transform.Find("Password").GetComponent<InputField>(); passwordToggle = transform.Find("PasswordToggle").GetComponent<Toggle>(); errorText = transform.Find("ErrorText").GetComponent<Text>(); usernameInput.onValueChanged.AddListener(UserOrPwdValueChange); passwordInput.onValueChanged.AddListener(UserOrPwdValueChange); loginButton.onClick.AddListener(LoginButtonClick); logupButton.onClick.AddListener(RegisterButtonClick); passwordToggle.onValueChanged.AddListener(ShowPwdToggleClick); } // Use this for initialization void Start () { } private void OnEnable() { errorText.enabled = false; usernameInput.text = ""; passwordInput.text = ""; } // Update is called once per frame void Update () { if (Input.GetKeyDown(KeyCode.Tab)) { if (usernameInput.isFocused) { passwordInput.Select(); } else if (passwordInput.isFocused) { usernameInput.Select(); } } } //当用户名或密码值发生改变时的事件,因为内部逻辑是相同的,所以使用了一个方法 void UserOrPwdValueChange(string value) { //当输入内容改变的时候,判断你的错误提示是否显示,如果显示让他消失 if (errorText.enabled) { errorText.enabled = false; } } //登录按钮事件 void LoginButtonClick() { //先判断用户名密码是否为空 if (usernameInput.text == null || usernameInput.text == "") { errorText.text = "请输入用户名!"; errorText.enabled = true; } else if (passwordInput.text == null || passwordInput.text == "") { errorText.text = "请输入密码!"; errorText.enabled = true; } else { if (usernameInput.text == user && passwordInput.text == password) { errorText.text = "登录成功";//暂时作为提示处理 errorText.enabled = true; } else { //当用户名密码错误时,清空你的输入框 usernameInput.text = ""; passwordInput.text = ""; errorText.text = "用户名密码错误";//暂时作为提示处理 errorText.enabled = true; } } } //注册按钮事件 void RegisterButtonClick() { logupObj.SetActive(true); gameObject.SetActive(false); } //显示密码的事件 void ShowPwdToggleClick(bool isOn) { if (isOn)//显示密码 { passwordInput.contentType = InputField.ContentType.Standard; } else { passwordInput.contentType = InputField.ContentType.Password; } passwordInput.ForceLabelUpdate(); } }
注册逻辑:
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class UGUI_LogupUI : MonoBehaviour { public GameObject loginObj; private Button returnButton; private Button submitButton; private InputField usernameInput; private InputField pwd1Input; private InputField pwd2Input; private Text errorText; void Awake() { returnButton = transform.Find("ReturnButton").GetComponent<Button>(); submitButton = transform.Find("LogupButton").GetComponent<Button>(); usernameInput = transform.Find("User").GetComponent<InputField>(); pwd1Input = transform.Find("Password").GetComponent<InputField>(); pwd2Input = transform.Find("PasswordAgain").GetComponent<InputField>(); errorText = transform.Find("ErrorText").GetComponent<Text>(); returnButton.onClick.AddListener(ReturnButtonClick); submitButton.onClick.AddListener(SubmitButtonClick); pwd1Input.onValueChanged.AddListener(PwdValueChange); pwd2Input.onValueChanged.AddListener(PwdValueChange); usernameInput.onValueChanged.AddListener(PwdValueChange); } // Use this for initialization void Start () { } private void OnEnable() { //每次进入注册界面需要初始化,错误提示去掉,所有的输入框重置 errorText.enabled = false; usernameInput.text = ""; pwd1Input.text = ""; pwd2Input.text = ""; } // Update is called once per frame void Update () { } //只要密码改不了,就需要判断错误提示是否显示,如果显示需要给隐藏 void PwdValueChange(string value) { if (errorText.enabled) { errorText.enabled = false; } } //确定按钮事件 void SubmitButtonClick() { if (usernameInput.text == null || usernameInput.text == "") { errorText.text = "请输入用户名"; errorText.enabled = true; } else if (pwd1Input.text == null || pwd1Input.text == "") { errorText.text = "请输入密码"; errorText.enabled = true; } else if (pwd2Input.text == null || pwd2Input.text == "") { errorText.text = "请确认密码"; errorText.enabled = true; } else { //所有Input都有内容的情况下,首先判断两次密码是否一致 if (pwd1Input.text == pwd2Input.text) { UGUI_LoginUI.user = usernameInput.text; UGUI_LoginUI.password = pwd1Input.text; ReturnButtonClick();//直接跳回登录界面 } else { errorText.text = "两次密码不一致!"; errorText.enabled = true; } } } void ReturnButtonClick() { loginObj.SetActive(true); gameObject.SetActive(false); } }