使用总结:
1. IOC容器:
定义:
控制反转(IoC)也称为依赖注入(DI)。这是一个过程:通过这个过程,对象只能通过构造函数参数,工厂方法的参数或从工厂方法构造或返回对象实例之后在对象实例上设置的属性来定义它们的依赖关系;然后通过创建bean时注入这些依赖项。这个过程本质上是bean本身的逆过程(因此称为控制反转(IOC)),它通过直接构造类或诸如Service Locator模式之类的机制来控制依赖项的实例化或位置。
bean是由ioc容器实例化,组装和管理的对象,BeanFactory提供了配置框架和基本功能
ApplicationContext(该接口表示ioc容器,负责实例化,配置和组装bean)添加了更多企业特定的功能。ApplicationContext是BeanFactory的完整超集
配置:注解+xml+groovy
- 实例化容器:即创建ApplicationContext的实例
有很多种形式:各有其含义及使用场景,正常不需要手动创建
ClassPathXmlApplicationContext/GenericApplicationContext…
//直接配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="accountDao"
class="org.springframework.samples.jpetstore.dao.jpa.JpaAccountDao">
<!-- additional collaborators and configuration for this bean go here -->
</bean>
<bean id="itemDao" class="org.springframework.samples.jpetstore.dao.jpa.JpaItemDao">
<!-- additional collaborators and configuration for this bean go here -->
</bean>
<!-- more bean definitions for data access objects go here -->
</beans>
//配置基础上导入的
<beans>
<import resource="services.xml"/>
<import resource="resources/messageSource.xml"/>
<import resource="/resources/themeSource.xml"/>
<bean id="bean1" class="..."/>
<bean id="bean2" class="..."/>
</beans>
//------------------------------------------------
ApplicationContext context = new ClassPathXmlApplicationContext("services.xml", "daos.xml");
//groovy
beans {
dataSource(BasicDataSource) {
driverClassName = "org.hsqldb.jdbcDriver"
url = "jdbc:hsqldb:mem:grailsDB"
username = "sa"
password = ""
settings = [mynew:"setting"]
}
sessionFactory(SessionFactory) {
dataSource = dataSource
}
myService(MyService) {
nestedBean = { AnotherBean bean ->
dataSource = dataSource
}
}
}
//------------------------------------------------
ApplicationContext context = new GenericGroovyApplicationContext("services.groovy", "daos.groovy");
bean的功能定义
属性 | 含义 |
---|---|
Class | 实例类名 |
Name | bean的别名,多个使用逗号分隔 |
Scope | 生命周期 |
Constructor arguments | 构造参数 |
Properties | 属性,依赖关系 |
Autowiring mode | 自动装配 |
Lazy initialization mode | 懒加载 |
Initialization method | 初始化方法 |
Destruction method | 销毁方法 |
bean的属性
属性 | 含义 |
---|---|
id | beanid |
name | bean别名,多个用逗号分隔;也可以使用标签定义 |
class | 类名;如果是内部类com.example.SomeThing$OtherThing |
factory-method | 静态工厂方式实例化;使用这个指定的方法创建实例 |
factory-bean | 实例工厂实例化,使用这个bean对应的方法创建实例;与factory-method连用 |
P标签/C标签 | 实现property的功能,优势:可以在编译期发现错误 |
depends-on | 弱引用关联,表示加载当前bean之前,先加载这个指定的bean;多个可用逗号分隔 |
lazy-init | 懒加载:第一次请求时创建bean实例,而不是启动时 |
autowire | 自动装配 no/byName/byType/constructor 根据属性名/属性类型/构造方法参数 自动匹配对应的bean实例 |
Scopes | singleton/prototype/request/session/application/websocket/自定义 |
init-method | 初始化方法: |
destroy-method | 销毁方法,bean销毁,容器重启 |
parent | 定义继承 |
abstract | 是否抽象 |
primary | 自动装配时的优先bean;对应注解对应 |
bean的内部标签
属性 | 含义 |
---|---|
constructor-arg | 构造注入参数 |
property(的ref/value/bean) | setter注入 |
lookup-method | 方法注入:比如像抽象类的抽象方法注入一个实例,使用这个抽象类调用方法时会使用这个实例的方法 |
replaced-method | 方法替换:使用指定方法替换当前方法 |
qualifier | 对应注解对应 |
property的内部标签,可注入的内容
bean | ref | idref | list | set | map | props | value | null
此外还有复合属性<property name="fred.bob.sammy" value="123" />
beans的属性
属性 | 含义 |
---|---|
default-lazy-init | 全局定义懒加载开关 |
default-init-method | 全局定义默认初始化方法 |
default-destroy-method | 全局定义默认销毁方法 |
