文章目录
API
- createBottomTabNavigator(
RouteConfigs,BottomTabNavigatorConfig
)
参数说明
RouteConfigs
navigationOptions
- title:底部tab上的文字,如:“首页”,“我的”
- tabBarVisibletabBarVisible:
Boolean
是否显示tabBar,如果是false将隐藏底部tabBar,默认true
- tabBarIcon:设置
title
上面的icon。
值为组件或方法,当它的值是方法的时候此方法接收{ focused, horizontal, tintColor}作为参数(此方法的返回值应该是一个组件)
horizontal:手机屏幕横着的时候horizontal为true,否则为false,当设备方向发生改变时icon重新渲染。
focused:当前tab选中的时候focused为true否则为false
tintColor:当前tab选中的时候值为tabBarOptions
中的activeTintColor
,否则为tabBarOptions
中的inactiveTintColor
- tabBarLabel:它的值可以是
字符串、组件、方法
;如果是方法那么此方法接收{ focused: boolean, tintColor: string }。未设置此属性时,title
发挥作用 - tabBarButtonComponent:值为组件
- tabBarTestID:tab button的ID
- tabBarOnPress:
Function
参数为包含navigation
和defaultHandler
的对象。
BottomTabNavigatorConfig
- initialRouteName :打开tabBar页面的时候显示的第一个页面的路由名称,它的值为RouteConfigs的一个key
- order:由RouteConfigs的key组成的一个数组,按照数组的顺序显示tabs
- backBehavior:按下返回键时的行为,
initialRoute
返回initialRouteName
对应的页面。none
返回上一页面。默认initialRoute
- lazy:
Boolean
默认true。如果为false的时候会把所有的tabs对应的页面渲染。true的时候只会打开页面的时候进行渲染 - tabBarComponent:
- tabBarOptions:
object
tabBarOptions:{
activeTintColor:"",//活跃状态的label和icon颜色
activeBackgroundColor:"",活跃状态的tab背景色
inactiveTintColor:"",//非活跃状态的label和icon颜色
inactiveBackgroundColor:"",//非活跃状态的tab背景色
showLabel:true,//是否显示tab上的文字,默认true
showIcon:true,//是否显示tab上的icon,默认true
style:{//tabBar的样式
},
labelStyle:{//tabBar上label的样式
},
tabStyle:{//tabBar上tab的样式
},
allowFontScaling:true,//
}
示例
如果BottomTabBar要使用icon需要运行以下两条命令
yarn add react-native-vector-icons
react-native link react-native-vector-icons
App.js
import React from 'react'
import {
createStackNavigator,
createBottomTabNavigator,
createAppContainer
} from 'react-navigation'
