一、实验目的
1.掌握栈的顺序存储结构和链式存储结构;
2..验证顺序栈以及链栈及其基本操作的实现;
3验证栈的操作特性。
二、实验内容
1.建立一个空栈;
2.对已建立的栈进行插入、删除、去栈顶元素等基本操作。
三、设计与编码
1.本实验用到的理论知识。
涉及了栈的后进先出的特性,栈的顺序存储结构—顺序栈和栈的链式存储结构—链栈。
2.算法设计
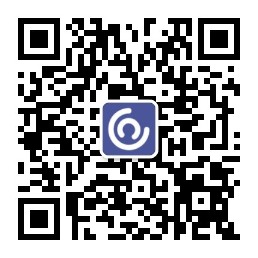
(1)顺序栈验证实验:
SeqStack.h
#ifndef SeqStack_H
#define SeqStack_H
const int StackSize=10;
template<class DataType>
class SeqStack
{
public:
SeqStack();
~SeqStack(){};
voidPush(DataType x);
DataTypePop();
DataTypeGetTop();
intEmpty();
private:
DataTypedata[StackSize];
inttop;
};
#endif
SeqStack.cpp
#include"SeqStack.h"
template<class DataType>
SeqStack<DataType>::SeqStack()
{
top=-1;
}
template<class DataType>
voidSeqStack<DataType>::Push(DataType x)
{
if(top==StackSize-1)throw"上溢";
top++;
data[top]=x;
}
template<class DataType>
DataType SeqStack<DataType>::Pop()
{
DataTypex;
if(top==-1)throw"下溢";
x=data[top--];
returnx;
}
template<class DataType>
DataType SeqStack<DataType>::GetTop()
{
if(top!=-1)
returndata[top];
}
template<class DataType>
int SeqStack<DataType>::Empty()
{
if(top==-1)return1;
elsereturn 0;
}
#include<iostream>
using namespace std;
#include "SeqStack.cpp"
void main()
{
SeqStack<int>S;
if(S.Empty())
cout<<"栈已空"<<endl;
else
cout<<"栈非空"<<endl;
cout<<"对56,65,10执行入栈操作"<<endl;
S.Push(56);
S.Push(65);
S.Push(10);
cout<<"栈顶元素:"<<endl;
cout<<S.GetTop()<<"执行操作"<<endl;
cout<<"执行一次出栈操作并对69执行入栈"<<endl;
S.Pop();
S.Push(69);
cout<<"栈顶元素"<<endl;
cout<<S.GetTop()<<endl;
}
(2)链栈验证实验
#include<iostream.h>
struct Node
{
int data;
Node *next;
};
class LinkStack
{
public:
LinkStack() { top=NULL; }
~LinkStack();
void Push(int x);
int Pop();
int GetTop() { if(top!=NULL)return top->data; }
bool Empty()
{
if(top==NULL)return 1;
else return 0;
}
private:
Node *top;
};
LinkStack::~LinkStack()
{
Node *p;
while(top!=NULL)
{
p=top;
top=top->next;
delete p;
}
}
void LinkStack::Push(int x)
{
Node *s=new Node;
s->data=x;
s->next=top;
top=s;
}
int LinkStack::Pop()
{
Node *p;
int x;
if(top==NULL)cout<<"Stack is empty"<<endl;
else
{
x=top->data;
p=top;
top=p->next;
delete p;
return x;
}
}
int main()
{
LinkStack s;
cout<<"对56、69和94执行压栈操作"<<endl;
s.Push(56);
s.Push(69);
s.Push(94);
cout<<"栈顶元素为:"<<endl;
cout<<s.GetTop()<<endl;
cout<<"执行一次出栈操作"<<endl;
cout<<s.Pop()<<endl;
cout<<"栈顶元素为:"<<endl;
cout<<s.GetTop()<<endl;
return 0;
}
四、运行与测试
1.遇到的问题是结构性问题,通过百度以及查阅书本解决。
2.设计的测试数据及结果如上。