bean的实例化:
- 使用构造方法实例化(默认)
- 使用静态工厂方法实例化factory-method:使用当前这个bean的指定的这个方法创建实例(同一个类)
- 使用实例工厂实例化factory-bean,factory-method 与2类似:使用指定的这个bean的方法创建实例(不同的类)
bean的注入:
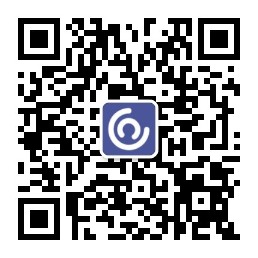
- 基于构造函数的依赖
- 基于setter的依赖注入
标签:
为占位符提供元素:
context:property-placeholder/
带覆盖的属性:
context:property-override/
容器启动加载注解:
context:annotation-config/
注解:
@ConstructorProperties:修饰构造方法,用于注入
@Lookup(“myCommand”):修饰未实现的方法,方法注入
@Required:修饰setter方法,如果注入的内容为空,容器报错,防止后续实例调用的空指针
@Autowired:修饰构造方法、setter方法、任意名称和多个参数的方法、字段、字段+构造方法组合,自动装配;如果自动装配失败会报错
@Autowired(required = false) 表示该属性为自动装配非必要属性,如果无法自动装配,则忽略属性
@Primary:由于按类型自动装配可能会导致多个候选人,因此通常需要对选择过程有更多控制权;该注解可以在自动转配的存在多个bean的候选时,指定一个优先
@Qualifier(“main”):修饰字段、方法参数,也可以作为元注解(自定义注解),需要更多的选择过程时使用;限定构造参数;除此之外可以使用java的泛型去做限定
@Resource:修饰字段、setter方法;@Resource(name=“myMovieFinder”)指定bean的名称注入
@PostConstruct和@PreDestroy:替换初始化回调及销毁回调方法
@Value:修饰方法参数,可使用占位符
@Scope:修饰类、@bean的方法,指定生命周期
@Bean:被用于指示一个方法实例、配置、初始化为一个spring ioc容器管理的对象;与bean标签配置一样,表示声明一个bean
@Bean(initMethod = "init")
@Bean(destroyMethod = "cleanup")
@Bean(name = "myThing")
@Bean({"dataSource", "subsystemA-dataSource", "subsystemB-dataSource"})//多个名字
@Description:描述
@Configuration:修饰类;表示其主要目的是作为bean定义的来源
@Configuration
public class AppConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
//与一下配置一样
<beans>
<bean id="myService" class="com.acme.services.MyServiceImpl"/>
</beans>
类路径扫描及托管组件:
@Component:是任何spring管理组件的通用构造型
@Repository,@Service和,@Controller是@Component更具体的用例的专业化(分别在持久性,服务和表示层)
@Service:修饰类,自动注册bean
@Repository:修饰类,自动注册bean
@Configuration:修饰configuration类
@ComponentScan(basePackages = “org.example”):修饰config类,检索的范围,有这两个注解修饰config类,才能扫描到@Service及@Repository的自动注册
xml的替代方法
<context:component-scan base-package="org.example"/>
与过滤器结合使用@Filter:
@ComponentScan(basePackages = “org.example”,
includeFilters = @Filter(type = FilterType.REGEX, pattern = “.*Stub.*Repository”),
excludeFilters = @Filter(Repository.class))
spring支持jsr330的标准注释
@Import:允许@Bean从另一个配置类加载定义
@ImportResource:修饰类,加载资源文件
@Profile:可以指示当一个或多个指定的配置文件处于活动状态时指定一个
@Profile(“default”)默认配置
@PropertySource:提供便利和声明的机制添加PropertySource 到Spring的Environment。@PropertySource(“classpath:/com/myco/app.properties”)
@EnableLoadTimeWeaving:
@EventListener:事件监听器
@Order:指定顺序
接口:
org.springframework.beans.factory.InitializingBean:初始化方法
org.springframework.beans.factory.DisposableBean:销毁方法
Lifecycle、LifecycleProcessor 为任何具有自己的生命周期要求的对象(例如启动和停止某些后台进程)定义了基本方法
org.springframework.context.ApplicationContextAware、
org.springframework.beans.factory.BeanNameAware、其他Aware接口:可以拿到context上下文对象并处理,不同的接口提供不同的功能
org.springframework.beans.factory.config.BeanPostProcessor:定义了您可以实现的回调方法,以提供您自己的(或覆盖容器的默认)实例化逻辑,依赖关系解析逻辑等
org.springframework.beans.factory.config.BeanFactoryPostProcessor:与BeanPostProcessor相似,唯一区别: 其对bean配置元数据进行操作;Spring IoC容器允许BeanFactoryPostProcessor读取配置元数据并可能在容器实例化除实例之外的任何bean 之前更改它
org.springframework.beans.factory.FactoryBean:自定义实例化逻辑
CustomAutowireConfigurer:自定义限定符注释类型
AnnotationConfigApplicationContext:实例化容器,提供注册class等方法;
setActiveProfiles激活个人文件
AnnotationConfigWebApplicationContext:典型的springmvc web监听器使用
LoadTimeWeaver:
MessageSource:提供国际化i18n功能;可以选择不同的配置文件
Event:事件接口
注解与接口的功能可能不限于此,个人整理,欢迎批评指正