数据库类
conn.php
<?php
class opmysql{
private $host = 'localhost'; //服务器地址
private $name = 'root'; //登录账号
private $pwd = '123456'; //登录密码
private $dBase = 'db_reglog'; //数据库名称
private $conn = ''; //数据库链接资源
private $result = ''; //结果集
private $msg = ''; //返回结果
private $fields; //返回字段
private $fieldsNum = 0; //返回字段数
private $rowsNum = 0; //返回结果数
private $rowsRst = ''; //返回单条记录的字段数组
private $filesArray = array(); //返回字段数组
private $rowsArray = array(); //返回结果数组
//初始化类
function __construct($host='',$name='',$pwd='',$dBase=''){
if($host != '')
$this->host = $host;
if($name != '')
$this->name = $name;
if($pwd != '')
$this->pwd = $pwd;
if($dBase != '')
$this->dBase = $dBase;
$this->init_conn();
}
//链接数据库
function init_conn(){
$this->conn=@mysql_connect($this->host,$this->name,$this->pwd);
@mysql_select_db($this->dBase,$this->conn);
mysql_query("set names gb2312");
}
//查询结果
function mysql_query_rst($sql){
if($this->conn == ''){
$this->init_conn();
}
$this->result = @mysql_query($sql,$this->conn);
}
//取得字段数
function getFieldsNum($sql){
$this->mysql_query_rst($sql);
$this->fieldsNum = @mysql_num_fields($this->result);
}
//取得查询结果数
function getRowsNum($sql){
$this->mysql_query_rst($sql);
if(mysql_errno() == 0){
return @mysql_num_rows($this->result);
}else{
return '';
}
}
//取得记录数组(单条记录)
function getRowsRst($sql){
$this->mysql_query_rst($sql);
if(mysql_error() == 0){
$this->rowsRst = mysql_fetch_array($this->result,MYSQL_ASSOC);
return $this->rowsRst;
}else{
return '';
}
}
//取得记录数组(多条记录)
function getRowsArray($sql){
$this->mysql_query_rst($sql);
if(mysql_errno() == 0){
while($row = mysql_fetch_array($this->result,MYSQL_ASSOC)) {
$this->rowsArray[] = $row;
}
return $this->rowsArray;
}else{
return '';
}
}
//更新、删除、添加记录数
function uidRst($sql){
if($this->conn == ''){
$this->init_conn();
}
@mysql_query($sql);
$this->rowsNum = @mysql_affected_rows();
if(mysql_errno() == 0){
return $this->rowsNum;
}else{
return '';
}
}
//获取对应的字段值
function getFields($sql,$fields){
$this->mysql_query_rst($sql);
if(mysql_errno() == 0){
if(mysql_num_rows($this->result) > 0){
$tmpfld = @mysql_fetch_row($this->result);
$this->fields = $tmpfld[$fields];
}
return $this->fields;
}else{
return '';
}
}
//错误信息
function msg_error(){
if(mysql_errno() != 0) {
$this->msg = mysql_error();
}
return $this->msg;
}
//释放结果集
function close_rst(){
mysql_free_result($this->result);
$this->msg = '';
$this->fieldsNum = 0;
$this->rowsNum = 0;
$this->filesArray = '';
$this->rowsArray = '';
}
//关闭数据库
function close_conn(){
$this->close_rst();
mysql_close($this->conn);
$this->conn = '';
}
}
$conne = new opmysql();//新建数据连接源
?>
index.php
实现cookie自动登陆
<?php
session_start();
header('Content-Type:text/html;charset=gb2312');
if(!empty($_COOKIE['name']) and !is_null($_COOKIE['name'])){
$_SESSION['name'] = $_COOKIE['name'];
header('location:http://'.$_SERVER['SERVER_NAME'].dirname($_SERVER['SCRIPT_NAME']).'/main.php');
}else{
header('location:http://'.$_SERVER['SERVER_NAME'].dirname($_SERVER['SCRIPT_NAME']).'/login.php');
}
?>
main.php
登陆成功后反馈的信息
扫描二维码关注公众号,回复:
4359990 查看本文章
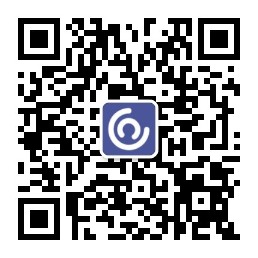
<?php
session_start();
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=gb2312" />
<title>登录成功</title>
</head>
<body>
<?php echo '欢迎光临!'.$_SESSION['name']; ?>
</body>
</html>
login.php
登陆界面
