IOC容器本质:
Ioc本质上是一个对象容器,这里称为bean容器,你可以在程序一开启的时候就将
程序中可能用到的所有对象全部放入Ioc容器,当用某个对象的时候再从容器里面拿。
这种对象预加载的操作可以大大提高程序的运行速度,因为你在程序在运行中不需要
花时间去创建对象,而是直接去Ioc容器里面拿就行了。本质是将创建对象的时间费用
转移到了开启程序的一瞬间。
如上所描述,可知Ioc基本的使用就在于
如何向Ioc内注入对象
如何从Ioc内拿出对象
扫描二维码关注公众号,回复:
14808190 查看本文章
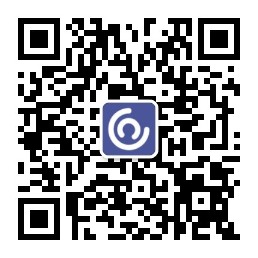
Ioc的使用的基本步骤如下:
导入Maven依赖
在maven项目下的Resources中创建spring的applicationContext.xml配置文件
在main程序中通过ClassPathXmlApplicationContext对象读取spring配置文件
使用DI(基于xml/基于注解)向spring容器中注入bean
Maven依赖
spring常用maven依赖如下
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-expression</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.42</version>
<scope>runtime</scope>
</dependency>
</dependencies>
Spring容器的Bean配置文件
建议文件名:applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<!--①使用spring约束-->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
<!-- 2.使用基于注解的包扫描器-->
<context:component-scan base-package=""/>
</beans>
应用程序读取Spring配置文件
ApplicationContext ioc=new
ClassPathXmlApplicationContext("applicationContext.xml");
返回的ioc即为ioc容器,通过它可以从容器中获取bean
DI( 基于xml的)
通过xml配置文件向ioc容器注入bean
<?xml version="1.0" encoding="UTF-8"?>
<!--使用spring约束-->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
<!-- 2.使用基于注解的包扫描器-->
<!-- <context:component-scan base-package="com.itheima"/>-->
<bean id="user" class="com.itheima.entity.User" scope="singleton">
<!-- 基于类的属性进行注入(需要类中有对应属性的GetSet方法)-->
<property name="id" value="22"/>
<property name="name" value="小米1"/>
<property name="password" value="6666"/>
</bean>
<bean id="manager" class="com.itheima.entity.Manager" scope="singleton">
<!-- 基于类的构造器进行注入(需要类中有对应属性的构造器)-->
<constructor-arg name="id" value="22"/>
<constructor-arg name="name" value="小米2"/>
</bean>
</beans>
DL(基于注解的)
通过注解向ioc容器注入bean
<?xml version="1.0" encoding="UTF-8"?>
<!--使用spring约束-->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
<!-- 设置扫描注解的包扫描器-->
<context:component-scan base-package="com.itheima"/>
</beans>
其他提示
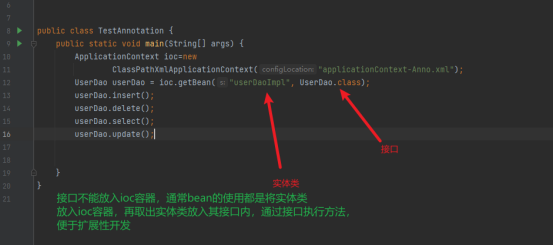