版权声明:辛辛苦苦写的,转载请注明出处噢 https://blog.csdn.net/wangjiangrong/article/details/80738778
前言
最近想研究下帧同步的问题,首先先解决unity客户端和服务器的通信问题。被之前的同事一直安利nodejs,所以自己就尝试下用nodejs搭建服务器,来实现和unity的通信。
本文用的第三方插件为Socket.IO,在unity的asset store中大家可以自行去下载
NodeJS部分
首先搭建一个空的nodejs工程,然后安装我们需要的两个软件包,express和socket.io
npm install express
npm install socket.io
安装成功后新建一个main.js脚本,内容如下,这段代码的作用通俗点讲就是启动一个socket,当有客户端连接的时候就会执行connection事件中的函数体。
// 官方连接:https://socket.io/
var io = require('socket.io')(8078);
console.log('server start');
io.on('connection', function (socket) {
console.log('client connection');
//触发客户端注册的自定义事件
socket.emit('ClientListener', { hello: 'world' });
//注册服务器的自定义事件
socket.on('ServerListener', function (data, callback) {
console.log('ServerListener email:' + data['email']);
callback({ abc: 123 });
});
//断开连接会发送
socket.on('disconnect', function () {
console.log('client disconnected');
});
});
然后我们在命令行下启动这个服务器即可:
Unity客户端部分:
下载好Socket.IO后,导入我们的工程当中,然后将SocketIO的prefab拖入到场景当中,修改url当中的ip地址为我们nodejs中的ip地址。
这个时候,其实我们只有启动客户端,就会发现已经能够连接上nodejs服务器了。如图
扫描二维码关注公众号,回复:
4943611 查看本文章
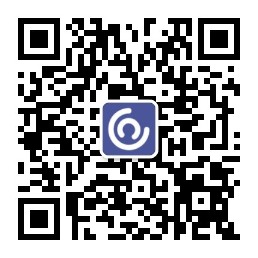
紧接着,我们可以写一下业务代码,新建一个SocketManager.cs文件,用来管理这个socket组件,绑定在SocketIO物体上,如下:
using SocketIO;
using System.Collections.Generic;
using UnityEngine;
namespace Tool {
public class SocketManager : MonoBehaviour {
SocketIOComponent m_socket;
void Start () {
m_socket = GetComponent<SocketIOComponent>();
if(m_socket != null) {
//系统的事件
m_socket.On("open", OnSocketOpen);
m_socket.On("error", OnSocketError);
m_socket.On("close", OnSocketClose);
//自定义的事件
m_socket.On("ClientListener", OnClientListener);
Invoke("SendToServer", 3);
}
}
public void SendToServer() {
Dictionary<string, string> data = new Dictionary<string, string>();
data["email"] = "[email protected]";
m_socket.Emit("ServerListener", new JSONObject(data), OnServerListenerCallback);
//断开连接,会触发close事件
//socket.Close();
}
#region 注册的事件
public void OnSocketOpen(SocketIOEvent ev) {
Debug.Log("OnSocketOpen updated socket id " + m_socket.sid);
}
public void OnClientListener(SocketIOEvent e) {
Debug.Log(string.Format("OnClientListener name: {0}, data: {1}", e.name, e.data));
}
public void OnSocketError(SocketIOEvent e) {
Debug.Log("OnSocketError: " + e.name + " " + e.data);
}
public void OnSocketClose(SocketIOEvent e) {
Debug.Log("OnSocketClose: " + e.name + " " + e.data);
}
#endregion
public void OnServerListenerCallback(JSONObject json) {
Debug.Log(string.Format("OnServerListenerCallback data: {0}", json));
}
}
}
接着,我们启动客户端,就会有如下Log,说明我们socket连接成功啦!!!
后续的知识以及更深入的理解还在慢慢研究ing。。。