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=gb2312" />
<title>用户登录</title>
<link rel="stylesheet" type="text/css" href="css/style.css" />
<script language="javascript" src="js/login.js"></script>
<script language="javascript" src="js/xmlhttprequest.js"></script>
</head>
<body>
用户名<input id="lgname" name="lgname" type="text"/><br>
密 码<input id="lgpwd" name="lgpwd" type="password" /><br>
验证码<input id="lgchk" type="text" maxlength="4" style="width:35px;">
<img id='chkid' src=""/><a id="changea">看不清</a><br>
<button id="lgbtn" >登陆</button>
<button id="rgbtn">注册</button>
<button id="fdbtn">找回密码</button>
<input id="chknm" name="chknm" type="hidden" value="" />
</body>
</html>
Ajax初始化
xmlhttprequest.js
var xmlhttp = false;
if (window.XMLHttpRequest) { //Mozilla、Safari等浏览器
xmlhttp = new XMLHttpRequest();
}
else if (window.ActiveXObject) { //IE浏览器
try {
xmlhttp = new ActiveXObject("Msxml2.XMLHTTP");
} catch (e) {
try {
xmlhttp = new ActiveXObject("Microsoft.XMLHTTP");
} catch (e) {}
}
}
login.js
登陆界面JS
// JavaScript Document
function $(id){
return document.getElementById(id);
}
window.onload = function(){
showval();
$('lgname').focus();
$('lgname').onkeydown = function(){//按回车向下移动光标
if(event.keyCode == 13){
$('lgpwd').select();
}
}
$('lgpwd').onkeydown = function(){
if(event.keyCode == 13){
$('lgchk').select();
}
}
$('lgchk').onkeydown = function(){
if(event.keyCode == 13){
chklg();
}
}
$('lgbtn').onclick = chklg;
function chklg(){
if($('lgname').value.match(/^[a-zA-Z_]\w*$/) == null){
alert('请输入合法名称');
$('lgname').select();
return false;
}
if($('lgname').value == ''){
alert('请输入用户名!');
$('lgname').focus();
return false;
}
if($('lgpwd').value == ''){
alert('请输入密码!');
$('lgpwd').focus();
return false;
}
if($('lgchk').value == ''){
alert('请输入验证码');
$('lgchk').select();
return false;
}
if($('lgchk').value != $('chknm').value){
alert('验证码输入错误');
$('lgchk').select();
return false;
}
//防SQL注入式攻击(
count = document.cookie.split(';')[0];
if(count.split('=')[1] >= 3){
alert('因为您的非法操作,您将无法再执行登录操作');
return false;
}
//)
//$('regimg').style.visibility = "visible";//显示登陆的进度条
//Ajax发送与接受(
url = 'login_chk.php?act='+(Math.random())+'&name='+$('lgname').value+'&pwd='+$('lgpwd').value;
xmlhttp.open('get',url,true);
xmlhttp.onreadystatechange = function(){
if(xmlhttp.readyState == 4){
if(xmlhttp.status == 200){
msg = xmlhttp.responseText;
if(msg == '0'){
alert('您还没有激活,请先登录邮箱进行激活操作。');
}else if(msg == '1'){
alert('用户名或密码输入错误,您还有2次机会');
$('lgpwd').select();
}else if(msg == '2'){
alert('用户名或密码输入错误,您还有1次机会');
$('lgpwd').select();
}else if(msg == '3'){
alert('因为登录次数过多,您的帐号已被冻结,请联系管理员');
$('lgname').select();
}else if(msg == '4'){
alert('用户名输入错误');
$('lgname').select();
}else if(msg == '-1'){
alert('登录成功');
location = 'main.php';
}else{
alert(msg);
}
//$('regimg').style.visibility = "hidden";
}
}
}
xmlhttp.send(null);
}
//)
$('changea').onclick = showval;//刷新验证码
//生成验证码(
function showval(){
num = '';
for(i=0;i<4;i++){
tmp = Math.ceil((Math.random() * 15));
if(tmp > 9){
switch(tmp){
case(10):
num += 'a';
break;
case(11):
num += 'b';
break;
case(12):
num += 'c';
break;
case(13):
num += 'd';
break;
case(14):
num += 'e';
break;
case(15):
num += 'f';
break;
}
}else{
num += tmp;
}
}
$('chkid').src='valcode.php?num='+num;
$('chknm').value = num;
}
//)
$('fdbtn').onclick = function(){
