一 快速排序
1 法一
#include <stdio.h>
int Partition(int a[],int low,int high) //返回枢纽位置
{
int key=a[low]; //把第一个元素作为枢纽元素
while(low<high)
{
while(low<high&&a[high]>=key) --high; //从后面循环,若元素大于枢纽,继续循环
//否则把低位元素赋值为小于枢纽元素的值,已经保存过低位元素,不用交换,直接替换即可
a[low]=a[high];
//从前面循环,若小于枢纽,继续往前走,不然把该值赋给高位元素
while(low<high&&a[low]<=key) ++low;
a[high]=a[low];
}
//当low为high时,退出循环
a[low]=key; //把枢纽值还给它
return low;
}
void QSort(int a[],int low,int high)
{
if(low<high)
{
int pivotkey=Partition(a,low,high);
QSort(a,low,pivotkey-1);
QSort(a,pivotkey+1,high);
}
}
int main()
{
int a[]={5};
QSort(a,0,0);
printf("a排序后是:\n");
for(int i=0;i<1;i++)
printf("%d",a[i]);
printf("\n");
int b[]={4,2};
QSort(b,0,1);
printf("b排序后是:\n");
for(int i=0;i<2;i++)
printf("%d",b[i]);
printf("\n");
int c[]={3,1,5,2,7,9,5,0,8};
QSort(c,0,8);
printf("c排序后是:\n");
for(int i=0;i<9;i++)
printf("%d",c[i]);
}
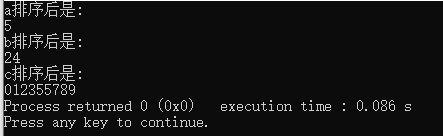
2 法二:
#include <cstdio>
#include <stdexcept>
Swap(int *a,int *b) //按指针传递交换数据
{
int t=*a;
*a=*b;
*b=t;
}
int Partition(int a[],int first,int last)
{
//if(a==NULL||first<0||last<0)
//throw new std::exception("Invalid Parameters!");
int index=first; //a[index]作为枢纽值的下标
Swap(&a[index],&a[last]); //把枢纽值放到最后
int small=first-1; //small表示目前已经确定的比枢纽值小的数组值的最大下标
for(int i=first;i<last;i++)
{
if(a[i]<a[last])
{
++small;
if(small!=i) //如果不等的话,说明small下标的值大于或等于枢纽值,需要交换,以保证small下标所指的值小于枢纽值
{
Swap(&a[small],&a[i]);
}
}
}
++small; //这个位置上的值依然小于枢纽值,同时为了把枢纽值换到中间
Swap(&a[small],&a[last]);
return small;
}
void QuickSort(int a[],int first,int last)
{
if(first==last)
return;
int pivot=Partition(a,first,last);
if(pivot>first)
QuickSort(a,first,pivot-1);
if(pivot<last)
QuickSort(a,pivot+1,last);
}
int main()
{
int a[]={5};
QuickSort(a,0,0);
printf("a排序后是:\n");
for(int i=0;i<1;i++)
printf("%d",a[i]);
printf("\n");
int b[]={4,2};
QuickSort(b,0,1);
printf("b排序后是:\n");
for(int i=0;i<2;i++)
printf("%d",b[i]);
printf("\n");
int c[]={3,1,5,2,7,9,5,0,8};
QuickSort(c,0,8);
printf("c排序后是:\n");
for(int i=0;i<9;i++)
printf("%d",c[i]);
}
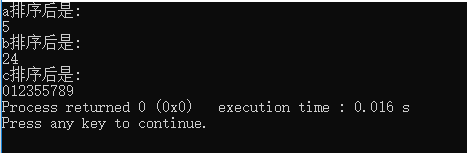
二 归并排序
#include <stdlib.h>
#include <stdio.h>
void Merge(int sourceArr[],int tempArr[], int startIndex, int midIndex, int endIndex)
{
int i = startIndex, j=midIndex+1, k = startIndex;
while(i!=midIndex+1 && j!=endIndex+1)
{
if(sourceArr[i] > sourceArr[j])
tempArr[k++] = sourceArr[j++];
else
tempArr[k++] = sourceArr[i++];
}
while(i != midIndex+1)
tempArr[k++] = sourceArr[i++];
while(j != endIndex+1)
tempArr[k++] = sourceArr[j++];
for(i=startIndex; i<=endIndex; i++)
sourceArr[i] = tempArr[i];
}
//内部使用递归
void MergeSort(int sourceArr[], int tempArr[], int startIndex, int endIndex)
{
int midIndex;
if(startIndex < endIndex)
{
midIndex = startIndex + (endIndex-startIndex) / 2;//避免溢出int
MergeSort(sourceArr, tempArr, startIndex, midIndex);
MergeSort(sourceArr, tempArr, midIndex+1, endIndex);
Merge(sourceArr, tempArr, startIndex, midIndex, endIndex);
}
}
int main(int argc, char * argv[])
{
int a[9] = {50, 10, 20, 30, 70, 40, 80, 60,50};
int i, b[9];
MergeSort(a, b, 0, 8);
for(i=0; i<9; i++)
printf("%d ", a[i]);
printf("\n");
return 0;
}
