第一部分:理论知识
1.AWT与Swing简介
(1)Swing用户界面库是非基于对等体的GUI工具箱。
Swing具有更丰富并且更方便的用户界面元素集合。
Swing对底层平台的依赖很少,因此与平台相关的bug很少。
Swing会带来交叉平台上的统一视觉体验。
Swing类库被放在javax.swing包里。
(2)两者之间的关系:
大部分AWT组件都有其Swing的等价组件。
Swing组件的名字一般是在AWT组件名前面添加一个字母“J”,如:JButton,JFrame,JPanel等。
2.创建框架
(1)组件:通常把由Component类的子类或间接子类创建的对象称为一个组件。
(2)容器是Java中能容纳和排列组件的组件。.常用的容器是框架(Frame,JFrame)
(3)Container类提供了一个方法add(),用来在容器类组件对象中添加其他组件。
(4)容器本身也是一个组件,可以把一个容器添加到另一个容器里,实现容器嵌套。
3.框架
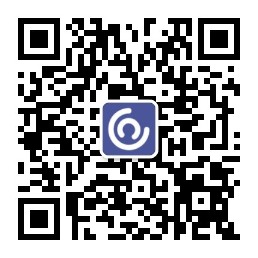
(1)创建空框架
. 在Java中,常采用框架(Frame)创建初始界面,即GUI的顶层窗口. AWT库中有一个基于对等体的Frame类。
. 该类的Swing版本为JFrame,JFrame是Frame子类。
定位:
—常用Component类的setLocation和setBounds方法常用属性
—Title:框架标题
—IconImage:框架图标
java.awt.Component 1.0
– boolean isVisible() 检查组件是否可见
– void setVisible(boolean b) 设置组件可见
– void setSize(int width,int height) 把组件缩放到指
定宽度和高度
– void setBounds(int x,int y,int
width,int height) 移动并缩放组件
– Dimension getSize() 得到组件的大小
– void setSize(Dimension d) 把组件缩放到指定的大小
4.2D图形
(1)Java SE了包含一个Java 2D库,该库提供了一个非常强大的图形操作集。
(2) Graphics 2D 类是Graphics 类的子类, Graphics2D类对象通常可用Graphics对象转换而来。
5.颜色的使用
Graphics2D类的setPaint方法(Graphics类为setColor方法)用来设置颜色。
例:g2.setPaint(Color.RED);g2.drawString(“Set Color”,100,100);
(1)Color类中定义的13种标准颜色
BLACK、BLUE、CYAN、DARK_GRAY、GRAY、GREEN、
LIGHT_GRAY、MAGENTA、ORANGE、PINK、RED、
WHITE、YELLOW
6,字体的使用:
(1)AWT中定义的五种逻辑字体名
SanaSerif
Serif
Monospaced
Dialog
DialogInput
这些逻辑字体在不同语言和操作系统上映射为不同
的物理字体。
(2)字体风格
Font.PLAIN
Font.BOLD
Fond.ITALIC
Fond.BOLD + Font.ITALIC
(3)设置字体
Font serif=new Font(“Serif”,Font.BOLD,14);
g2.setFont(serif);
第二部分:实验部分:
1、实验目的与要求
(1) 掌握Java GUI中框架创建及属性设置中常用类的API;
(2) 掌握Java GUI中2D图形绘制常用类的API;
(3) 了解Java GUI中2D图形中字体与颜色的设置方法;
(4) 了解Java GUI中2D图像的载入方法。
2、实验内容和步骤
实验1: 导入第10章示例程序,测试程序并进行代码注释。
测试程序1:
l 运行下列程序,观察程序运行结果。
import javax.swing.*; public class SimpleFrameTest { public static void main(String[] args) { JFrame frame = new JFrame(); frame.setBounds(0, 0,300, 200); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } } |
package demo; import javax.swing.*; public class SimpleFrameTest { public static void main(String[] args) { JFrame frame = new JFrame(); frame.setBounds(300, 300,300, 300);//前两个参数是位置,后两个参数是窗口的长和宽 frame.setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE);//设置默认的关闭操作 frame.setVisible(false); //设置窗口是否可见 } }
l 在elipse IDE中调试运行教材407页程序10-1,结合程序运行结果理解程序;与上面程序对比,思考异同;
l 掌握空框架创建方法;
l 了解主线程与事件分派线程概念;
l 掌握GUI顶层窗口创建技术。
package simpleFrame; import java.awt.*; import javax.swing.*; /** * @version 1.34 2018-04-10 * @author Cay Horstmann */ public class SimpleFrameTest { public static void main(String[] args) { EventQueue.invokeLater(() -> { SimpleFrame frame = new SimpleFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// frame.setVisible(true);//判断页面是否可见 }); } } class SimpleFrame extends JFrame { private static final int DEFAULT_WIDTH = 300; private static final int DEFAULT_HEIGHT = 200; public SimpleFrame()//构造器 { setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);//设置弹出页面的大小 } }