fd = window.open('found.php','found','width=300,height=200');//在新窗口中打开
fd.moveTo(screen.width/2,200);
}
$('rgbtn').onclick = function(){
open('register.php','_parent','',false);//直接打开
}
}
login_chk.php
Ajax登陆后台验证
<?php
session_start();
header('Content-Type:text/html;charset=gb2312');
include_once 'conn/conn.php';
$name = addslashes($_GET['name']);
$pwd = $_GET['pwd'];
if(!empty($name) and !empty($pwd)){
$sql = "select name,count,active from tb_member where name = '".$name."'";
$active = $conne->getFields($sql,2);
$count = $conne->getFields($sql,1);
$conne->close_rst();
if($active == ''){
if(!isset($_COOKIE['count']) or $_COOKIE['count'] == 0){
setcookie('count',1);
}else{
setcookie('count',$_COOKIE['count']+1);
}
$reback = 4;
}else if($active == 0){//判断用户名是否被激活
$reback = '0';
}else if($count >= 3){//判断用户的登陆次数
$reback = '3';
}else{
$sql .= " and password = '".md5($pwd)."'";
$num = $conne->getRowsNum($sql);
if($num == 0 or $num == ''){//如果用户名密码错误,登录次数加1
$num = $conne->uidRst("update tb_member set count = ".($count+1)." where name = '".$name."'");
$reback = ($count+1);
}else{//登陆成功,清空count字段值
if($count != 0){
$num = $conne->uidRst("update tb_member set count = 0 where name = '".$name."'");
}
//设置COOKIE
if(isset($_COOKIE['count']) and $_COOKIE['count'] != 0){
setcookie('count',0);
}
setcookie('name',$name,time()+60*10);
$_SESSION['name'] = $name;
$reback = '-1';
}
}
}
echo $reback;
?>
生成验证码图片
valcode.php
<?php
//header("content-type:image/png");
$num = $_GET['num'];
$imagewidth=60;
$imageheight=18;
$numimage = imagecreate($imagewidth,$imageheight);
imagecolorallocate($numimage,240,240,240);
for($i=0;$i<strlen($num);$i++){
$x = mt_rand(1,8)+$imagewidth*$i/4;
$y = mt_rand(1,$imageheight/4);
$color=imagecolorallocate($numimage,mt_rand(0,150),mt_rand(0,150),mt_rand(0,150));
imagestring($numimage,5,$x,$y,$num[$i],$color);
}
for($i=0;$i<200;$i++){
$randcolor=imagecolorallocate($numimage,rand(200,255),rand(200,255),rand(200,255));
imagesetpixel($numimage,rand()%70,rand()%20,$randcolor);
}
imagepng($numimage);
imagedestroy($numimage);
?>
注册页面
register.php
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=gb2312" />
<title>注册</title>
<link rel="stylesheet" type="text/css" href="css/style.css" />
<script language="javascript" src="js/xmlhttprequest.js"></script>
<script language="javascript" src="js/register.js"></script>
</head>
<body>
<div id="container">
<div id="rgbgdiv">
<div id="regnamediv"><b>注册名称:</b>
<input id="regname" name="regname" type="text" />
<div id="namediv">请输入用户名</div>
</div>
<div id="regpwddiv1"><b>注册密码:</b>
<input id="regpwd1" name="regpwd1" type="password" />
<div id="pwddiv1">请输入密码</div>
</div>
<div id="regpwddiv2"><b>确认密码:</b>
<input id="regpwd2" name="regpwd2" type="password" />
<div id="pwddiv2">请输入确认密码</div>
</div>
<div id="regemaildiv"><b>电子邮箱:</b>
<input id="email" name="email" type="text" />
<div id="emaildiv">用户激活和找回密码使用</div>
</div>
<div id="morediv" style="display:none;">
<hr id="part" />
<div id="regquestiondiv"><b>密保问题:</b>
<input id="question" name="question" type="text" />
<div id="questiondiv">用户激活和找回密码使用</div>
</div>
<div id="reganswerdiv"><b>密保答案:</b>
<input id="answer" name="answer" type="text" />
<div id="answerdiv">用户激活和找回密码使用</div>
</div>
<div id="regrealnamediv"><b>真实姓名:</b>
<input id="realname" name="realname" type="text" />
