一、Mybatis与Spring集成
1.1.集成思想
1.由spring来管理数据源和事务
2.需要通过spring通过单例的方式来管理SqlSessionFactory(在程序中每次创建一个工厂都会消耗很多资源,以及降程序的运行效率,因此通过所有的SqlSession都通过一个SqlSessionFactory来创建,不需要每个SqlSession都由一个工程来创建,这样太影响性能了)
3.持久层Mapper接口也可以通过Spring来整合,自动生成Mapper的代理对象。
1.2.导入jar包:
spring相关的jar包、mybatis相关的jar包、数据库驱动jar包、日志jar包、mybatis整合spring的jar包、数据库连接池jar包
1.3.创建工程
1.4.编码阶段
这里通过一个案例来进行演示:
通过查账户的余额;
数据库设计:
accoun表
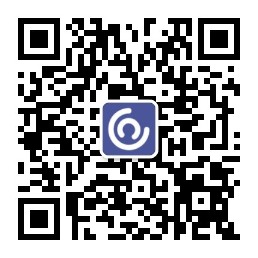
整合传统的dao层开发:
编写Po类:Account
public class Account { private Integer id; private String username; private Integer money; //省略getter和setter方法 }
编写Account.xml映射文件:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <!-- namespace:命名空间,它的作用就是对SQL进行分类化管理,可以理解为SQL隔离 注意:使用mapper代理开发时,namespace有特殊且重要的作用 --> <mapper namespace="test"> <!-- 根据id查询account --> <select id="findAccountById" parameterType="Integer" resultType="account"> SELECT * FROM ACCOUNT WHERE id=#{id} </select> </mapper>
编写SqlMapConfig.xml全局配置文件:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!-- 设置别名 --> <typeAliases> <package name="com.lc.po"/> </typeAliases> <!-- 加载映射文件 --> <mappers> <mapper resource="mybatis/Account.xml"/> </mappers> </configuration>
编写dao层:
dao层接口:
public interface AccountDao { public Account findAccountById(Integer id); }
dao层实现类:
dao层实现类通过继承spring整合Mybatis的SqlSessionDaoSupport,这样我们就不需要去创建SqlSession,而是Spring整合就给我们自动创建了。但是需要向该类注入SqlSessionFactory
public class AccountDaoImpl extends SqlSessionDaoSupport implements AccountDao{ @Override public Account findAccountById(Integer id) { return this.getSqlSession().selectOne("test.findAccountById", id); } @Override public void updateAccountMoney(NumberClass num) { this.getSqlSession().update("test.outMoney", num); } }编写service层:
service实现类:
public interface AccountService { public Account findAccountById(); }
service实现类:
public class AccountServiceImpl implements AccountService{ //方式一:注入accountDao接口 private AccountDao accountDao; public void setAccountDao(AccountDao accountDao) { this.accountDao = accountDao; } @Override public Account findAccountById() { return accountDao.findAccountById(2); } }
编写Spring配置文件applicationContext.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd "> <!-- 加载db.properties文件 --> <context:property-placeholder location="db.properties"/> <!-- 加载数据源 --> <bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource"> <property name="driverClassName" value="${db.driver}" /> <property name="url" value="${db.url}" /> <property name="username" value="${db.username}" /> <property name="password" value="${db.password}" /> <property name="maxActive" value="10" /> <property name="maxIdle" value="5" /> </bean> <!-- 由spring来加载事务管理器 --> <bean id="txManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <!-- 注入数据源 --> <property name="dataSource" ref="dataSource"></property> </bean> <!-- 配置事物通知 --> <tx:advice id="tx_advice" transaction-manager="txManager"> <tx:attributes> <tx:method name="find*" read-only="true"/> </tx:attributes> </tx:advice> <!-- 进行aop编程 --> <aop:config> <aop:pointcut expression="execution(* com.lc.service.AccountServiceImpl.*(..))" id="pointcutAccount"/> <aop:advisor advice-ref="tx_advice" pointcut-ref=""/> </aop:config> <!-- 配置sqlSessionFactory --> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <!-- 注入mybatis配置文件位置 --> <property name="configLocation" value="mybatis/SqlMapConfig.xml"></property> <!-- 注入数据源 --> <property name="dataSource" ref="dataSource"></property> </bean> <!-- 由Spring管理AccountDao --> <bean id="accountDao" class="com.lc.dao.AccountDaoImpl"> <!-- 注入sqlSessionFactory --> <property name="sqlSessionFactory" ref="sqlSessionFactory"></property> </bean> <!-- 由Spring管理AccountService --> <bean id="accountService" class="com.lc.service.AccountServiceImpl"> <!-- 注入accountDao --> <property name="accountDao" ref="accountDao"></property> </bean> </beans>
创建db.propertis:
db.driver=com.mysql.jdbc.Driver db.url=jdbc:mysql://localhost:3306/spring_study_day02 db.username=root db.password=12345678
编写测试类:
public class AccountServiceTest { private ApplicationContext application; @Before public void setUp() { application = new ClassPathXmlApplicationContext("spring/applicationContext.xml"); } @Test public void testFindAccountById() { AccountService accountService = (AccountService) application.getBean("accountService"); Account account = accountService.findAccountById(); System.out.println(account); } }
整合mapper接口方式开发
编写Po类:Account
public class Account { private Integer id; private String username; private Integer money; //省略getter和setter方法 }
编写AccountMapper.xml映射文件:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <!-- namespace:命名空间,它的作用就是对SQL进行分类化管理,可以理解为SQL隔离 注意:使用mapper代理开发时,namespace有特殊且重要的作用 --> <mapper namespace="com.lc.mapper.AccountMapper"> <!-- 根据id查询account --> <select id="findAccountById" parameterType="Integer" resultType="account"> SELECT * FROM ACCOUNT WHERE id=#{id} </select> </mapper>