测试程序2:
l 在elipse IDE中调试运行教材412页程序10-2,结合程序运行结果理解程序;
l 掌握确定框架常用属性的设置方法。
package sizedFrame; import java.awt.*; import javax.swing.*; /** * @version 1.35 2018-04-10 * @author Cay Horstmann */ public class SizedFrameTest { public static void main(String[] args) { EventQueue.invokeLater(() -> { SizedFrame frame = new SizedFrame(); frame.setTitle("SizedFrame"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); }); } } class SizedFrame extends JFrame { public SizedFrame() { //获取屏幕尺寸 Toolkit kit = Toolkit.getDefaultToolkit(); Dimension screenSize = kit.getScreenSize(); int screenHeight = screenSize.height; int screenWidth = screenSize.width; // 设置框架宽度、高度并让平台选择屏幕位置 setSize(screenWidth / 2, screenHeight / 2); setLocationByPlatform(true); //设置帧图标 Image img = new ImageIcon("icon.gif").getImage(); //生成ImgeIcon类对象,调用此对象的getImage方法 setIconImage(img); //用来定义用户界面的图标 } }
测试程序3:
l 在elipse IDE中调试运行教材418页程序10-3,结合运行结果理解程序;
l 掌握在框架中添加组件;
l 掌握自定义组件的用法。
package notHelloWorld; import javax.swing.*; import java.awt.*; /** * @version 1.34 2018-04-10 * @author Cay Horstmann */ public class NotHelloWorld { public static void main(String[] args) { EventQueue.invokeLater(() -> { NotHelloWorldFrame frame = new NotHelloWorldFrame(); frame.setTitle("NotHelloWorld"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); }); } } /** * A frame that contains a message panel */ class NotHelloWorldFrame extends JFrame { public NotHelloWorldFrame() { add(new NotHelloWorldComponent());//将指定组件追加到此容器的尾部 pack(); } } /** * A component that displays a message. */ class NotHelloWorldComponent extends JComponent { public static final int MESSAGE_X = 75; public static final int MESSAGE_Y = 100; private static final int DEFAULT_WIDTH = 300; private static final int DEFAULT_HEIGHT = 200; public void paintComponent(Graphics g) { g.drawString("Not a Hello, World program", MESSAGE_X, MESSAGE_Y); } public Dimension getPreferredSize() { return new Dimension(DEFAULT_WIDTH, DEFAULT_HEIGHT); } }
测试程序4:
l 在elipse IDE中调试运行教材424 -425页程序10-4,结合程序运行结果理解程序;
l 掌握2D图形的绘制方法。
package draw; import java.awt.*; import java.awt.geom.*; import java.awt.geom.Rectangle2D.Double; import javax.swing.*; /** * @version 1.34 2018-04-10 * @author Cay Horstmann */ public class DrawTest { public static void main(String[] args) { EventQueue.invokeLater(() -> { DrawFrame frame = new DrawFrame(); frame.setTitle("DrawTest"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); }); } } /** * A frame that contains a panel with drawings */ class DrawFrame extends JFrame { public DrawFrame() { add(new DrawComponent()); pack(); } } /** * A component that displays rectangles and ellipses. */ class DrawComponent