概述
本文为框架/工具/插件使用记录。
主要介绍在 Vue 2.X 项目和 Nuxt.js 项目中如何使用 vue-awesome-swiper
和 vue-lazyload
。
vue-awesome-swiper
NPM 包地址:https://www.npmjs.com/package/vue-awesome-swiper
NPM 安装:
npm install vue-awesome-swiper --save
Vue 2.X
方式一,全局使用:
import Vue from 'vue'
import VueAwesomeSwiper from 'vue-awesome-swiper'
// require styles
import 'swiper/dist/css/swiper.css'
Vue.use(VueAwesomeSwiper, /* { default global options } */)
方式二,在组件中使用:
// require styles
import 'swiper/dist/css/swiper.css'
import { swiper, swiperSlide } from 'vue-awesome-swiper'
export default {
components: {
swiper,
swiperSlide
}
}
HTML/JS用法:
<template>
<swiper :options="swiperOption">
<swiper-slide v-for="slide in swiperSlides">I'm Slide {{ slide }}</swiper-slide>
<div class="swiper-pagination" slot="pagination"></div>
</swiper>
</template>
<script>
export default {
name: 'carrousel',
data() {
return {
swiperOption: {
pagination: {
el: '.swiper-pagination'
}
},
swiperSlides: [1, 2, 3, 4, 5]
}
},
mounted() {
setInterval(() => {
console.log('simulate async data')
if (this.swiperSlides.length < 10) {
this.swiperSlides.push(this.swiperSlides.length + 1)
}
}, 3000)
}
}
</script>
Nuxt 2.3.X
方式一,全局使用:
1.在 plugins
文件夹下新建文件 vue-awesome-swiper.js
,内容是
import Vue from 'vue'
import VueAwesomeSwiper from 'vue-awesome-swiper/dist/ssr'
Vue.use(VueAwesomeSwiper)
2.在 nuxt.config.js
文件中配置插件
css: [
'@/assets/css/swiper.css'
],
plugins: [
{ src: "~/plugins/vue-awesome-swiper.js", ssr: false }
],
关于样式,我的实际做法是,将我需要的样式抽离出来,大概几十行,直接放在我页面的样式里面,没必要整个都引入。
方式二,在组件中使用:
方式二不需要新建文件也不要配置文件,直接在组件中使用。
nuxt项目中,swiper只在浏览器端引入使用,且是require形式,因为import只能写在最外层。
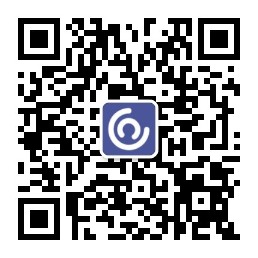
import Vue from 'vue'
// If used in nuxt.js/ssr, you should keep it only in browser build environment
if (process.browser) {
const VueAwesomeSwiper = require('vue-awesome-swiper/dist/ssr')
Vue.use(VueAwesomeSwiper)
}
export default{
data(){
//...
}
}
HTML/JS使用:
<!-- You can custom the "mySwiper" name used to find the swiper instance in current component -->
<template>
<div v-swiper:mySwiper="swiperOption" @someSwiperEvent="callback">
<div class="swiper-wrapper">
<div class="swiper-slide" v-for="banner in banners">
<img :src="banner">
</div>
</div>
<div class="swiper-pagination"></div>
</div>
</template>
<script>
export default {
data () {
return {
banners: [ '/1.jpg', '/2.jpg', '/3.jpg' ],
swiperOption: {
pagination: {
el: '.swiper-pagination'
},
// some swiper options...
}
}
},
mounted() {
setTimeout(() => {
this.banners.push('/4.jpg')
console.log('banners update')
}, 3000)
console.log(
'This is current swiper instance object', this.mySwiper,
'It will slideTo banners 3')
this.mySwiper.slideTo(3, 1000, false)
}
}
</script>
<style>
@import "@/assets/css/swiper.css";
</style>
vue-lazyload
NPM 包地址:https://www.npmjs.com/package/vue-lazyload
NPM 安装:
npm install vue-lazyload -D
Vue 2.X
main.js
import Vue from 'vue'
import App from './App.vue'
import VueLazyload from 'vue-lazyload'
Vue.use(VueLazyload)
// or with options
Vue.use(VueLazyload, {
preLoad: 1.3, //预加载的宽高比
error: 'dist/error.png', //图片加载失败时使用的图片源
loading: 'dist/loading.gif', //图片加载的路径
attempt: 1 //尝试加载次数
})
new Vue({
render: h => h(App),
}).$mount('#app')
在模板中简单使用,复杂的请看API:
<ul>
<li v-for="img in list">
<img v-lazy="img.src" >
</li>
</ul>
Nuxt 2.3.X
方式一,全局使用:
1.在 plugins
文件夹下新建文件 vue-lazyload.js
,内容是
import Vue from 'vue'
import VueLazyload from 'vue-lazyload'
Vue.use(VueLazyload)
// or with options
Vue.use(VueLazyload, {
preLoad: 1.3, //预加载的宽高比
error: 'dist/error.png', //图片加载失败时使用的图片源
loading: 'dist/loading.gif', //图片加载的路径
attempt: 1 //尝试加载次数
})
2.在 nuxt.config.js
文件中配置插件
plugins: [
{ src: "~/plugins/vue-lazyload.js" ,ssr: false}
],
方式二,在组件中使用:
方式二不需要新建文件也不要配置文件,直接在组件中使用。
import Vue from 'vue'
import VueLazyload from 'vue-lazyload'
Vue.use(VueLazyload)
在模板中简单使用,复杂的请看API:
<ul>
<li v-for="img in list">
<img v-lazy="img.src" >
</li>
</ul>
总结
这类技术使用记录文章本来不想写的,因为觉得没有太大的意义,但是想到自己在实践的过程中,因为一个小问题,网站找不到解决方案而浪费很多心力的时候,就想着还是要记录一下,没准需要的同学就因为其中的一个小问题而困恼呢,还是希望这种各种工具使用起来坑能少些。
END.