版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/qq_39591494/article/details/85254996
Python SMTP发送邮件
一、邮件的几个要素
使用代码发邮件的好处
- 可以批量发邮件,减少人工劳动
- 可以自动,定时,报警,报告等需求
电子邮件的工作原理
简单邮件传输协议(Simple Mail Transfer Protocol, SMTP)
是事实上的在Internet
传输Email
的标准。SMTP是一个相对简单的基于文本的协议。在其之上指定了一条消息的一个或多个接收者 (在大多数情况下被确认是存在的) ,然后消息文本会被传输。
发送邮件分几步?
- 打开邮件
- 登陆
- 写正文
- 发送
- 退出
Python里面怎么发邮件?
smtplib模块,使用smtp对象进行各种操作
方法 | 描述 |
---|---|
SMTP.set_debuglevel(level) |
设置输出debug调试信息,默认不输出 |
SMTP.docmd(cmd[,argstring] | 发送一个命令到SMTP服务器) |
SMTP.connect ([host[, port]]) | 连接到指定的SMTP服务器 |
SMTP.helo([hostname]) | 使用helo指令向SMTP服务器确认你的身份 |
SMTP.ehlo(hostname) |
使用ehlo指令像ESMTP(SMTP扩展)确认你的身份 |
SMTP.ehlo_or_helo_if_needed() | 如果在以前的会话连接中没有提供ehlo或者helo指令,这个方法会调用ehlo()或helo() |
SMTP.has_extn(name) | 判断指定名称是否在SMTP服务器上 |
SMTP.verify(address) | 判断邮件地址是否在SMTP服务器上 |
SMTP.starttls([keyfile[, certfile]]) | 使SMTP连接运行在TLS模式,所有的SMTP指令都会被加密 |
SMTP.login(user, password) |
登录SMTP服务器 |
SMTP.sendmail(from_addr, to_addrs, msg,mail_options=[], rcpt_options=[]) | 发送邮件 from_addr:邮件发件人 to_addrs:邮件收件人 msg:发送消息 |
SMTP.quit() |
关闭SMTP会话 |
SMTP.close() | 关闭SMTP服务器连接 |
怎么使用Python发邮件?
Python创建 SMTP 对象语法如下:
import smtplib
smtpObj = smtplib.SMTP( [host [, port [, local_hostname]]] )
参数说明:
- host: SMTP 服务器主机。 你可以指定主机的ip地址或者域名如: csdn.com,这个是可选参数。
- port: 如果你提供了 host 参数, 你需要指定 SMTP 服务使用的端口号,一般情况下 SMTP 端口号为25。
- local_hostname: 如果 SMTP 在你的本机上,你只需要指定服务器地址为 localhost 即可。
Python SMTP 对象使用 sendmail 方法发送邮件,语法如下:
SMTP.sendmail(from_addr, to_addrs, msg[, mail_options, rcpt_options])
参数说明:
- from_addr: 邮件发送者地址。
- to_addrs: 字符串列表,邮件发送地址。
- msg: 发送消息
这里要注意一下第三个参数,msg 是字符串,表示邮件。我们知道邮件一般由标题,发信人,收件人,邮件内容,附件等构成,发送邮件的时候,要注意 msg 的格式。这个格式就是 smtp 协议中定义的格式。
发个邮件普通邮件
#!/usr/bin/env python
# -*- coding:utf-8 -*-
import smtplib
from smtplib import SMTP_SSL
from email.header import Header
from email.mime.text import MIMEText
def get_password(): # 获取邮箱授权码
return "邮箱授权码"
def send_email(username):
smtp = SMTP_SSL("smtp.qq.com","465") # 邮件服务器地址
smtp.login("[email protected]", get_password()) # 邮件账户密码
text = f"{username}: \n 欢迎来到'延瓒@Yankerp'CSDN博客站点..."
msg = MIMEText(text, "plain", "utf-8")
msg["Subject"] = Header("欢迎您来到延瓒@Yankerp", "utf-8") # 邮件的标题.