extends JComponent { private static final int DEFAULT_WIDTH = 400; private static final int DEFAULT_HEIGHT = 400; public void paintComponent(Graphics g) { Graphics2D g2 = (Graphics2D) g; // 画一个长方形; double leftX = 100; double topY = 100; double width = 200; double height = 150; Double rect = new Rectangle2D.Double(leftX, topY, width, height); g2.draw(rect); // 画封闭椭圆 java.awt.geom.Ellipse2D.Double ellipse = new Ellipse2D.Double(); ellipse.setFrame(rect); g2.draw(ellipse); // 画一条对角线 g2.draw(new Line2D.Double(leftX, topY, leftX + width, topY + height)); //用同一个中心画一个圆 double centerX = rect.getCenterX(); double centerY = rect.getCenterY(); double radius = 150; java.awt.geom.Ellipse2D.Double circle = new Ellipse2D.Double(); circle.setFrameFromCenter(centerX, centerY, centerX + radius, centerY + radius); g2.draw(circle); } public Dimension getPreferredSize() { return new Dimension(DEFAULT_WIDTH, DEFAULT_HEIGHT); }
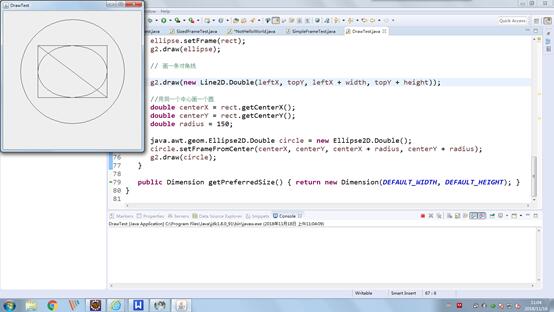
}
测试程序5:
l 在elipse IDE中调试运行教材432页-433程序10-5,结合程序运行结果理解程序;
l 了解2D图形中字体的设置的方法;
package font; import java.awt.*; import java.awt.font.*; import java.awt.geom.*; import java.awt.geom.Rectangle2D.Double; import javax.swing.*; /** * @version 1.35 2018-04-10 * @author Cay Horstmann */ public class FontTest { public static void main(String[] args) { EventQueue.invokeLater(() -> { FontFrame frame = new FontFrame(); frame.setTitle("FontTest"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); }); } } /** * A frame with a text message component */ class FontFrame extends JFrame { public FontFrame() { add(new FontComponent()); pack(); } } /** * A component that shows a centered message in a box. */ class FontComponent extends JComponent { private static final int DEFAULT_WIDTH = 300; private static final int DEFAULT_HEIGHT = 200; public void paintComponent(Graphics g) { Graphics2D g2 = (Graphics2D) g; String message = "Hello, World!"; Font f = new Font("Serif", Font.BOLD, 36); g2.setFont(f); // 测量消息的大小 FontRenderContext context = g2.getFontRenderContext(); Rectangle2D bounds = f.getStringBounds(message, context); // 设置(x,y)=文本左上角 double x = (getWidth() - bounds.getWidth()) / 2; double y = (getHeight() - bounds.getHeight()) / 2; // 增加到Y到达基线 double ascent = -bounds.getY(); double baseY = y + ascent; // 汲取信息 g2.drawString(message, (int) x, (int) baseY); g2.setPaint(Color.LIGHT_GRAY); // 绘制基线 g2.draw(new Line2D.Double(x, baseY, x + bounds.getWidth(), baseY)); // 绘制封闭矩形 Double rect = new Rectangle2D.Double(x, y, bounds.getWidth(), bounds.getHeight()); g2.draw(rect); } public Dimension getPreferredSize() { return new Dimension(DEFAULT_WIDTH, DEFAULT_HEIGHT); } }
测试程序6:
l 在elipse IDE中调试运行教材436页-437程序10-6,结合程序运行结果理解程序;
l 了解2D图形图像的显示方法。
