文章目录
10-1 Python学习笔记
在文本编辑器中新建一个文件,写几句话来总结一下你至此学到的Python知识,其中每一行都以“In Python you can” 打头。将这个文件命名为learning_python.txt,并将其存储到为完成本章练习而编写的程序所在的目录中。编写一个程序,它读取这个文件,并将你所写的内容打印三次:第一次打印时读取整个文件;第二次打印时遍历文件对象;第三次打印时将各行存储在一个列表中,再在with代码块打印它们。
(1)打印时读取整个文件
file_name = "py_learning.txt"
with open(file_name) as file_object:
lines = file_object.read()
print(lines.rstrip())
小结:
- 方法read()读取文件的全部内容。
- 方法rstrip()删除末尾的空格。
- 方法strip()删除两端的空格。
△(2)打印时遍历文件对象。
file_name = "learning_python.txt"
with open(file_name) as file_object:
for line in file_object:
print(line.strip())
展示一种错误写法
在写这段的时候写成:
lines = file_object.read()
for line in lines:
print(line.strip())
要多加注意。
(3)将各行存储在一个列表中,再在with代码块外打印它们
file_name = "learning_python.txt"
with open(file_name) as file_object:
lines = file_object.readlines()
for line in lines:
print(line.strip())
上述输出结果均为:
In Python you can establish dictionary.
In Python you can establish list.
In Python you can use while, for and if to do logical judgment.
In Python you can use function to solve problems.
10-2 C语言学习笔记
可用方法replace()将字符串中的特定单词都替换为另一个单词。下面是一个简单的示例,演示了如何将句子中的‘dog’替换为‘cat’:
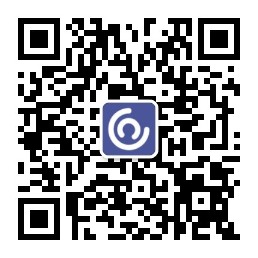
>>> message = "I really like dogs."
>>> message.replace('dog', 'cat')
'I really like cats.'
读取你刚创建的文件learning_python.txt中的每一行,将其中的Python都替换为另一门语言的名称,如C。将修改后的各行都打印到屏幕上。
file_name = "learning_python.txt"
with open(file_name) as file_objects:
contents = file_objects.read()
contents_change = contents.replace('Python', 'C')
print(contents_change)
build
In C you can establish dictionary.
In C you can establish list.
In C you can use while, for and if to do logical judgment.
In C you can use function to solve problems.
10-3 访客
编写一个程序,提示用户输入其名字。用户作出响应后,将其名字写入到文件guest.txt中。
filename = "guest.txt"
username = input("Please input your name:")
with open(filename, 'w') as file_object:
file_object.write(username)
Tools—SublimeREPL—Python—Python-runcurrentfile
Please input your name:
输入 “Yan Junze”
Please input your name:Yan Junze
***Repl Closed***
打开与该Python程序同一个文件夹的guest.txt
该字符串被写入文件。
10-4 访客名单
编写一个while循环,提示用户输入其名字。用户输入名字后,在屏幕上打印一条问候语,并将一条访问记录添加到文件guest_book.txt中。确保这个文件中的每条记录都独占一行。
filename = "guest_book.txt"
print("Enter 'quit' when you are finished.\n")
active = True
while active:
username = input("What's your name? ")
if username == "quit":
active = False
else:
with open(filename, 'a') as file_object:
file_object.write(username.title() + " has visited the website.\n")
print(username.title() + ", welcome to our website.")
Tools—SublimeREPL—Python—Python-runcurrentfile
Enter 'quit' when you are finished.
What's your name? yan junze
Yan Junze, welcome to our website.
What's your name? liu shiyu
Liu Shiyu, welcome to our website.
What's your name? quit
***Repl Closed***
txt的写入结果如下图。
(这道题参考了答案)
原本的写法也写得不好。while循环使用得很草率,没有利用逻辑值的赋值。
下面是错误示范
filename = "guest_book.txt"
active = True
while active:
username = input("Please input your name:")
with open(filename, 'a') as file_object:
file_object.write(username)
print("Welcome to the Website, " + username.title())
username = input("Please input your name:")
但虽然写得不对,也不明白为什么不对。
10-5 关于编程的调查
编写一个while循环,询问用户为何喜欢编程。每当用户输入一个原因后,都将其添加到一个存储所有原因的文件中。
这题的做法基本和10-4没有区别
filename = "love_programming_reason.txt"
print("Enter 'quit' when you finished.\n")
active = True
while active:
reason = input("Why do you love programming?\n")
if reason == 'quit':
active = False
else:
with open(filename, 'a') as file_object:
file_object.write(reason)
print("Thank you!")
Tools—SublimeREPL—Python—Python-runcurrentfile
Enter 'quit' when you finished.
