1.map
map()函数接收两个参数,一个是函数,一个是序列
map将传入的函数依次作用到序列的每个元素,并且把结果
作为新的序列返回
#求绝对值:
print((map(abs,[-1,3,-4,-5])))
输出:
<map object at 0x7f2aef1e5208>、
map打印的不是一个列表而是一个对象,所以我们需要转换为列表才能打印。
print(list(map(abs,[-1,3,-4,-5])))
输出:
[1, 3, 4, 5]
# 需求:用户接收一串数字;讲该字符串中的所有数字转化为整型
# 并以列表格式输出
num = input('')
def isint(num):
return int(float(num))
print(list(map(isint,num.split(' '))))
# 对于序列的每个元素求阶乘
def factoria(x):
"""对与x求阶乘"""
res = 1
for i in range(1,x+1):
res = res * i
return res
li = [random.randint(2,7) for i in range(10)]
print(list(map(factoria,li)))
输出:
[24, 5040, 2, 2, 24, 120, 2, 24, 720, 5040]
2.reduce
reduce:把一个函数作用在一个序列上,这个函数必须接收两个参数
reduce把结果继续和序列的下一个元素做累积计算
reduce(f,[x1,x2,x3,x4]) = f(f(f(x1,x2),x3),x4)
例1:3的阶乘
from functools import reduce
"""
python2:reduce为内置函数
python3:from functools import reduce,这里我用的python3
"""
def multi(x,y):
return x*y
print(reduce(multi,range(1,4)))
输出:
6
例2:求1-5的和
def add(x,y):
return x+y
print(reduce(add,[1,2,3,4,5]))
输出:
15
3.filter 过滤函数
和map()类似,filter()也接收一个函数和一个序列
但是和map()不同的是,filter()把传入的函数依次作用于每个元素,
然后根据返回值是True还是False决定保留还是丢弃该元素.
例1:100以内的偶数
#只能一个形参,函数的返回值只能是True或者False
def isodd(num):
if num %2==0:
return True
else:
return False
print(list(filter(isodd,range(100))))
4.sorted
.sort()与sorted()的区别:
li = [1,2,4,6,3]
li.sort() # sort在原有列表中排序
print(li)
a = sorted(li) # sorted是给新生成变量排序
print(a)
#默认sort和sorted方法由小到大进行排序,reverse=True 由大到小进行排序
a = sorted(li,reverse=True)
print(a)
这里sorted()是一个高阶函数,我们来了解一下他的用法:
info = [
# 商品名称 商品数量 商品价格
['apple1', 200, 32],
['apple4', 40, 12],
['apple3', 40, 2],
['apple2', 1000, 23]
]
#默认按照商品数量进行排序
print(sorted(info))
输出:
[['apple1', 200, 32], ['apple2', 1000, 23], ['apple3', 40, 2], ['apple4', 40, 12]]
怎么样按照商品数量和商品价格排序呢?
1.商品数量
info = [
# 商品名称 商品数量 商品价格
['apple1', 200, 32],
['apple4', 40, 12],
['apple3', 40, 2],
['apple2', 1000, 23]
]
def sorted_by_count(x):
return x[1]
print(sorted(info,key=sorted_by_count)) # key代表排序的关键字
输出:
[['apple4', 40, 12], ['apple3', 40, 2], ['apple1', 200, 32], ['apple2', 1000, 23]]
2.商品价格
info = [
# 商品名称 商品数量 商品价格
['apple1', 200, 32],
['apple4', 40, 12],
['apple3', 40, 2],
['apple2', 1000, 23]
]
# 按照商品价格进行排序
def sorted_by_price(x):
return x[2]
print(sorted(info,key=sorted_by_price)) # key代表排序的关键字
输出:
[['apple3', 40, 2], ['apple4', 40, 12], ['apple2', 1000, 23], ['apple1', 200, 32]]
如果商品数量一致时候怎么办?如果商品数量一致,则按照商品价格由小到大进行排序。
扫描二维码关注公众号,回复:
4592906 查看本文章
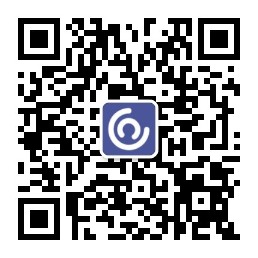
#先按照商品数量由小到大进行排序,如果商品数量一致,
#则按照商品价格由小到大进行排序
def sorted_by_count_price(x):
return x[1], x[2]
print(sorted(info, key=sorted_by_count_price)) # key代表排序的关键字