<div id="realnamediv">用户的真实姓名</div>
</div>
<div id="regbirthdaydiv"><b>出生日期:</b>
<input id="birthday" name="birthday" type="text" />
<div id="birthdaydiv">用户的出生日期。格式:YYYY-MM-DD</div>
</div>
<div id="regtelephonediv"><b>联系电话:</b>
<input id="telephone" name="telephone" type="text" />
<div id="telephonediv">用户的联系电话</div>
</div>
<div id="regqqdiv"><b>QQ号 码:</b>
<input id="qq" name="qq" type="text" />
<div id="qqdiv">用户QQ号</div>
</div>
</div>
<div id="btndiv2">
<button id="regbtn" disabled="disabled"> </button>
<button id="morebtn"> </button>
<button id="logbtn"> </button>
</div>
</div>
<div id="imgdiv" style=" visibility: hidden;"> </div>
</div>
</body>
</html>
注册Ajax
register.js
// JavaScript Document
function $(id){
return document.getElementById(id);
}
window.onload = function(){
$('regname').focus();
var cname1,cname2,cpwd1,cpwd2,cemail;
//设置激活按钮
function chkreg(){
if((cname1 == 'yes') && (cname2 == 'yes') && (cpwd1 == 'yes') && (cpwd2 == 'yes') && (cemail == 'yes')){
$('regbtn').disabled = false;
}else{
$('regbtn').disabled = true;
}
}
//验证用户名
$('regname').onkeyup = function (){
name = $('regname').value;
cname2 = '';
if(name.match(/^[a-zA-Z_]*/) == ''){
$('namediv').innerHTML = '<font color=red>必须以字母或下划线开头</font>';
cname1 = '';
}else if(name.length < 2){
$('namediv').innerHTML = '<font color=red>注册名称必须大于等于2位</font>';
cname1 = '';
}else{
$('namediv').innerHTML = '<font color=green>注册名称符合标准</font>';
cname1 = 'yes';
}
chkreg();
}
//验证是否存在该用户
$('regname').onblur = function(){
name = $('regname').value;
if(cname1 == 'yes'){
xmlhttp.open('get','chkname.php?name='+name,true);
xmlhttp.onreadystatechange = function(){
if(xmlhttp.readyState == 4){
if(xmlhttp.status == 200){
var msg = xmlhttp.responseText;
if(msg == '1'){
$('namediv').innerHTML="<font color=green>恭喜您,该用户名可以使用!</font>";
cname2 = 'yes';
}else if(msg == '2'){
$('namediv').innerHTML="<font color=red>用户名被占用!</font>";
cname2 = '';
}else{
$('namediv').innerHTML="<font color=red>"+msg+"</font>";
cname2 = '';
}
}
}
chkreg();
}
xmlhttp.send(null);
}
}
//验证密码
$('regpwd1').onkeyup = function(){
pwd = $('regpwd1').value;
pwd2 = $('regpwd2').value;
if(pwd.length < 6){
$('pwddiv1').innerHTML = '<font color=red>密码长度最少需要6位</font>';
cpwd1 = '';
}else if(pwd.length >= 6 && pwd.length < 12){
$('pwddiv1').innerHTML = '<font color=green>密码符合要求。密码强度:弱</font>';
cpwd1 = 'yes';
}else if((pwd.match(/^[0-9]*$/)!=null) || (pwd.match(/^[a-zA-Z]*$/) != null )){
$('pwddiv1').innerHTML = '<font color=green>密码符合要求。密码强度:中</font>';
cpwd1 = 'yes';
}else{
$('pwddiv1').innerHTML = '<font color=green>密码符合要求。密码强度:高</font>';
cpwd1 = 'yes';
}
if(pwd2 != '' && pwd != pwd2){
$('pwddiv2').innerHTML = '<font color=red>两次密码不一致!</font>';
cpwd2 = '';
}else if(pwd2 != '' && pwd == pwd2){
$('pwddiv2').innerHTML = '<font color=green>密码输入正确</font>';
cpwd2 = 'yes';
}
chkreg();
}
//验证确认密码
$('regpwd2').onkeyup = function(){
pwd1 = $('regpwd1').value;
pwd2 = $('regpwd2').value;
if(pwd1 != pwd2){
$('pwddiv2').innerHTML = '<font color=red>两次密码不一致!</font>';
cpwd2 = '';
}else{
$('pwddiv2').innerHTML = '<font color=green>密码输入正确</font>';
cpwd2 = 'yes';
chkreg();
}
}
//验证email
$('email').onkeyup = function(){
emailreg = /^\w+([-+.]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*$/;
$('email').value.match(emailreg);
if($('email').value.match(emailreg) == null){
