https://leetcode.com/problems/hand-of-straights/
Alice has a hand
of cards, given as an array of integers.
Now she wants to rearrange the cards into groups so that each group is size W
, and consists of W
consecutive cards.
Return true
if and only if she can.
Example 1:
Input: hand = [1,2,3,6,2,3,4,7,8], W = 3 Output: true Explanation: Alice'shand
can be rearranged as[1,2,3],[2,3,4],[6,7,8]
.
Example 2:
Input: hand = [1,2,3,4,5], W = 4 Output: false Explanation: Alice'shand
can't be rearranged into groups of4
.
Note:
1 <= hand.length <= 10000
0 <= hand[i] <= 10^9
1 <= W <= hand.length
代码:
扫描二维码关注公众号,回复:
4604952 查看本文章
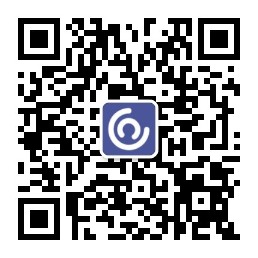

class Solution { public: bool isNStraightHand(vector<int>& hand, int W) { int n = hand.size(); map<int, int> mp; if(n % W) return false; if(W == 1) return true; sort(hand.begin(), hand.end()); for(int i = 0; i < n; i ++) mp[hand[i]] ++; int ans = 0; for(int i = 0; i < n - 1; i ++) { if(mp[hand[i]]) { int temp = hand[i]; mp[temp] --; int cnt = 1; for(int j = i + 1; j < n; j ++) { if(hand[j] - temp == 1 && mp[hand[j]] && cnt < W) { mp[hand[j]] --; cnt ++; temp = hand[j]; } } if(cnt == W) ans ++; } } if(ans == n / W) return true; return false; } };
我笨拙的 code 这个是在 map 专题里面的(因为看到 map 这个 tag 里面题目只有两个!激起了刷掉的欲望!) 一定是我还没透彻理解 map 今天杭州和苏州都在下雨 今天感冒终于见好转 等 FH 忙完再找 debug 吧!