package image; import java.awt.*; import javax.swing.*; /** * @version 1.34 2015-05-12 * @author Cay Horstmann */ public class ImageTest { public static void main(String[] args) { EventQueue.invokeLater(() -> { JFrame frame = new ImageFrame(); frame.setTitle("ImageTest"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); }); } } /** * A frame with an image component */ class ImageFrame extends JFrame { public ImageFrame() { add(new ImageComponent()); pack(); } } /** * A component that displays a tiled image */ class ImageComponent extends JComponent { private static final int DEFAULT_WIDTH = 300; private static final int DEFAULT_HEIGHT = 200; private Image image; public ImageComponent() { image = new ImageIcon("blue-ball.gif").getImage(); } public void paintComponent(Graphics g) { if (image == null) return; int imageWidth = image.getWidth(null); int imageHeight = image.getHeight(null); // 在左上角绘制图像 g.drawImage(image, 0, 0, null); // 在组件上平铺图像 for (int i = 0; i * imageWidth <= getWidth(); i++) for (int j = 0; j * imageHeight <= getHeight(); j++) if (i + j > 0) g.copyArea(0, 0, imageWidth, imageHeight, i * imageWidth, j * imageHeight); } public Dimension getPreferredSize() { return new Dimension(DEFAULT_WIDTH, DEFAULT_HEIGHT); }
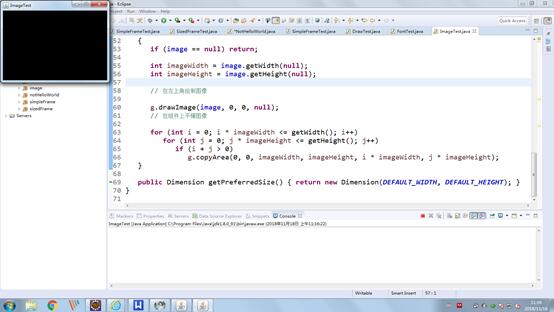
}
package image; import java.awt.*; import javax.swing.*; /** * @version 1.34 2015-05-12 * @author Cay Horstmann */ public class ImageTest { public static void main(String[] args) { EventQueue.invokeLater(() -> { JFrame frame = new ImageFrame(); frame.setTitle("ImageTest"); frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); frame.setVisible(true); }); } } /** * A frame with an image component */ class ImageFrame extends JFrame { public ImageFrame() { add(new ImageComponent()); pack(); } } /** * A component that displays a tiled image */ class ImageComponent extends JComponent { private static final int DEFAULT_WIDTH =500; private static final int DEFAULT_HEIGHT = 300; private Image image; public ImageComponent() { image = new ImageIcon("water.gif").getImage(); } public void paintComponent(Graphics g) { if (image == null) return; int imageWidth = image.getWidth(null); int imageHeight = image.getHeight(null); // 在左上角绘制图像 g.drawImage(image, 0, 0, null); // 在组件上平铺图像 for (int i = 0; i * imageWidth <= getWidth(); i++) for (int j = 0; j * imageHeight <= getHeight(); j++) if (i + j > 0) g.copyArea(0, 0, imageWidth, imageHeight, i * imageWidth, j * imageHeight); } public Dimension getPreferredSize() { return new Dimension(DEFAULT_WIDTH, DEFAULT_HEIGHT); } }
实验2:课后完成PTA平台题目集:2018秋季西北师范大学面向对象程序设计(Java)练习题集(ch6-ch9)
第三部分:实验总结:
本周学习了图形程序设计的内容,基本了解了用户界面的框架设置、属性,以及如何关闭窗口,用JFrame。(EXIT_ON_CLOSE、JFrame.DO_NOTHING_ON_CLOSE的用法);本章知识对我而言就是2D图形的处理,在web前段开发技术课程中基本了解了字体、颜色的设置,最基本的用法需要牢记掌握,因此这一章比较容易理解这部分的内容;最后一个实验,需要修改一下窗口的可关闭操作以及图片的路径设置,在实验课上,有了学长的演示,下来自己做的时候就很简单。但是毕竟这是在引领下完成的,我还需要思考自己能不能做出来。