项目环境
jdk1.8
spring4.1.7
tomcat8.5.37
集成步骤
1.在pom文件中添加swagger依赖
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.4.0</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.4.0</version>
<exclusions>
<exclusion>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>19.0</version>
</dependency>
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-jdk8</artifactId>
<version>1.1.0.Final</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>${jackson.verson}</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>${jackson.verson}</version>
<exclusions>
<exclusion>
<artifactId>jackson-annotations</artifactId>
<groupId>com.fasterxml.jackson.core</groupId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>${jackson.verson}</version>
</dependency>
2.创建swagger配置类:SwaggerConfig.java
@EnableSwagger2
@ComponentScan(basePackages = {"cn.smbms.controller"})
@Configuration
public class SwaggerConfig extends WebMvcConfigurationSupport {
@Bean
public Docket createRestApi() {
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("超市订单管理系统API")
.termsOfServiceUrl("")
.contact("")
.version("1.0")
.build();
}
}
3.修改Spring MVC配置文件 springmvc-servlet.xml
<mvc:default-servlet-handler/> //这个一定要加上否则访问不到swagger-ui.html
<context:component-scan base-package="cn.smbms.controller"></context:component-scan>
4.在工程中使用swagger
创建LoginController:
@Api(value="API")
@Controller
public class LoginController {
@Resource
private UserService userService;
@ApiOperation(value = "系统管理员登录", httpMethod = "POST",
protocols = "HTTP",produces = "application/json",
response = Object.class,notes = "管理员登录"+
"<p>登录成功:flag = ‘true’ | 用户名或密码错误:flag = ‘false’ </p>" )
@RequestMapping(value="/dologin.html",method=RequestMethod.POST)
@ResponseBody
public Object doLogin(
@ApiParam(required = true, name = "userCode", value ="用户名")
@RequestParam String userCode,
@ApiParam(required = true, name = "userPassword", value ="用户密码")
@RequestParam String userPassword)throws Exception{
Map<String,String> map = new HashMap<String,String>();
User user =userService.login(userCode, userPassword);
if(null!=user){
map.put("flag", "true");
}else{
map.put("flag","false");
}
return JSON.toJSONString(map);
}
}
创建UserController:
@Api(value="API")
@Controller
@RequestMapping("/sys/user") //http://localhost:8080/smbms/sys/user/add.html
public class UserController {
@Resource
private UserService userService;
@ApiOperation(value="添加用户",httpMethod="POST",produces="application/json",
protocols = "HTTP",response = Object.class,notes = "管理员登录"+
"<p>添加用户成功:flag = ‘true’ | 添加用户失败:flag = ‘false’ </p>" )
@RequestMapping(value="/add.html",method=RequestMethod.POST)
@ResponseBody
public Object addSave(
@RequestBody User user){
Map<String,String> map = new HashMap<String, String>();
user.setCreatedBy(1);
user.setCreationDate(new Date());
if(userService.add(user)){
map.put("flag", "true");
}else{
map.put("flag", "false");
}
return JSON.toJSONString(map);
}
}
因为以上的 addSave方法参数为一个User对象,所以需要在User类中标注swagger注解以说明属性
@ApiModel(value="User",description="用户信息")
public class User {
@ApiModelProperty("[非必填] 用户ID")
private Integer id; //id
@ApiModelProperty("[必填] 用户名")
private String userCode;
@ApiModelProperty("[非必填] 用户姓名")
private String userName;
@ApiModelProperty("[必填] 用户密码,长度不能小于6位")
private String userPassword;
@ApiModelProperty("[非必填] 用户出生日期")
private Date birthday;
