版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/Neo233/article/details/85385417
Return all non-negative integers of length N
such that the absolute difference between every two consecutive digits is K
.
Note that every number in the answer must not have leading zeros except for the number 0
itself. For example, 01
has one leading zero and is invalid, but 0
is valid.
You may return the answer in any order.
Example 1:
Input: N = 3, K = 7 Output: [181,292,707,818,929] Explanation: Note that 070 is not a valid number, because it has leading zeroes.
Example 2:
Input: N = 2, K = 1 Output: [10,12,21,23,32,34,43,45,54,56,65,67,76,78,87,89,98]
Note:
1 <= N <= 9
0 <= K <= 9
这个题的意思是:N为数的位数,K为可以调整的数的大小
class Solution {
public int[] numsSameConsecDiff(int N, int K) {
if(N == 1){
return new int[]{0,1,2,3,4,5,6,7,8,9};
}//如果为1的话就是所有的个位数,注意0也包含在内
int array[] = new int[]{1,2,3,4,5,6,7,8,9};//1到9进行循环
for(int i = 2 ; i <= N;++i){//位数的循环
ArrayList<Integer> list = new ArrayList<>();//易于添加
for(int j : array){//调取array中的数组
int y = j % 10;//抽取出个位数来
if(K + y < 10){
list.add(10 * j + y + K);
}//加上K之后的数
if(y - K >= 0 && K != 0){
list.add(10 * j + y - K);
}//减掉K之后的数
}
array = list.stream().mapToInt(j->j).toArray();//将list中的数转化为array中的数
}
return array;
}
}
假如N=3,K=8,array中数字的变化:
1,2,3,4,5,6,7,8,9
19,80,91
191,808,919
扫描二维码关注公众号,回复:
4732882 查看本文章
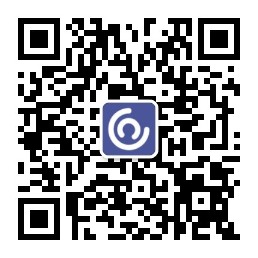
其中代码中的list.stream().mapToInt().toArray()为Java8中的语法,其中stream是将list中的数变为数据流,mapToInt是将流转化为list,其中的J->J是转化的方式,就是1:1映射(如果为J->J*J则为平方的方式转化),toArray是将之前转化的数变为Array的形式。
以下附上Java8的文档:Java8