$('emaildiv').innerHTML = '<font color=red>错误的email格式</font>';
cemail = '';
}else{
$('emaildiv').innerHTML = '<font color=green>输入正确</font>';
cemail = 'yes';
}
chkreg();
}
//显示/隐藏详细信息
$('morebtn').onclick = function(){
if($('morediv').style.display == ''){
$('morediv').style.display = 'none';
}else{
$('morediv').style.display = '';
}
}
//登录按钮
$('logbtn').onclick = function(){
window.open('login.php','_parent','',false);
}
//正式注册
$('regbtn').onclick = function(){
$('imgdiv').style.visibility = 'visible';
url = 'register_chk.php?name='+$('regname').value+'&pwd='+$('regpwd1').value+'&email='+$('email').value;
url += '&question=' +$('question').value+'&answer='+$('answer').value;
url += '&realname=' +$('realname').value+'&birthday='+$('birthday').value;
url += '&telephone='+$('telephone').value+'&qq='+$('qq').value;
xmlhttp.open('get',url,true);
xmlhttp.onreadystatechange = function(){
if(xmlhttp.readyState == 4){
if(xmlhttp.status == 200){
msg = xmlhttp.responseText;
if(msg == '1'){
alert('注册成功,请到您的邮箱中获取激活码!');
location='index.php';
}else if(msg == '-1'){
alert('您的服务器不支持Zend_mail,或者邮箱填写错误。请仔细检查!!');
}else{
alert(msg);
}
$('imgdiv').style.visibility = 'hidden';
}
}
}
xmlhttp.send(null);
}
}
注册后台处理,发送验证邮件需要下载Zend Framework
register_chk.php
<?php
include_once 'conn/conn.php';
require_once 'Zend/Mail.php'; //调用发送邮件的文件
require_once 'Zend/Mail/Transport/Smtp.php'; //调用SMTP验证文件
$reback = '0';
$url = 'http://'.$_SERVER['SERVER_NAME'].dirname($_SERVER['SCRIPT_NAME']).'/activation.php';
$url .= '?name='.trim($_GET['name']).'&pwd='.md5(trim($_GET['pwd']));
//发送激活邮件
$subject="激活码的获取";
$mailbody='注册成功。您的激活码是:'.'<a href="'.$url.'" target="_blank">'.$url.'</a><br>'.'请点击该地址,激活您的用户!';
//定义邮件内容
$envelope="[email protected]"; //定义登录使用的邮箱
$config = array('auth' => 'login',
'username' => 'mrsoft8888',
'password' => 'mrsoft8888'); //定义SMTP的验证参数
$transport = new Zend_Mail_Transport_Smtp('smtp.sohu.com', $config); //实例化验证的对象
$mail = new Zend_Mail('GBK'); //实例化发送邮件对象
$mail->setBodyHtml($mailbody); //发送邮件主体
$mail->setFrom($envelope, '明日科技典型模块程序测试邮箱,恭喜您用户注册成功!'); //定义邮件发送使用的邮箱
$mail->addTo($_GET['email'], '获取用户注册激活码'); //定义邮件的接收邮箱
$mail->setSubject('获取注册用户的激活码'); //定义邮件主题
$mail->send($transport); //执行发送操作
/* 网络版发送邮件方法 */
$birthday='0000-00-00';
if($_GET['birthday']!='')
$birthday=$_GET['birthday'];
$sql = "insert into tb_member(name,password,question,answer,email,realname,birthday,telephone,qq) values('".trim($_GET['name'])."','".md5(trim($_GET['pwd']))."','".$_GET['question']."','".$_GET['answer']."','".$_GET['email']."','".$_GET['realname']."','".$birthday."','".$_GET['telephone']."','".$_GET['qq']."')";
$num = $conne->uidRst($sql);
if($num == 1){
$reback = '1';
}
echo $reback;
?>
验证用户名是否被占用
chkname.php
<?php
include_once "conn/conn.php";
$sql = "select * from tb_member where name='".$_GET['name']."'";
$num = $conne->getRowsNum($sql);
if($num == 1){
echo '2';
}else if($num == 0){
echo '1';
}else{
echo $conne->msg_error();
}
?>
找回密码的页面
found.php
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=gb2312" />
<title>找回密码</title>
<link rel="stylesheet" href="css/style.css" />
<script language="javascript" src="js/found.js"></script>
<script language="javascript" src="js/xmlhttprequest.js"></script>
</head>
<body>
<div id="fdbgdiv" >
<div id="top"> >>密码找回</div>
