科学计算库Numpy
1、读取文件
numpy.gerfromtxt()用于读取文件,其中传入的参数依次是:
1、需要读取txt文件位置,此处文件与程序位于同一目录下
2、delimiter 分割的标记
3、dtype 转换类型,如果文件中既有文本类型也有数字类型,就先转成文本类型
help(numpy.genformtxt)用于查看帮助文档


1 import numpy 2 3 world_alcohol = numpy.genfromtxt("world_alcohol.txt", delimiter=",",dtype=str) 4 print(type(world_alcohol)) 5 print(world_alcohol) 6 print(help(numpy.genfromtxt))
2、构造ndarray
numpy.array()中传入数组参数,可以是一维的也可以是二维三维的,numpy会将其转变成ndayyay结构。


1 import numpy 2 vector = numpy.array([1,2,3,4]) 3 matrix = numpy.array([[1,2,3],[4,5,6]]) 4 print(vector) 5 print("----------------------") 6 print(matrix) 7 # [1 2 3 4] 8 # ---------------------- 9 # [[1 2 3] 10 # [4 5 6]]
注意:传入的参数必须是同一结构,不是同一结构将会发生转换


1 import numpy 2 vector1 = numpy.array([1,2,3,4.]) 3 vector2 = numpy.array([1,2,3,4]) 4 vector3 = numpy.array([1,2,'3',4]) 5 print(vector1) # [1. 2. 3. 4.] 6 print(vector2) # [1,2,3,4] 7 print(vector3) # ['1' '2' '3' '4']
利用.shape查看结构


1 import numpy 2 vector = numpy.array([1,2,3,4]) 3 matrix = numpy.array([[1,2,3],[4,5,6]]) 4 print(vector.shape) 5 print(matrix.shape) 6 7 # (4,) 8 # (2, 3)
利用.dtype查看类型


1 import numpy 2 vector = numpy.array([1,2,3,4]) 3 print(vector.dtype)# int32
利用.ndim查看维度


1 import numpy 2 vector = numpy.array([1,2,3,4]) 3 matrix = numpy.array([[1,2,3],[4,5,6],[7,8,9]]) 4 print(vector.ndim)# 1 5 print(matrix.ndim)# 2
利用.size查看元素的数量
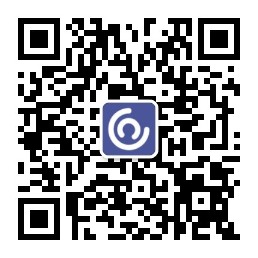
print(matrix.size) # 9
3、获取与计算
切片索引取值
vector = numpy.array([5, 10, 15, 20]) print(vector[0:3]) # 切片索引取值# [ 5 10 15]
利用返回值获取元素
import numpy vector = numpy.array([5, 10, 15, 20]) print(vector == 10) # [False True False False] # 利用返回值获取元素 print(vector[vector == 10])# [10]
将整型类型进行转换
vector = vector.astype(str) print(vector.dtype)# <U11
求和
matrix = numpy.array([[1,2,3], [4,5,6], [7,8,9]]) print(matrix.sum())# 45 print(matrix.sum(1))# 按每行求和 # [ 6 15 24] print(matrix.sum(0))# 按每列求和 # [12 15 18]
sum(1) 是 sum(axis=1)) 的缩写,1表示按照 x轴方向求和,0表示按照y轴方向求和
4、常用函数
reshape:生成0-14的15个数字,使用reshape(3,5)将其构造成一个三行五列的array


1 import numpy as np 2 arr = np.arange(15).reshape(3, 5) 3 arr 4 5 array([[ 0, 1, 2, 3, 4], 6 [ 5, 6, 7, 8, 9], 7 [10, 11, 12, 13, 14]])
zero:生成指定结构的默认为0.的array


1 np.zeros ((3,4)) 2 3 #array([[ 0., 0., 0., 0.], 4 # [ 0., 0., 0., 0.], 5 # [ 0., 0., 0., 0.]])
ones:生成一个三维的array,通过dtype指定类型


1 np.ones( (2,3,4), dtype=np.int32 ) 2 3 # array([[[1, 1, 1, 1], 4 # [1, 1, 1, 1], 5 # [1, 1, 1, 1]], 6 7 # [[1, 1, 1, 1], 8 # [1, 1, 1, 1], 9 # [1, 1, 1, 1]]])
arange指定范围和数值间的间隔array,注意范围包左不包右


1 np.arange(0,10,2) 2 3 # array([0, 2, 4, 6, 8])
random随机数:生成指定结构的随机数,可以用于生成随机权重


1 np.random.random((2,3)) 2 3 # array([[ 0.86166627, 0.37756207, 0.94265883], 4 # [ 0.9768257 , 0.96915312, 0.33495431]])
ndarray运算:矩阵之间的相加、相减、开根号、e平方


1 a = np.array([10,20,30,40]) 2 b = np.array(4) 3 4 print(a - b) # array([ 6, 16, 26, 36]) 5 print(a**2) # array([ 100, 400, 900, 1600]) 6 print(np.sqrt(B)) # array([[ 1.41421356, 0. ], 7 # [ 1.73205081, 2. ]]) 8 9 print(np.exp(B)) # array([[ 7.3890561 , 1. ], 10 # [ 20.08553692, 54.59815003]])
向下取整np.floor()和向下取整np.ceil()


