#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Sun Feb 3 20:28:26 2019
@author: myhaspl
sample:
Area,Value,Room,Living,School,Year,Floor
60,135,2,1,1,1944,6
87,450,3,1,1,1952,4
87,450,3,1,1,1952,4
87,500,3,1,1,1952,4
...
"""
import pandas as pd
import numpy as np
import tensorflow as tf
import pylab as plt
trainData=pd.read_csv("bjhouse.csv")
train=trainData[:1000]
trainX=train['Area'].values.reshape(-1,1)
trainY=train['Value'].values.reshape(-1,1)
sampleCount=trainX.shape[0]
learning_rate=0.8
trainingEpochs=500
dispCount=10
sx=tf.placeholder(tf.float32)
sy=tf.placeholder(tf.float32)
w=tf.Variable(np.random.randn(),name="w",dtype=tf.float32)
b=tf.Variable(np.random.randn(),name="b",dtype=tf.float32)
y_=tf.multiply(sx,w)+b
cost=tf.reduce_sum(tf.pow(y_-sy,2)/(2*sampleCount))
trainStep=tf.train.AdamOptimizer(learning_rate).minimize(cost)
init=tf.global_variables_initializer()
sess=tf.Session()
sess.run(init)
for epoch in range(trainingEpochs):
trainSample=zip(trainX,trainY)
for (x,y) in trainSample:
sess.run(trainStep,feed_dict={sx:x,sy:y})
if (epoch+1)% dispCount ==0:
trainCost=sess.run(cost,feed_dict={sx:trainX,sy:trainY})
print("epoch{},cost:{:.04f}".format(epoch+1,trainCost))
print("train finished")
print("w:{},b:{},cost:{}".format(sess.run(w),sess.run(b),sess.run(cost,feed_dict={sx:trainX,sy:trainY})))
plt.rcParams['font.sans-serif'] = ['SimHei'] # 替换sans-serif字体
plt.rcParams['axes.unicode_minus'] = False # 解决坐标轴负数的负号显示问题
plt.plot(trainX,trainY,"go",label="原数据")
plt.plot(trainX,trainX*sess.run(w),label="拟合数据")
plt.legend()
plt.show()
epoch10,cost:3925.0693
epoch20,cost:3926.9109
epoch30,cost:3927.0454
epoch40,cost:3927.0542
epoch50,cost:3927.0552
epoch60,cost:3927.0554
epoch70,cost:3927.0554
epoch80,cost:3927.0554
epoch90,cost:3927.0554
epoch100,cost:3927.0554
epoch110,cost:3927.0554
epoch120,cost:3927.0554
epoch130,cost:3927.0554
epoch140,cost:3927.0554
epoch150,cost:3927.0554
epoch160,cost:3927.0554
epoch170,cost:3927.0554
epoch180,cost:3927.0554
epoch190,cost:3927.0554
epoch200,cost:3927.0554
epoch210,cost:3927.0554
epoch220,cost:3927.0554
epoch230,cost:3927.0554
epoch240,cost:3927.0554
epoch250,cost:3927.0554
epoch260,cost:3927.0554
epoch270,cost:3927.0554
epoch280,cost:3927.0554
epoch290,cost:3927.0554
epoch300,cost:3927.0554
epoch310,cost:3927.0554
epoch320,cost:3927.0554
epoch330,cost:3927.0554
epoch340,cost:3927.0554
epoch350,cost:3927.0554
epoch360,cost:3927.0554
epoch370,cost:3927.0554
epoch380,cost:3927.0554
epoch390,cost:3927.0554
epoch400,cost:3927.0554
epoch410,cost:3927.0554
epoch420,cost:3927.0554
epoch430,cost:3927.0554
epoch440,cost:3927.0554
epoch450,cost:3927.0554
epoch460,cost:3927.0554
epoch470,cost:3927.0554
epoch480,cost:3927.0554
epoch490,cost:3927.0554
epoch500,cost:3927.0554
train finished
w:4.52463436126709,b:21.11363410949707,cost:3927.055419921875
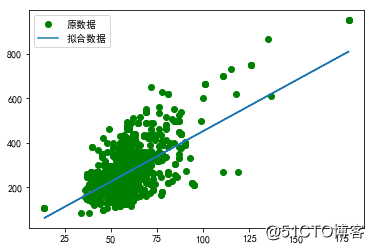