Once Grisha found a tree (connected graph without cycles) with a root in node 1.
But this tree was not just a tree. A permutation p of integers from 0 to n−1 is written in nodes, a number pi is written in node i.
As Grisha likes to invent some strange and interesting problems for himself, but not always can solve them, you need to help him deal with two types of queries on this tree.
Let's define a function MEX(S), where S is a set of non-negative integers, as a smallest non-negative integer that is not included in this set.
Let l be a simple path in this tree. So let's define indices of nodes which lie on l as u1, u2, …, uk.
Define V(l) as a set {pu1, pu2, … , puk}.
Then queries are:
- For two nodes i and j, swap pi and pj.
- Find the maximum value of MEX(V(l)) in all possible l.
The first line contains a single integer n (2≤n≤2⋅105) — the number of nodes of a tree.
The second line contains n integers — p1, p2, …, pn (0≤pi<n) — the permutation p, it's guaranteed that all numbers are different .
The third line contains n−1 integers — d2, d3, …, dn (1≤di<i), where di is a direct ancestor of node i in a tree.
The fourth line contains a single integer q (1≤q≤2⋅105) — the number of queries.
The following q lines contain the description of queries:
At the beginning of each of next q lines, there is a single integer t (1 or 2) — the type of a query:
- If t=1, the line also contains two integers i and j (1≤i,j≤n) — the indices of nodes, where values of the permutation should be swapped.
- If t=2, you need to find the maximum value of MEX(V(l)) in all possible l.
For each type 2 query print a single integer — the answer for this query.
6 2 5 0 3 1 4 1 1 3 3 3 3 2 1 6 3 2
3 2
6 5 2 1 4 3 0 1 1 1 3 3 9 2 1 5 3 2 1 6 1 2 1 4 2 2 1 1 6 2
3 2 4 4 2
Number written in brackets is a permutation value of a node.
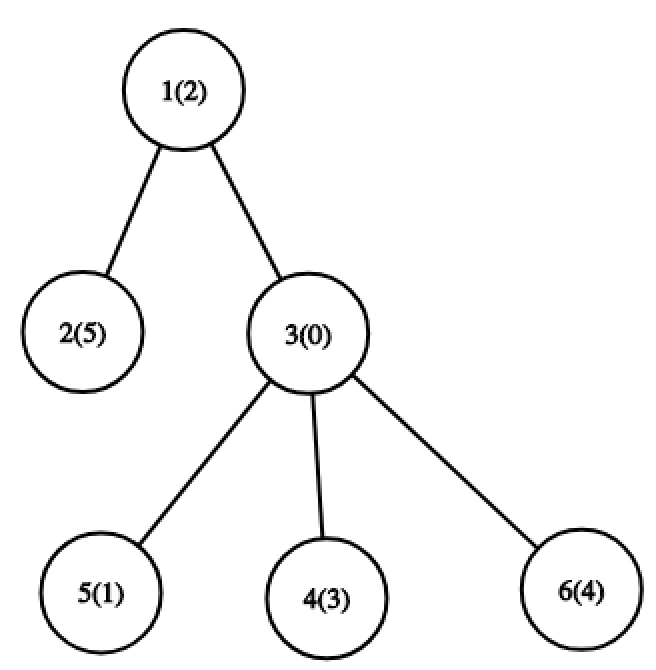

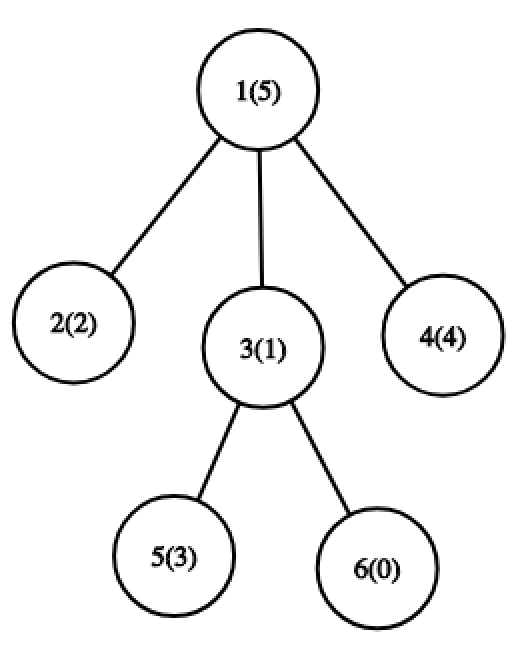

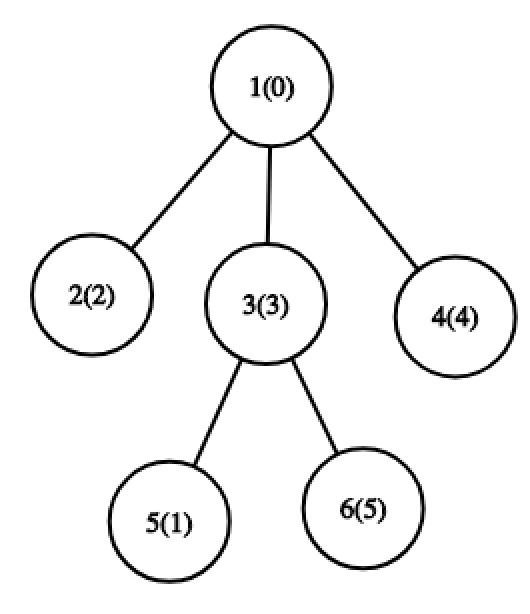
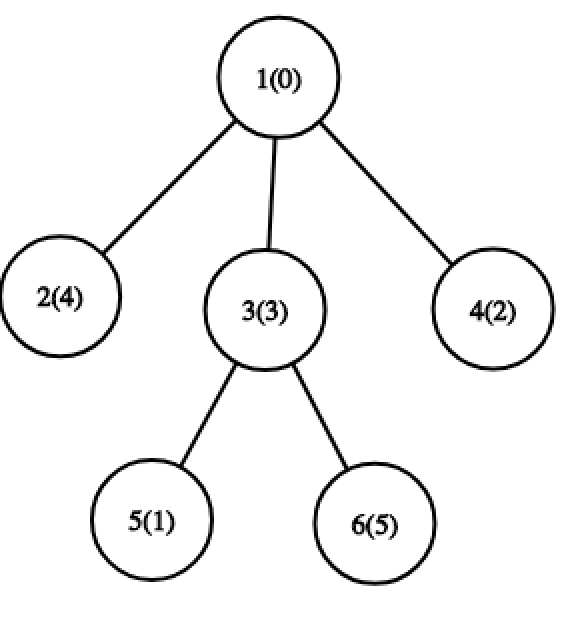