<div id="foundnamediv">找回账号: <input id="foundname" type="text" style=" width: 100px; height:15px; border:1px #000000 solid;" /></div>
<div id="foundnamediv">密保问题: <input id="fdquestion" type="text" style=" width: 100px; height:15px; border:1px #000000 solid;" /></div>
<div id="foundnamediv">密保答案: <input id="fdanswer" type="text" style=" width: 100px; height:15px; border:1px #000000 solid;" /></div>
<div id="foundnamediv" align="center"><button id="step1"></button></div>
</div>
</body>
</html>
找回密码的Ajax
found.js
// JavaScript Document
function $(id){
return document.getElementById(id);
}
window.onload = function(){
$('foundname').focus();
$('step1').onclick = function(){
if($('foundname').value != '' && $('fdquestion').value != '' && $('fdanswer').value != ''){
xmlhttp.open('get','found_chk.php?foundname='+$('foundname').value+'&question='+$('fdquestion').value+'&answer='+$('fdanswer').value,true);
xmlhttp.onreadystatechange = function(){
if(xmlhttp.readyState == 4 && xmlhttp.status == 200){
msg = xmlhttp.responseText;
if(msg == '1'){
alert('找回密码成功,请登录邮箱注册邮箱!');
window.close();
}else{
alert('填写的信息错误');
}
}
}
xmlhttp.send(null);
}else{
alert('请填写完成信息');
$('foundname').focus();
return false;
}
}
}
found_chk.php
找回密码的后台,发送包含密码的邮件,与注册时类似
<?php
include_once 'conn/conn.php';
require_once 'Zend/Mail.php'; //调用发送邮件的文件
require_once 'Zend/Mail/Transport/Smtp.php'; //调用SMTP验证文件
$reback = '0';
$name = $_GET['foundname'];
$question = $_GET['question'];
$answer = $_GET['answer'];
$sql = "select email from tb_member where name = '".$name."' and question = '".$question."' and answer = '".$answer."'";
$email = $conne->getFields($sql,0);
if($email != '')
{
$rnd = rand(1000,time());
$sql = "update tb_member set password = '".md5($rnd)."' where name = '".$name."' and question = '".$question."' and answer = '".$answer."'";
$tmpnum = $conne->uidRst($sql);
if($tmpnum >= 1)
{
$subject="找回密码";
$mailbody='密码找回成功。您帐号的新密码是'.$rnd;
$reback='1';
$envelope="[email protected]";
$config = array('auth' => 'login',
'username' => 'mrsoft8888',
'password' => 'mrsoft8888'); //定义SMTP的验证参数
$transport = new Zend_Mail_Transport_Smtp('smtp.sohu.com', $config); //实例化验证的对象
$mail = new Zend_Mail('GBK'); //实例化发送邮件对象
$mail->setBodyHtml($mailbody); //发送邮件主体
$mail->setFrom($envelope, '明日科技典型模块程序测试邮箱,修改用户注册密码!'); //定义邮件发送使用的邮箱
$mail->addTo($email, '获取用户新密码'); //定义邮件的接收邮箱
$mail->setSubject($subject); //定义邮件主题
$mail->send($transport);
}
else
{
$reback = $sql;
}
}
echo $reback;
?>
利用邮件的超链接激活页面
activation.php
<?php
session_start();
header('Content-Type:text/html;charset=gb2312');
include_once("conn/conn.php");
if (!empty($_GET['name']) && !is_null($_GET['name'])){ //激活注册用户
$num=$conne->getRowsNum("select * from tb_member where name='".$_GET['name']."' and password = '".$_GET['pwd']."'");
if ($num>0){
$upnum=$conne->uidRst("update tb_member set active = 1 where name='".$_GET['name']."' and password = '".$_GET['pwd']."'");
if($upnum > 0){
$_SESSION['name'] = $_GET['name'];
echo "<script>alert('用户激活成功!');window.location.href='main.php';</script>";
}else{
echo "<script>alert('您已经激活!');window.location.href='main.php';</script>";
}
}else{
echo "<script>alert('用户激活失败!');window.location.href='register.php';</script>";
}
}
?>
------------修改自<<PHP开发典型模块大全>>-------------------
详细↓
http://www.cnblogs.com/xchaos/archive/2012/03/10/2389479.html