msg["from"] = "[email protected]"
msg["to"] = "[email protected]"
smtp.sendmail("[email protected]", "[email protected]", msg.as_string())
smtp.quit()
if __name__ == "__main__":
send_email("亲")
发个带附件邮件
def send_email_attach(body, attachment):
smtp = SMTP_SSL("smtp.qq.com") # 邮件服务器地址
smtp.login("[email protected]", get_password()) # 邮件账户密码
msg = MIMEMultipart() # 构造一个MIMEMultipart对象代表邮件本身
msg["Subject"] = Header("欢迎您来到延瓒@Yankerp", "utf-8")
# plain代表纯文本
msg.attach(MIMEText(body, "plain", "utf-8"))
with open(attachment, "rb") as f:
mime = MIMEBase("text", "txt", filename=attachment)
mime.add_header("Content-Disposition", "attachment", filename=attachment)
mime.set_payload(f.read())
encoders.encode_base64(mime)
msg.attach(mime)
try:
smtp.sendmail("[email protected]", "[email protected]", msg.as_string())
smtp.quit()
except smtplib.SMTPException as e:
print(e)
if __name__ == "__main__":
# send_email("亲")
send_email_attach("亲", r"D:\文档\csdn.md")
带个html
def send_email_all(body, maintype="plain", attachment=None):
smtp = SMTP_SSL("smtp.qq.com") # 邮件服务器地址
smtp.login("[email protected]", get_password()) # 邮件账户密码
smtp.set_debuglevel(1)
msg = MIMEMultipart() # 构造一个MIMEMultipart对象代表邮件本身
msg["Subject"] = Header("欢迎您来到延瓒@Yankerp", "utf-8")
# plain代表纯文本
msg.attach(MIMEText(body, maintype, "utf-8"))
if attachment:
with open(attachment, "rb") as f:
mime = MIMEBase("text", "txt", filename=attachment)
mime.add_header("Content-Disposition", "attachment", filename=attachment)
mime.set_payload(f.read())
encoders.encode_base64(mime)
msg.attach(mime)
try:
smtp.sendmail("[email protected]", "[email protected]", msg.as_string())
smtp.quit()
except smtplib.SMTPException as e:
print(e)
if __name__ == "__main__":
send_email_all(html, "html", r"D:\文档\监控平台.txt")
"""
html = """
<h1>Welcome to YanZan@Yankerp</h1>
<h2>info</h2>
<table border="1">
<tr>
<th>姓名</th>
<th>城市</th>
</tr>
<tr>
<td>Yankerp</td>
<td>北京</td>
</tr>
</table>
扫描二维码关注公众号,回复:
4664281 查看本文章
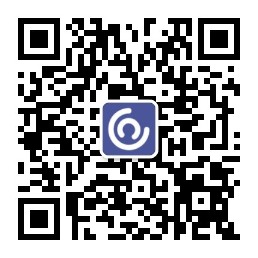
测试代码
#!/usr/bin/env python
# -*- coding:utf-8 -*-
import smtplib
from smtplib import SMTP_SSL
from email.header import Header
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.base import MIMEBase
from email import encoders
def get_password(): # 获取邮箱授权码
return "邮箱授权码"
def send_email(username):
smtp = SMTP_SSL("smtp.qq.com", "465") # 邮件服务器地址
smtp.login("[email protected]", get_password()) # 邮件账户密码
text = f"{username}: \n 欢迎来到'延瓒@Yankerp'CSDN博客站点..."
msg = MIMEText(text, "plain", "utf-8")
msg["Subject"] = Header("欢迎您来到延瓒@Yankerp", "utf-8") # 邮件的标题.
# msg["from"] = "[email protected]"
# msg["to"] = "[email protected]"
smtp.sendmail("[email protected]", "[email protected]", msg.as_string())
smtp.quit()
def send_email_attach(body, attachment):
smtp = SMTP_SSL("smtp.qq.com") # 邮件服务器地址
smtp.login("[email protected]", get_password()) # 邮件账户密码
msg = MIMEMultipart() # 构造一个MIMEMultipart对象代表邮件本身
msg["Subject"] = Header("欢迎您来到延瓒@Yankerp", "utf-8")
# plain代表纯文本
msg.attach(MIMEText(body, "plain", "utf-8"))
with open(attachment, "rb") as f:
mime = MIMEBase("text", "txt", filename=attachment)
mime.add_header("Content-Disposition", "attachment", filename=attachment)
mime.set_payload(f.read())
encoders.encode_base64(mime)
msg.attach(mime)
try:
smtp.sendmail("[email protected]", "[email protected]", msg.as_string())
smtp.quit()
except smtplib.SMTPException as e:
print(e)
def send_email_all(body, maintype="plain", attachment=None):
smtp = SMTP_SSL("smtp.qq.com") # 邮件服务器地址
smtp.login("[email protected]", get_password()) # 邮件账户密码
smtp.set_debuglevel(1)
msg = MIMEMultipart() # 构造一个MIMEMultipart对象代表邮件本身
msg["Subject"] = Header("欢迎您来到延瓒@Yankerp", "utf-8")
# plain代表纯文本
msg.attach(MIMEText(body, maintype, "utf-8"))
if attachment:
with open(attachment, "rb") as f:
mime = MIMEBase("text", "txt", filename=attachment)
mime.add_header("Content-Disposition", "attachment", filename=attachment)
mime.set_payload(f.read())
encoders.encode_base64(mime)
msg.attach(mime)
try:
smtp.sendmail("[email protected]", "[email protected]", msg.as_string())
smtp.quit()
except smtplib.SMTPException as e:
print(e)
html = """
<h1>Welcome to YanZan@Yankerp</h1>
<h2>info</h2>
<table border="1">
<tr>
<th>姓名</th>
<th>城市</th>
</tr>
<tr>
<td>Yankerp</td>
<td>北京</td>
</tr>
</table>
"""
if __name__ == "__main__":
# send_email("亲")
# send_email_attach("亲", r"D:\文档\csdn.md")
send_email_all(html, "html", r"D:\文档\监控平台.txt")