一、使用Spring框架中的MultipartFile实现后台代码逻辑处理
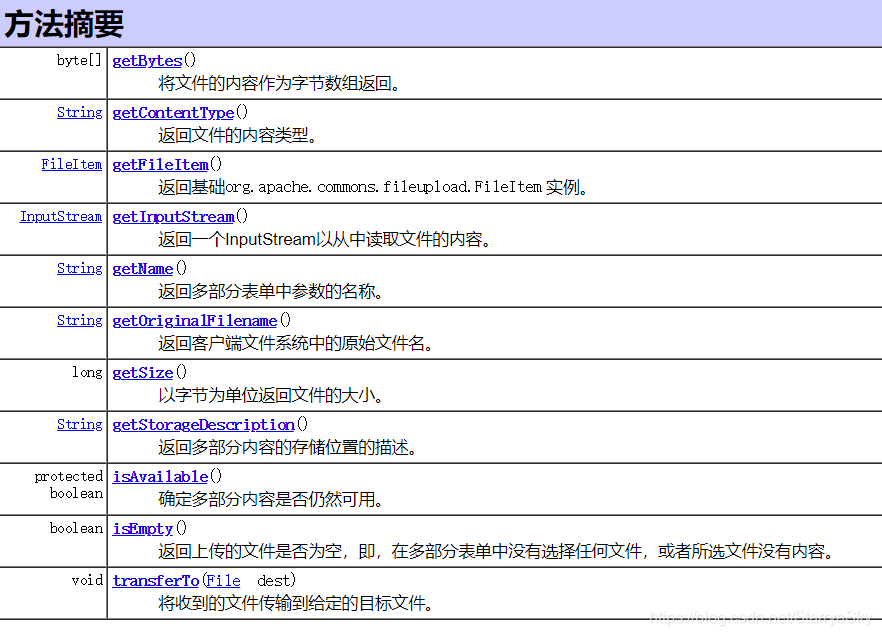
2. 后台逻辑代码
@Controller
public class FileUploadController {
@ResponseBody
@RequestMapping(value="/fileUpload",method=RequestMethod.POST)
public String fileUpload(@RequestParam("file")MultipartFile multFile,HttpServletRequest request) {
String multFileName = multFile.getOriginalFilename();
System.out.println("文件名为:"+multFileName);
long size = multFile.getSize();
System.out.println("文件大小:"+size);
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd-HH-mm-ss-SS");
String times = simpleDateFormat.format(new Date());
String suffix = multFileName.substring(multFileName.lastIndexOf("."));
System.out.println("文件后缀名"+suffix);
try {
InputStream inputStream = multFile.getInputStream();
InputStreamReader inputStreamReader = new InputStreamReader(inputStream,"GBK");
char[] chars = new char[1024];
int len = inputStreamReader.read(chars);
String context = new String(chars,0,len);
System.out.println("文本内容是:"+context);
} catch (IOException e1) {
e1.printStackTrace();
}
String path = "E:/FileUpload";
File newFile = new File(path+"/"+times+suffix);
if(!newFile.getParentFile().exists()) {
newFile.getParentFile().mkdirs();
}
try {
multFile.transferTo(newFile);
} catch (IllegalStateException | IOException e) {
e.printStackTrace();
}
return "上传成功";
}
@RequestMapping("/")
public String toIndex() {
return "index";
}
}
二、前端代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>文件上传下载测试</title>
<script src="${request.contextPath}/js/jquery-1.12.4.min.js"></script>
</head>
<body>
<div style="width: 100%;margin: 0 20% ;display: flex; flex-direction: column; width: 200px; ">
<h1>文件上传</h1>
<form id="uploadForm" action="${request.contextPath}/fileUpload" method="POST" enctype="multipart/form-data">
<input id="file" type="file" name="file"/>
<input type="text" name="text1" />
<input type="text" name="text2" />
<div style="margin-top: 10px;">
<input type="submit" value="直接上传"/>
<button type="button" id="ajaxBtn">ajax后台上传</button>
</div>
</form>
<h1>文件下载</h1>
<script type="text/javascript">
$("#ajaxBtn").on('click',function(){
console.log("ajaj异步文件上传开始---");
var formData = new FormData($("#uploadForm")[0]);
$.ajax({
url:'${request.contextPath}/fileUpload' ,
type:'POST',
data:formData ,
cache: false ,
contentType:false ,
processData:false ,
success:function(data){
console.log("ajax返回值"+data);
}
});
});
</script>
</div>
</body>
</html>
三、打印结果
1. eclipse控制台打印
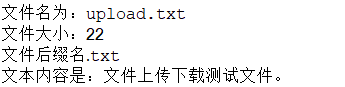
2. 浏览器后台打印
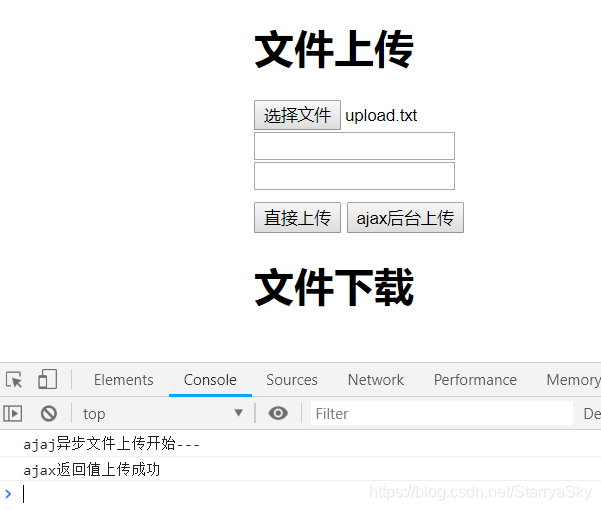