编写SqlMapConfig.xml全局配置文件:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!-- 设置别名 --> <typeAliases> <package name="com.lc.po"/> </typeAliases> <!-- 加载映射文件 --> <mappers> <!-- <mapper resource="mybatis/Account.xml"/> --> <package name="com.lc.mapper"/> </mappers> </configuration>
编写Mapper接口:
public interface AccountMapper { public Account findAccountById(Integer id); }
编写service层:
service接口:
public interface AccountService { public Account findAccountById(); }
service实现类:
public class AccountServiceImpl implements AccountService{ //方式二:注入mapper接口代理类 private AccountMapper accountMapper; public void setAccountMapper(AccountMapper accountMapper) { this.accountMapper = accountMapper; } @Override public Account findAccountById() { return accountMapper.findAccountById(1); } }
编写Spring配置文件applicationContext.xml:
这里是通过MapperFacotryBean生成单个Mapper接口的代理类:这种方式不推荐:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd "> <!-- 加载db.properties文件 --> <context:property-placeholder location="db.properties"/> <!-- 加载数据源 --> <bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource"> <property name="driverClassName" value="${db.driver}" /> <property name="url" value="${db.url}" /> <property name="username" value="${db.username}" /> <property name="password" value="${db.password}" /> <property name="maxActive" value="10" /> <property name="maxIdle" value="5" /> </bean> <!-- 由spring来加载事务管理器 --> <bean id="txManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <!-- 注入数据源 --> <property name="dataSource" ref="dataSource"></property> </bean> <!-- 配置事物通知 --> <tx:advice id="tx_advice" transaction-manager="txManager"> <tx:attributes> <tx:method name="find*" read-only="true"/> </tx:attributes> </tx:advice> <!-- 进行aop编程 --> <aop:config> <aop:pointcut expression="execution(* com.lc.service.AccountServiceImpl.*(..))" id="pointcutAccount"/> <aop:advisor advice-ref="tx_advice" pointcut-ref="pointcutAccount"/> </aop:config> <!-- 配置sqlSessionFactory --> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <!-- 注入mybatis配置文件位置 --> <property name="configLocation" value="mybatis/SqlMapConfig.xml"></property> <!-- 注入数据源 --> <property name="dataSource" ref="dataSource"></property> </bean> <!-- 由Spring创建accountMapper的代理类 --> <!-- 这种方式是:单个mapper代理类配置 --> <bean id="accountMapper" class="org.mybatis.spring.mapper.MapperFactoryBean"> <!-- 注入代理接口 --> <property name="mapperInterface" value="com.lc.mapper.AccountMapper"></property> <!-- 注入sqlSessionFactory --> <property name="sqlSessionFactory" ref="sqlSessionFactory"></property> </bean> <!-- 由Spring管理AccountService --> <bean id="accountService" class="com.lc.service.AccountServiceImpl"> <!-- 注入mapper代理类 --> <property name="accountMapper" ref="accountMapper"></property> </bean> </beans>
这里是MapperScannerConfigurer类来批量的生成Mappr接口的代理,需要向该类注入Mapper接口所在的包basePackage的值(注意该种方式生成的Mapper接口代理类的默认id为Mapper接口名称且首字母小写);这种方式推荐
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd "> <!-- 加载db.properties文件 --> <context:property-placeholder location="db.properties"/> <!-- 加载数据源 --> <bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource"> <property name="driverClassName" value="${db.driver}" /> <property name="url" value="${db.url}" /> <property name="username" value="${db.username}" /> <property name="password" value="${db.password}" /> <property name="maxActive" value="10" /> <property name="maxIdle" value="5" /> </bean> <!-- 由spring来加载事务管理器 --> <bean id="txManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <!-- 注入数据源 --> <property name="dataSource" ref="dataSource"></property> </bean> <!-- 配置事物通知 --> <tx:advice id="tx_advice" transaction-manager="txManager"> <tx:attributes> <tx:method name="find*" read-only="true"/> </tx:attributes> </tx:advice> <!-- 进行aop编程 --> <aop:config> <aop:pointcut expression="execution(* com.lc.service.AccountServiceImpl.*(..))" id="pointcutAccount"/> <aop:advisor advice-ref="tx_advice" pointcut-ref="pointcutAccount"/> </aop:config> <!-- 配置sqlSessionFactory --> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <!-- 注入mybatis配置文件位置 --> <property name="configLocation" value="mybatis/SqlMapConfig.xml"></property> <!-- 注入数据源 --> <property name="dataSource" ref="dataSource"></property> </bean> <!-- 由Spring创建accountMapper的代理类 --> <!-- 批量mapper代理类配置:默认bean的id为类名(首字母小写) --> <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <!-- 注入扫描包 --> <property name="basePackage" value="com.lc.mapper"></property> <!-- 指定使用的SqlSessionFactory --> <property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"></property> </bean> <!-- 由Spring管理AccountService --> <bean id="accountService" class="com.lc.service.AccountServiceImpl"> <!-- 注入mapper代理类 --> <property name="accountMapper" ref="accountMapper"></property> </bean> </beans>
测试类:
public class AccountServiceTest { private ApplicationContext application; @Before public void setUp() { application = new ClassPathXmlApplicationContext("spring/applicationContext.xml"); } @Test public void testFindAccountById() { AccountService accountService = (AccountService) application.getBean("accountService"); Account account = accountService.findAccountById(); System.out.println(account); } }