击打小球
from tkinter import *
import random
import time
class Ball:
def __init__(self, canvas, paddle, color):
self.canvas = canvas
self.paddle = paddle
self.id = canvas.create_oval(10, 10, 25, 25, fill=color)# canvas.create_oval(x0, y0, x1, y1, options)
self.canvas.move(self.id, 245, 100)# 小球起始位置(245,100)
starts = [-3, -2, -1, 1, 2, 3]
random.shuffle(starts) # 将序列的所有元素随机排序。
self.x = starts[0]
self.y = -3
self.canvas_width = self.canvas.winfo_width()# 调用winfo_width()函数来获取画布的宽度
self.canvas_height = self.canvas.winfo_height()# 调用winfo_height函数来获取画布的高度
self.hit_bottom = False
def hit_paddle(self, pos):
paddle_pos = self.canvas.coords(self.paddle.id)# paddle_pos(x0,y0,x1,y1),左上角和右下角坐标
if pos[2] >= paddle_pos[0] and pos[0] <= paddle_pos[2]: #x_bottom>=x0&&x_top<=x1
if pos[3] >= paddle_pos[1] and pos[3] <= paddle_pos[3]: #y_bottom>=y0&&y_bottom<=y1
return True # 没有击打到木板
return False # 击打到木板
def draw(self):
self.canvas.move(self.id, self.x, self.y)# 小球x轴加self.x,y轴加self.y
pos = self.canvas.coords(self.id) #获取某个对象在画布的坐标,返回一个数组(两个坐标,左上角的坐标和右下角的两个坐标)
if pos[3] >= self.canvas_height:
self.hit_bottom = True
print("你输了!")
print(ball.hit_bottom)
if pos[1] <= 0: #如果小球右上角的纵坐标小于顶,则往上移动三个像素
self.y = 3
if self.hit_paddle(pos) == True:#如果没有击打到paddle,那么向下边移动3像素
self.y = -3
if pos[0] <= 0:#如果在左边框了,那么向右边移动3像素
self.x = 3
if pos[2] >= self.canvas_width:#如果到右边框了,左移动3像素
self.x = -3
print(self.canvas.coords(self.id)) # 输出当前小球坐标
class Paddle:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_rectangle(0, 0, 100, 10, fill=color)#create_rectangle(x0, y0, x1, y1, option, ...),左上角和右下角坐标
self.canvas.move(self.id, 200, 389)
self.x = 0
self.canvas_width = self.canvas.winfo_width()
self.canvas.bind_all('<KeyPress-Left>', self.turn_left) # 左移
self.canvas.bind_all('<KeyPress-Right>', self.turn_right)# 右移
def turn_left(self, evt):#evt是向系统注册的事件
self.x = -2
def turn_right(self, evt):
self.x = 2
def draw(self):
self.canvas.move(self.id, self.x, 0)
pos = self.canvas.coords(self.id)
if pos[0] <= 0: # 若木板移动到最左边,则不能再移动
self.x = 0
elif pos[2] >= self.canvas_width: # 若木板移动到最右边,则不能再移动
self.x = 0
tk = Tk()
tk.title("Game")
tk.resizable(0, 0)# 使窗口的大小不可调整。参数(0,0)是指在水平方向和垂直方向都不改变
tk.wm_attributes("-topmost", 1) # wm_attributes告诉tkinter把包含我们画布的窗口放到所有其他窗口之前(-topmost)
canvas = Canvas(tk, width=500, height=400, bd=0, highlightthickness=0)# bd和highlightthickness确保画布之外没有边框
canvas.pack()
tk.update()
paddle = Paddle(canvas, 'blue')
ball = Ball(canvas, paddle, 'red')
while 1:
if ball.hit_bottom == False:
ball.draw()
paddle.draw()
else:
break
ball.draw()
paddle.draw()
tk.update_idletasks() # update_idletaske和update命令让tkinter快速重画屏幕
tk.update()
time.sleep(0.02)
print("game over")
tk.mainloop()
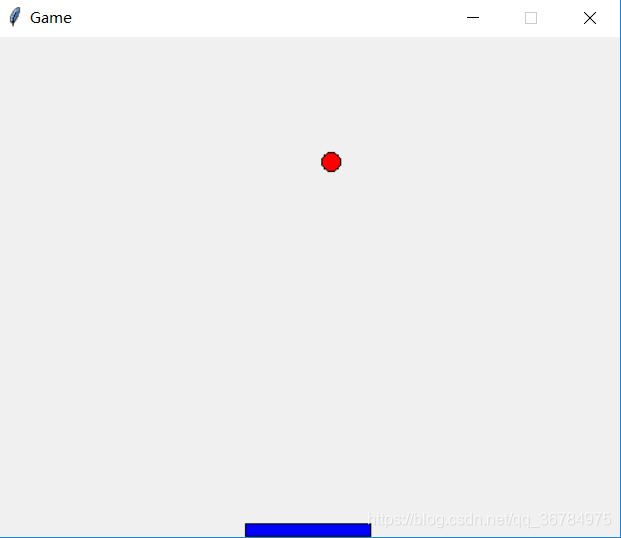