Why do you love programming?
it's interesting
Thank you!
Why do you love programming?
it's cool
Thank you!
Why do you love programming?
I enjoy it
Thank you!
Why do you love programming?
quit
***Repl Closed***
存储原因的文件:
10-6 加法运算
提示用户提供数值输入时,常出现的一个问题是,用户提供的是文本而不是数字。在这种情况下,当你尝试将输入转换为整数时,将引发ValueError异常。编写一个程序,提示用户输入两个数字,再将它们相加并打印结果。在用户输入的任何一个值不是数字时都捕获ValueError异常,并打印一条友好的错误消息。对你编写的程序进行测试:先输入两个数字,再输入一些文本而不是数字。
num2 = input("Please input the second number:")
try:
num3 = int(num1) + int(num2)
except ValueError:
print("Sorry, you need to input number instead of words.")
else:
print("The sum of these two numbers is " + str(num3))
Build—Tools—SublimeREPL—Python—Python-runcurrentfile
输入数字:
Please input the first number:5
Please input the second number:7
The sum of these two numbers is 12
***Repl Closed***
输入文本:
Please input the first number:5
Please input the second number:hello
Sorry, you need to input number instead of words.
***Repl Closed***
10-7 加法计算器
将你为完成练习10-6而编写的代码放在一个while循环中,让用户犯错(输入的是文本而不是数字)后能继续输入数字。
active = True
print("Enter 'q' when you want to quit.\n")
while active:
num1 = input("Please input the first number:")
if num1 == 'q':
active = False #False要大写
else:
num2 = input("Please input the second number:")
if num2 =='q':
active = False
else:
try:
num3 = int(num1) + int(num2)
except ValueError:
print("Sorry, you need to input number instead of words.\n")
else:
print("The sum of these two numbers is " + str(num3) + ".\n")
Tools—SublimeREPL—Python—Python-runcurrentfile
Enter 'q' when you want to quit.
Please input the first number:hello
Please input the second number:3
Sorry, you need to input number instead of words.
Please input the first number:byebye
Please input the second number:5
Sorry, you need to input number instead of words.
Please input the first number:5
Please input the second number:6
The sum of these two numbers is 11.
Please input the first number:q
***Repl Closed***
10-8 猫和狗
创建两个文件cats.txt和dogs.txt,在第一个文件中至少存储三只猫的名字,在第二个文件中至少存储三条狗的名字。编写一个程序,尝试读取这些文件,并将其内容打印到屏幕上。将这些代码放在一个try-except代码块中,以便在文件不存在时捕获FileNotFound错误,并打印一条友好的消息。将其中一个文件迁移到另一个地方,并确认except代码块中的代码将正确地执行。
def print_out(filename):
"""定义一个打印文件内容的函数"""
try:
with open(filename) as objects:
contents = objects.read()
except FileNotFoundError:
print("Sorry," + filename + "does not exist.")
else:
print(contents + "\n")
filenames = ['cats.txt', 'dogs.txt']
for filename1 in filenames:
print_out(filename1)
build
shagou
jiaozi
watermelon
lingling
Sorry,dogs.txtdoes not exist.
10-9 沉默的猫和狗
修改你在练习10-8中编写的except代码块,让程序在文件不存在时一言不发。
pass语句的使用和作用
def print_out(filename):
"""定义一个打印文件内容的函数"""
try:
with open(filename) as objects:
contents = objects.read()
except FileNotFoundError:
pass
else:
print(contents + "\n")
filenames = ['cats.txt', 'dogs.txt']
for filename1 in filenames:
print_out(filename1)
build
shagou
jiaozi
watermelon
lingling
10-10 常见单词
访问项目Gutenberg,并找一些你想分析的图书。下载这些作品的文本文件或将浏览器中的原始文本复制到文本文件中。
你可以使用方法count()来确定特定的单词或短语在字符串中出现了多少次。例如,下面的代码计算‘row’在一个字符串中出现了多少次:
>>> line = "Row, row, row your boat"
>>> line.count('row')
2
>>> line.lower().count('row')
3
请注意,通过使用lower()将字符串转换为小写,可捕捉要查找的单词出现的所有次数,而不管其大小写格式如何。
编写一个程序,它读取你在项目Gutenberg中获取的文件,并计算单词‘the’在每个文件中分别出现了多少次。
def count_words(filename, word_to_count):
"""计算文档中任意单词的数目"""
try:
with open(filename) as objects:
contents = objects.read()
except FileNotFoundError:
print("Sorry," + filename + " cannot be found.")
else:
contents1 = contents.lower()
number=contents.lower().count(word_to_count)
print("The number of the word '" + word_to_count + "' in" + filename
+ " is " + str(number))
filenames = ['grania.txt', 'Colored Troops in the French Army.txt']
for filename1 in filenames:
count_words(filename1, 'the')
build
The number of the word 'the' ingrania.txt is 3775
Sorry,Colored Troops in the French Army.txt cannot be found.