import Detail from './components/Detail'
import Home from "./components/Home";
import My from "./components/My";
import Ionicons from 'react-native-vector-icons/Ionicons'
const BottomNavigator = createBottomTabNavigator(
{
Home: {
screen: Home,
navigationOptions: {
title: "首页",
tabBarIcon:({ focused, horizontal, tintColor })=>{
return <Ionicons name={'ios-home'} size={25} style={{color:tintColor}}/>
}
}
},
Detail: {
screen: Detail,
navigationOptions: {
title: "详情",
tabBarIcon:({ focused, horizontal, tintColor })=>{
return <Ionicons name={'ios-heart'} size={25} style={{color:tintColor}}/>
}
}
},
My: {
screen: My,
navigationOptions: {
title: "我的",
tabBarIcon:({focused,horizontal,tintColor})=>{
return <Ionicons name={'logo-octocat'} size={25} style={{color:tintColor}}/>
}
}
}
},
{
tabBarOptions:{
activeTintColor:"red"
}
}
)
const StackNavigator = createStackNavigator(
{
Home: {
screen: BottomNavigator,
navigationOptions: {
title: "首页"
}
}
},
{
initialRouteName: "Home",
headerLayoutPreset: "center"
}
)
export default createAppContainer(StackNavigator)
Home.js
import React from 'react'
import {View, Text, Button} from 'react-native'
export default class Home extends React.Component{
render(){
return (
<View>
<Text>Home</Text>
</View>
)
}
}
Detail.js
import React from 'react'
import {View, Text, Button} from 'react-native'
export default class Detail extends React.Component{
render(){
return (
<View>
<Text>Detail</Text>
</View>
)
}
}
My.js
import React from 'react'
import {View, Text, Button} from 'react-native'
export default class My extends React.Component{
render(){
return (
<View>
<Text>个人中心</Text>
</View>
)
}
}
效果图
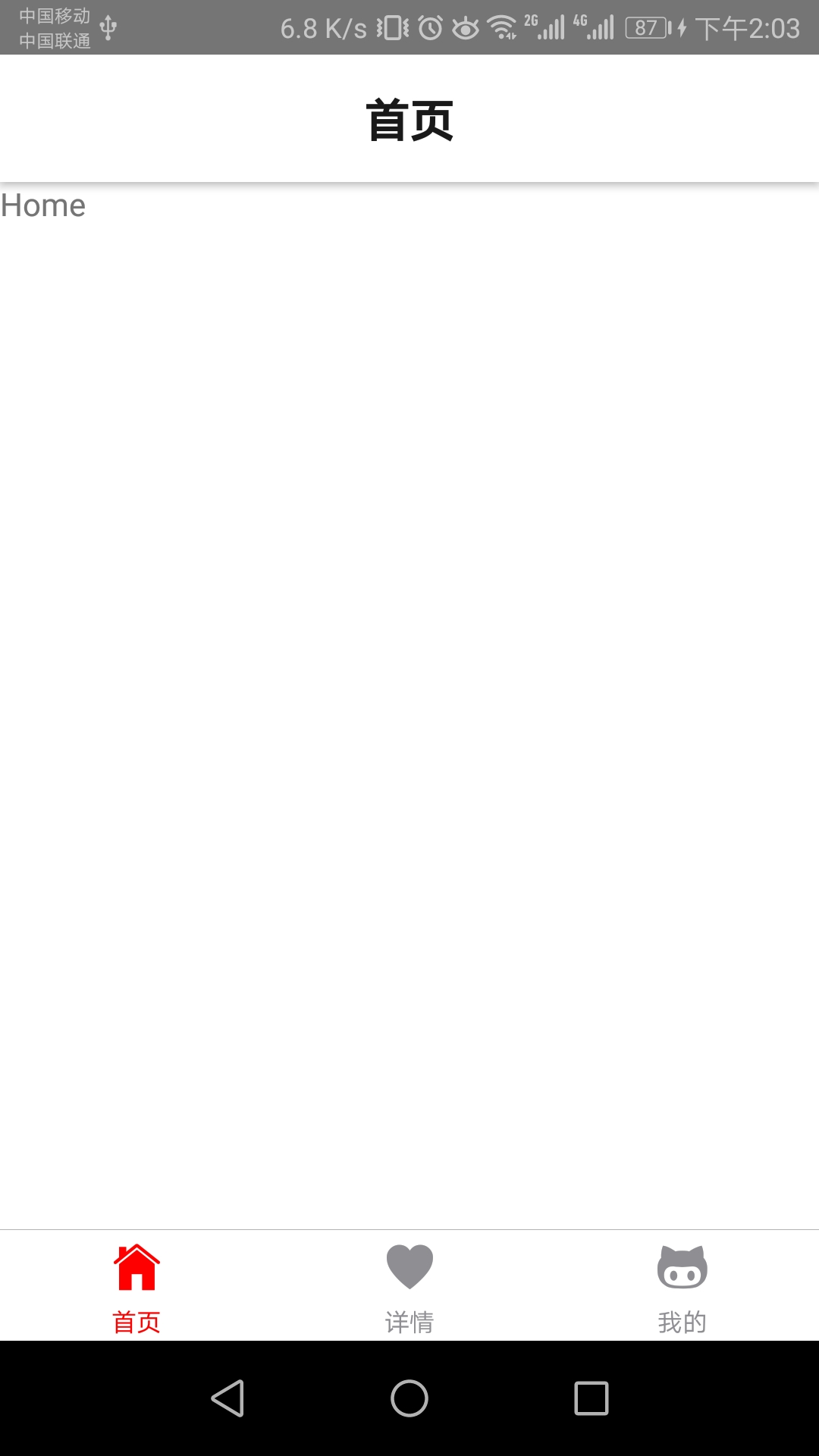