题目描述:
Given an array with n
integers, your task is to check if it could become non-decreasing by modifying at most 1
element.
We define an array is non-decreasing if array[i] <= array[i + 1]
holds for every i
(1 <= i < n).
Example 1:
Input: [4,2,3] Output: True Explanation: You could modify the first4
to1
to get a non-decreasing array.
Example 2:
Input: [4,2,1] Output: False Explanation: You can't get a non-decreasing array by modify at most one element.
Note: The n
belongs to [1, 10,000].
题目描述:
判断一个数组是否可以通过修改一个数组值变为非递减数组。
扫描二维码关注公众号,回复:
4326791 查看本文章
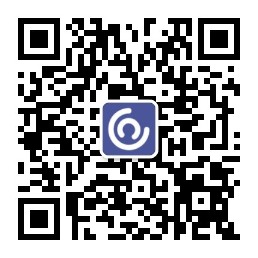
解题思路:
在出现 nums[i] < nums[i - 1] 时,需要考虑的是应该修改数组的哪个数,使得本次修改能使 i 之前的数组成为非递减数组,并且不影响后续的操作 。优先考虑令 nums[i - 1] = nums[i],因为如果修改 nums[i] = nums[i - 1] 的话,那么 nums[i] 这个数会变大,就有可能比 nums[i + 1] 大,从而影响了后续操作。还有一个比较特别的情况就是 nums[i] < nums[i - 2],只修改 nums[i - 1] = nums[i] 不能使数组成为非递减数组,只能修改 nums[i] = nums[i - 1]。具体解题思路及代码如下:
package Leetcode_Github; public class GreedyThought_NonDecreasingArray_665_1112 { public boolean CheckPossibility(int[] nums){ if (nums == null || nums.length <= 1) { return true; } //用来记录修改数组次数 int count = 0; for (int i = 1; i < nums.length && count < 2; i++) { //如果索引i的值比i-1的值大,进行下一次循环 if (nums[i] >= nums[i - 1]) { continue; } count++; //如果nums[i]的值比nums[i-2]的值小,将nums[i-1]的值赋给nums[i] if (i >= 2 && nums[i] < nums[i - 2]) { nums[i] = nums[i - 1]; } else //如果只是nums[i]的值小于nums[i-1],将nums[i]的值赋给nums[i-1] nums[i - 1] = nums[i]; } //如果数组修改次数为0或者1,返回true return count <= 1; } public static void main(String[] args) { int[] array = {3, 4, 2, 3}; GreedyThought_NonDecreasingArray_665_1112 test = new GreedyThought_NonDecreasingArray_665_1112(); boolean result = test.CheckPossibility(array); System.out.println(result); } }