两个问题
问题1
UnicodeDecodeError: 'gbk' codec can't decode byte 0x99 in position 1524: illegal multibyte sequence
使用notepad.exe小程序改变编码类型。
==①当txt文件编码为ANSI时,如上述可顺利运行 ==
②当txt文件编码为UTF-8时,第四行改为
with open(filename, 'r', encoding='UTF-8') as objects:
Unicode和Unicode big endian的情况有待探究
问题2
当第四行改为:
with open(filename, 'rb') as objects:
出现
TypeError: argument should be integer or bytes-like object, not 'str'
‘rb’表示读写二进制数据(存疑?)
需要补充一下编码知识
10-11 喜欢的数字
编写一个程序,提示用户输入他喜欢的数字,并使用json.dump()将这个数字存储到文件中。再编写一个程序,从文件中读取这个值,并打印消息“I know your favorite number! It’s___. ”
(1)用json.dump()将数字存储到文件中。
import json
filename = "favorite_number.json"
number = input("What's your favorite number?")
with open(filename, 'w') as f_obj:
json.dump(number, f_obj)
print("We'll remember your favorite number.")
Build—Tools—SublimeREPL—Python—Python-runcurrentfile
What's your favorite number?15
We'll remember your favorite number.
(2)从文件中读取这个值
import json
filename = "favorite_number.json"
with open(filename) as f_obj:
number = json.load(f_obj)
print("Your favorite number is " + number + ".")
build
Your favorite number is 15.
10-12 记住喜欢的数字
将练习10-11中的两个程序合而为一。如果存储了用户喜欢的数字,就向用户显示它,否则提示用户输入他喜欢的数字并将其存储到文件中。运行这个程序两次,看看它是否像预期的那样工作。
import json
filename = "favorite_number.json"
try:
with open(filename) as f_obj:
number = json.load(f_obj)
except FileNotFoundError:
number = input("What's your favorite number?")
with open(filename, 'w') as f_obj:
json.dump(number, f_obj)
print("We'll remember your favorite number.\n")
else:
print("Your favorite number is " + number + ".")
第一次:
Build—Tools—SublimeREPL—Python—Python-runcurrentfile
What's your favorite number? 15
We'll remember your favorite number.
***Repl Closed***
第二次:在已经输入favorite number的情况下。build。
Your favorite number is 15.
程序能够在两种情况下顺利运行。
10-13 验证用户
最后一个remember_me.py版本假设用户要么已输入其用户名,要么是首次运行该程序。我们应修改这个程序,以应对这样的情形:当前和最后一次运行该程序的用户并非同一个人。
为此,在greet_user()中打印欢迎用户回来的消息前,先询问他用户名是否是对的。如果不对,就调用get_new_username()让用户输入正确的用户名。
import json
def get_stored_username():
"""如果存储了用户名,就获取它"""
filename = 'username.json'
try:
with open(filename) as f_obj:
username = json.load(f_obj)
except FileNotFoundError:
return None
else:
return username
def get_new_username():
"""提示用户输入用户名"""
username = input("What is your name? ")
filename = 'username.json'
with open(filename, 'w') as f_obj:
json.dump(username, f_obj)
return username
def greet_new_user():
username = get_new_username()
print("We'll remember when you come back " + username + "!")
def greet_user():
"""问候用户,并指出其名字"""
username = get_stored_username()
if username:
correct = input("Is '" + username + "' your name? Input 'N' if it is not your name.\n"+
"And input anything else if it is your name.\n" )
correct1 = correct.upper()
if correct1 == 'N':
greet_new_user()
else:
print("Welcome back, " + username + "!")
else:
greet_new_user()
greet_user()
Build—Tools—SublimeREPL—Python—Python-runcurrentfile
①事先没有该file的情况。
What is your name? Wang
We'll remember when you come back Wang!
***Repl Closed***
②存在该file的情况:
(a)当用户变更,用户名不正确时。
Is 'Wang' your name? Input 'N' if it is not your name.
And input anything else if it is your name.
N
What is your name? Eric
We'll remember when you come back Eric!
***Repl Closed***
(b)当用户未变,用户名正确时。
Is 'Eric' your name? Input 'N' if it is not your name.
And input anything else if it is your name.
your
Welcome back, Eric!
***Repl Closed***
注意
Advantage: 对greet_new_user()部分进行了重构,建立一个新的函数。使无论是第一次运行程序还是更换用户,输入名字时均能收到问候。
Disadvantage: greet_user()函数中,if语句的嵌套问题;‘N’对应否,而任意其他键对应‘Yes’,是否不合理?需要思考。
总结
- 例题&习题
- 幕布
- 笔记
- 二刷