1 import numpy as np 2 a = np.floor(10*np.random.random((2,2))) 3 print(a) 4 a = np.ceil(np.random.random((2,2))) 5 print(a) 6 7 # [[2. 8.] 8 # [5. 0.]] 9 ####################### 10 # [[1. 1.] 11 # [1. 1.]]
转置(行列变换)a.T


1 import numpy as np 2 a = np.array([[1,2],[3,4]]) 3 print(a) 4 print(a.T)# 转置 5 # [[1 2] 6 # [3 4]] 7 ################ 8 # [[1 3] 9 # [2 4]]
变换结构a.resize(1,4)


1 import numpy as np 2 a = np.array([[1,2],[3,4]]) 3 a.resize(1,4) 4 a# array([[1, 2, 3, 4]])
矩阵运算
A*B
A.dot(B)
np.dot(A,B)


1 import numpy as np 2 A = np.array( [[1,1], 3 [0,1]] ) 4 B = np.array( [[2,0], 5 [3,4]] ) 6 print(A*B) 7 8 print (A.dot(B))# A*B 9 print(np.dot(A,B))# A*B 10 # [[2 0] 11 # [0 4]]
横向相加 np.hstack(a,b)


1 a = np.floor(10*np.random.random((2,2))) 2 b = np.floor(10*np.random.random((2,2))) 3 4 print(a) 5 print(b) 6 print(np.hstack((a,b))) 7 8 # [[ 2. 3.] 9 # [ 9. 3.]] 10 # [[ 8. 1.] 11 # [ 0. 0.]] 12 # [[ 2. 3. 8. 1.] 13 # [ 9. 3. 0. 0.]]
纵向相加 np.vstack(a,b)


1 print(np.vstack((a,b))) 2 3 # [[ 2. 3.] 4 # [ 9. 3.] 5 # [ 8. 1.] 6 # [ 0. 0.]]
矩阵纵向切割np.hsplit(a,3) # 把a竖切成3分


1 a = np.floor(10*np.random.random((2,12))) 2 print(a) 3 print(np.hsplit(a,3)) 4 5 # [[1. 4. 9. 1. 7. 2. 6. 3. 5. 4. 1. 8.] 6 # [0. 0. 4. 4. 7. 9. 1. 6. 7. 3. 9. 2.]] 7 8 9 # [array([[1., 4., 9., 1.], 10 # [0., 0., 4., 4.]]), 11 # array([[7., 2., 6., 3.], 12 # [7., 9., 1., 6.]]), 13 # array([[5., 4., 1., 8.], 14 # [7., 3., 9., 2.]])]
矩阵横向切割 np.vsplit(a,3) # 把a横的切成3分


1 b = np.floor(10*np.random.random((12,2))) 2 print(b) 3 print(np.vsplit(b,3)) 4 5 # [[8. 0.] 6 # [9. 1.] 7 # [9. 1.] 8 # [7. 7.] 9 # [7. 1.] 10 # [9. 0.] 11 # [8. 0.] 12 # [7. 4.] 13 # [8. 8.] 14 # [7. 5.] 15 # [9. 8.] 16 # [1. 5.]] 17 18 [array([[8., 0.], 19 [9., 1.], 20 [9., 1.], 21 [7., 7.]]), 22 array([[7., 1.], 23 [9., 0.], 24 [8., 0.], 25 [7., 4.]]), 26 array([[8., 8.], 27 [7., 5.], 28 [9., 8.], 29 [1., 5.]])]
复制的区别
地址复制:通过 b = a 复制 a 的值,b 与 a 指向同一地址,改变 b 同时也改变 a。


1 a = np.arange(12) 2 b = a 3 print(a is b) 4 5 print(a.shape) 6 print(b.shape) 7 b.shape = (3,4) 8 print(a.shape) 9 print(b.shape) 10 11 # True 12 # (12,) 13 # (12,) 14 # (3, 4) 15 # (3, 4)
复制值:通过 a.view() 仅复制值,当对 c 值进行改变会改变 a 的对应的值,而改变 c 的 shape 不改变 a 的 shape,只有值会变,矩阵结构不变


1 a = np.arange(12)# [ 0 1 2 3 4 5 6 7 8 9 10 11] 2 c = a.view() 3 print(c is a) 4 5 c.shape = (2,6) 6 c[0,0] = 9999 7 8 print(a) 9 print(c) 10 11 # False 12 # [9999 1 2 3 4 5 6 7 8 9 10 11] 13 # [[9999 1 2 3 4 5] 14 # [ 6 7 8 9 10 11]]
完全拷贝:a.copy() 进行的完整的拷贝,产生一份完全相同的独立的复制


1 a = np.arange(12) 2 c = a.copy() 3 print(c is a) 4 5 c.shape = 2,6 6 c[0,0] = 9999 7 8 print(a) 9 print(c) 10 11 # False 12 # [ 0 1 2 3 4 5 6 7 8 9 10 11] 13 # [[9999 1 2 3 4 5] 14 # [ 6 7 8 9 10 11]]