@ApiModelProperty("[必填] 用户性别")
private Integer gender;
@ApiModelProperty("[必填] 用户手机号")
private String phone;
@ApiModelProperty("[非必填] 用户地址")
private String address;
@ApiModelProperty("[必填] 用户角色 1.管理员 2.部门经理 3.普通员工")
private Integer userRole;
@ApiModelProperty("[非必填]后端从session中获取")
private Integer createdBy;
@ApiModelProperty("[非必填]后端获取系统当前日期")
private Date creationDate;
@ApiModelProperty("[非必填]后端从session中获取")
private Integer modifyBy;
@ApiModelProperty("[非必填]后端获取系统当前日期")
private Date modifyDate;
private String userRoleName;
private String idPicPath;
private String workPicPath;
public String getWorkPicPath() {
return workPicPath;
}
public void setWorkPicPath(String workPicPath) {
this.workPicPath = workPicPath;
}
public String getIdPicPath() {
return idPicPath;
}
public void setIdPicPath(String idPicPath) {
this.idPicPath = idPicPath;
}
public User(){}
public User(Integer id,String userCode,String userName,String userPassword,Integer gender,Date birthday,String phone,
String address,Integer userRole,Integer createdBy,Date creationDate,Integer modifyBy,Date modifyDate){
this.id = id;
this.userCode = userCode;
this.userName = userName;
this.userPassword = userPassword;
this.gender = gender;
this.birthday = birthday;
this.phone = phone;
this.address = address;
this.userRole = userRole;
this.createdBy = createdBy;
this.creationDate = creationDate;
this.modifyBy = modifyBy;
this.modifyDate = modifyDate;
}
public String getUserRoleName() {
return userRoleName;
}
public void setUserRoleName(String userRoleName) {
this.userRoleName = userRoleName;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserCode() {
return userCode;
}
public void setUserCode(String userCode) {
this.userCode = userCode;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getUserPassword() {
return userPassword;
}
public void setUserPassword(String userPassword) {
this.userPassword = userPassword;
}
public Integer getGender() {
return gender;
}
public void setGender(Integer gender) {
this.gender = gender;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Integer getUserRole() {
return userRole;
}
public void setUserRole(Integer userRole) {
this.userRole = userRole;
}
public Integer getCreatedBy() {
return createdBy;
}
public void setCreatedBy(Integer createdBy) {
this.createdBy = createdBy;
}
public Date getCreationDate() {
return creationDate;
}
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
public Integer getModifyBy() {
return modifyBy;
}
public void setModifyBy(Integer modifyBy) {
this.modifyBy = modifyBy;
}
public Date getModifyDate() {
return modifyDate;
}
public void setModifyDate(Date modifyDate) {
this.modifyDate = modifyDate;
}
}
说明:以上的login和addSave方法调用了逻辑层的业务方法,大家可以使用伪代码模拟业务方法即可。只需按照以上内容创建三个类即可。
上传到服务器访问:swagger-ui.html
直接通过tomcat访问:
http://IP:port/{context-path}/swagger-ui.html
生产环境下,只开放80端口,通过Tomcat无法访问Swagger
所以只能通过Nginx进行Swagger的访问
修改nginx.conf :需要注释掉root节 以及 .html的缓存
http://IP/{context-path}/swagger-ui.html
swagger注解说明
下面针对以上内容使用到的swagger注解进行说明:
@Api 表明可供swagger展示的接口类(标注在类上)
@ApiOperation描述API方法(用在方法上)
@ApiOperation(value = “接口说明”,
httpMethod = “接口请求方式”,
produces = “接口返回值的具体类型”,
response = 接口返回的对象,
protocols = “通讯协议(e.g:http, https)”,
notes = “接口发布说明")
@ApiParam单个参数描述
@ApiModel
用对象接收参数(用在类上面)
@ApiModelProperty
用对象接收参数时,描述对象的一个字段(用在属性上面)
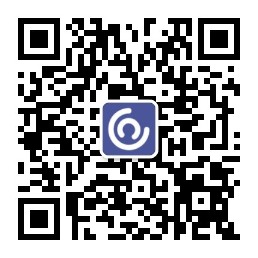